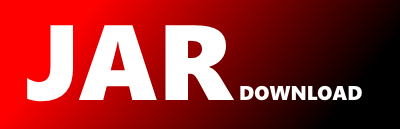
com.pulumi.azurenative.digitaltwins.kotlin.inputs.EventGridArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.digitaltwins.kotlin.inputs
import com.pulumi.azurenative.digitaltwins.inputs.EventGridArgs.builder
import com.pulumi.azurenative.digitaltwins.kotlin.enums.AuthenticationType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Properties related to EventGrid.
* @property accessKey1 EventGrid secondary accesskey. Will be obfuscated during read.
* @property accessKey2 EventGrid secondary accesskey. Will be obfuscated during read.
* @property authenticationType Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
* @property deadLetterSecret Dead letter storage secret for key-based authentication. Will be obfuscated during read.
* @property deadLetterUri Dead letter storage URL for identity-based authentication.
* @property endpointType The type of Digital Twins endpoint
* Expected value is 'EventGrid'.
* @property identity Managed identity properties for the endpoint.
* @property topicEndpoint EventGrid Topic Endpoint.
*/
public data class EventGridArgs(
public val accessKey1: Output,
public val accessKey2: Output? = null,
public val authenticationType: Output>? = null,
public val deadLetterSecret: Output? = null,
public val deadLetterUri: Output? = null,
public val endpointType: Output,
public val identity: Output? = null,
public val topicEndpoint: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.digitaltwins.inputs.EventGridArgs =
com.pulumi.azurenative.digitaltwins.inputs.EventGridArgs.builder()
.accessKey1(accessKey1.applyValue({ args0 -> args0 }))
.accessKey2(accessKey2?.applyValue({ args0 -> args0 }))
.authenticationType(
authenticationType?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.deadLetterSecret(deadLetterSecret?.applyValue({ args0 -> args0 }))
.deadLetterUri(deadLetterUri?.applyValue({ args0 -> args0 }))
.endpointType(endpointType.applyValue({ args0 -> args0 }))
.identity(identity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.topicEndpoint(topicEndpoint.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EventGridArgs].
*/
@PulumiTagMarker
public class EventGridArgsBuilder internal constructor() {
private var accessKey1: Output? = null
private var accessKey2: Output? = null
private var authenticationType: Output>? = null
private var deadLetterSecret: Output? = null
private var deadLetterUri: Output? = null
private var endpointType: Output? = null
private var identity: Output? = null
private var topicEndpoint: Output? = null
/**
* @param value EventGrid secondary accesskey. Will be obfuscated during read.
*/
@JvmName("rewvesvuttddkjsw")
public suspend fun accessKey1(`value`: Output) {
this.accessKey1 = value
}
/**
* @param value EventGrid secondary accesskey. Will be obfuscated during read.
*/
@JvmName("kijfsdeomhhhsotn")
public suspend fun accessKey2(`value`: Output) {
this.accessKey2 = value
}
/**
* @param value Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*/
@JvmName("doihrxwvawfttpjj")
public suspend fun authenticationType(`value`: Output>) {
this.authenticationType = value
}
/**
* @param value Dead letter storage secret for key-based authentication. Will be obfuscated during read.
*/
@JvmName("ndkujfljhnxajisi")
public suspend fun deadLetterSecret(`value`: Output) {
this.deadLetterSecret = value
}
/**
* @param value Dead letter storage URL for identity-based authentication.
*/
@JvmName("nakrfkibbgqfoign")
public suspend fun deadLetterUri(`value`: Output) {
this.deadLetterUri = value
}
/**
* @param value The type of Digital Twins endpoint
* Expected value is 'EventGrid'.
*/
@JvmName("guxdhsngomjjecqi")
public suspend fun endpointType(`value`: Output) {
this.endpointType = value
}
/**
* @param value Managed identity properties for the endpoint.
*/
@JvmName("icutlifqnccpgsrk")
public suspend fun identity(`value`: Output) {
this.identity = value
}
/**
* @param value EventGrid Topic Endpoint.
*/
@JvmName("wiecnxefelrhuvdc")
public suspend fun topicEndpoint(`value`: Output) {
this.topicEndpoint = value
}
/**
* @param value EventGrid secondary accesskey. Will be obfuscated during read.
*/
@JvmName("vqmnnjiovgasojjp")
public suspend fun accessKey1(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.accessKey1 = mapped
}
/**
* @param value EventGrid secondary accesskey. Will be obfuscated during read.
*/
@JvmName("mrxvvchnhualkmgh")
public suspend fun accessKey2(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessKey2 = mapped
}
/**
* @param value Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*/
@JvmName("chqygeupvilxjxqw")
public suspend fun authenticationType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*/
@JvmName("kyitcwxooiywbyyp")
public fun authenticationType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*/
@JvmName("gijucwlcnglpgxef")
public fun authenticationType(`value`: AuthenticationType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value Dead letter storage secret for key-based authentication. Will be obfuscated during read.
*/
@JvmName("liuhixwmwchggpwa")
public suspend fun deadLetterSecret(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadLetterSecret = mapped
}
/**
* @param value Dead letter storage URL for identity-based authentication.
*/
@JvmName("stkdwshyapsopade")
public suspend fun deadLetterUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadLetterUri = mapped
}
/**
* @param value The type of Digital Twins endpoint
* Expected value is 'EventGrid'.
*/
@JvmName("rcqbigtkcjimqrwh")
public suspend fun endpointType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpointType = mapped
}
/**
* @param value Managed identity properties for the endpoint.
*/
@JvmName("sgsfeyxysxjihueq")
public suspend fun identity(`value`: ManagedIdentityReferenceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identity = mapped
}
/**
* @param argument Managed identity properties for the endpoint.
*/
@JvmName("qerlamegfgddtlrv")
public suspend fun identity(argument: suspend ManagedIdentityReferenceArgsBuilder.() -> Unit) {
val toBeMapped = ManagedIdentityReferenceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.identity = mapped
}
/**
* @param value EventGrid Topic Endpoint.
*/
@JvmName("jwetnranhljtompa")
public suspend fun topicEndpoint(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.topicEndpoint = mapped
}
internal fun build(): EventGridArgs = EventGridArgs(
accessKey1 = accessKey1 ?: throw PulumiNullFieldException("accessKey1"),
accessKey2 = accessKey2,
authenticationType = authenticationType,
deadLetterSecret = deadLetterSecret,
deadLetterUri = deadLetterUri,
endpointType = endpointType ?: throw PulumiNullFieldException("endpointType"),
identity = identity,
topicEndpoint = topicEndpoint ?: throw PulumiNullFieldException("topicEndpoint"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy