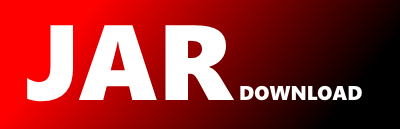
com.pulumi.azurenative.devtestlab.kotlin.outputs.GetVirtualMachineResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.devtestlab.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A virtual machine.
* @property allowClaim Indicates whether another user can take ownership of the virtual machine
* @property applicableSchedule The applicable schedule for the virtual machine.
* @property artifactDeploymentStatus The artifact deployment status for the virtual machine.
* @property artifacts The artifacts to be installed on the virtual machine.
* @property computeId The resource identifier (Microsoft.Compute) of the virtual machine.
* @property computeVm The compute virtual machine properties.
* @property createdByUser The email address of creator of the virtual machine.
* @property createdByUserId The object identifier of the creator of the virtual machine.
* @property createdDate The creation date of the virtual machine.
* @property customImageId The custom image identifier of the virtual machine.
* @property dataDiskParameters New or existing data disks to attach to the virtual machine after creation
* @property disallowPublicIpAddress Indicates whether the virtual machine is to be created without a public IP address.
* @property environmentId The resource ID of the environment that contains this virtual machine, if any.
* @property expirationDate The expiration date for VM.
* @property fqdn The fully-qualified domain name of the virtual machine.
* @property galleryImageReference The Microsoft Azure Marketplace image reference of the virtual machine.
* @property id The identifier of the resource.
* @property isAuthenticationWithSshKey Indicates whether this virtual machine uses an SSH key for authentication.
* @property labSubnetName The lab subnet name of the virtual machine.
* @property labVirtualNetworkId The lab virtual network identifier of the virtual machine.
* @property lastKnownPowerState Last known compute power state captured in DTL
* @property location The location of the resource.
* @property name The name of the resource.
* @property networkInterface The network interface properties.
* @property notes The notes of the virtual machine.
* @property osType The OS type of the virtual machine.
* @property ownerObjectId The object identifier of the owner of the virtual machine.
* @property ownerUserPrincipalName The user principal name of the virtual machine owner.
* @property password The password of the virtual machine administrator.
* @property planId The id of the plan associated with the virtual machine image
* @property provisioningState The provisioning status of the resource.
* @property scheduleParameters Virtual Machine schedules to be created
* @property size The size of the virtual machine.
* @property sshKey The SSH key of the virtual machine administrator.
* @property storageType Storage type to use for virtual machine (i.e. Standard, Premium).
* @property tags The tags of the resource.
* @property type The type of the resource.
* @property uniqueIdentifier The unique immutable identifier of a resource (Guid).
* @property userName The user name of the virtual machine.
* @property virtualMachineCreationSource Tells source of creation of lab virtual machine. Output property only.
*/
public data class GetVirtualMachineResult(
public val allowClaim: Boolean? = null,
public val applicableSchedule: ApplicableScheduleResponse,
public val artifactDeploymentStatus: ArtifactDeploymentStatusPropertiesResponse,
public val artifacts: List? = null,
public val computeId: String,
public val computeVm: ComputeVmPropertiesResponse,
public val createdByUser: String,
public val createdByUserId: String,
public val createdDate: String? = null,
public val customImageId: String? = null,
public val dataDiskParameters: List? = null,
public val disallowPublicIpAddress: Boolean? = null,
public val environmentId: String? = null,
public val expirationDate: String? = null,
public val fqdn: String,
public val galleryImageReference: GalleryImageReferenceResponse? = null,
public val id: String,
public val isAuthenticationWithSshKey: Boolean? = null,
public val labSubnetName: String? = null,
public val labVirtualNetworkId: String? = null,
public val lastKnownPowerState: String,
public val location: String? = null,
public val name: String,
public val networkInterface: NetworkInterfacePropertiesResponse? = null,
public val notes: String? = null,
public val osType: String,
public val ownerObjectId: String? = null,
public val ownerUserPrincipalName: String? = null,
public val password: String? = null,
public val planId: String? = null,
public val provisioningState: String,
public val scheduleParameters: List? = null,
public val size: String? = null,
public val sshKey: String? = null,
public val storageType: String? = null,
public val tags: Map? = null,
public val type: String,
public val uniqueIdentifier: String,
public val userName: String? = null,
public val virtualMachineCreationSource: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.devtestlab.outputs.GetVirtualMachineResult): GetVirtualMachineResult = GetVirtualMachineResult(
allowClaim = javaType.allowClaim().map({ args0 -> args0 }).orElse(null),
applicableSchedule = javaType.applicableSchedule().let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.ApplicableScheduleResponse.Companion.toKotlin(args0)
}),
artifactDeploymentStatus = javaType.artifactDeploymentStatus().let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.ArtifactDeploymentStatusPropertiesResponse.Companion.toKotlin(args0)
}),
artifacts = javaType.artifacts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.ArtifactInstallPropertiesResponse.Companion.toKotlin(args0)
})
}),
computeId = javaType.computeId(),
computeVm = javaType.computeVm().let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.ComputeVmPropertiesResponse.Companion.toKotlin(args0)
}),
createdByUser = javaType.createdByUser(),
createdByUserId = javaType.createdByUserId(),
createdDate = javaType.createdDate().map({ args0 -> args0 }).orElse(null),
customImageId = javaType.customImageId().map({ args0 -> args0 }).orElse(null),
dataDiskParameters = javaType.dataDiskParameters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.DataDiskPropertiesResponse.Companion.toKotlin(args0)
})
}),
disallowPublicIpAddress = javaType.disallowPublicIpAddress().map({ args0 -> args0 }).orElse(null),
environmentId = javaType.environmentId().map({ args0 -> args0 }).orElse(null),
expirationDate = javaType.expirationDate().map({ args0 -> args0 }).orElse(null),
fqdn = javaType.fqdn(),
galleryImageReference = javaType.galleryImageReference().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.GalleryImageReferenceResponse.Companion.toKotlin(args0)
})
}).orElse(null),
id = javaType.id(),
isAuthenticationWithSshKey = javaType.isAuthenticationWithSshKey().map({ args0 ->
args0
}).orElse(null),
labSubnetName = javaType.labSubnetName().map({ args0 -> args0 }).orElse(null),
labVirtualNetworkId = javaType.labVirtualNetworkId().map({ args0 -> args0 }).orElse(null),
lastKnownPowerState = javaType.lastKnownPowerState(),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
networkInterface = javaType.networkInterface().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.NetworkInterfacePropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
notes = javaType.notes().map({ args0 -> args0 }).orElse(null),
osType = javaType.osType(),
ownerObjectId = javaType.ownerObjectId().map({ args0 -> args0 }).orElse(null),
ownerUserPrincipalName = javaType.ownerUserPrincipalName().map({ args0 -> args0 }).orElse(null),
password = javaType.password().map({ args0 -> args0 }).orElse(null),
planId = javaType.planId().map({ args0 -> args0 }).orElse(null),
provisioningState = javaType.provisioningState(),
scheduleParameters = javaType.scheduleParameters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.devtestlab.kotlin.outputs.ScheduleCreationParameterResponse.Companion.toKotlin(args0)
})
}),
size = javaType.size().map({ args0 -> args0 }).orElse(null),
sshKey = javaType.sshKey().map({ args0 -> args0 }).orElse(null),
storageType = javaType.storageType().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
uniqueIdentifier = javaType.uniqueIdentifier(),
userName = javaType.userName().map({ args0 -> args0 }).orElse(null),
virtualMachineCreationSource = javaType.virtualMachineCreationSource(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy