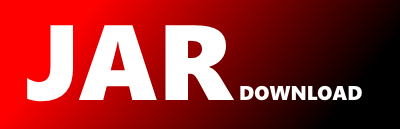
com.pulumi.azurenative.education.kotlin.StudentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.education.kotlin
import com.pulumi.azurenative.education.StudentArgs.builder
import com.pulumi.azurenative.education.kotlin.enums.StudentRole
import com.pulumi.azurenative.education.kotlin.inputs.AmountArgs
import com.pulumi.azurenative.education.kotlin.inputs.AmountArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Student details.
* Azure REST API version: 2021-12-01-preview. Prior API version in Azure Native 1.x: 2021-12-01-preview.
* ## Example Usage
* ### Student
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var student = new AzureNative.Education.Student("student", new()
* {
* BillingAccountName = "{billingAccountName}",
* BillingProfileName = "{billingProfileName}",
* Budget = new AzureNative.Education.Inputs.AmountArgs
* {
* Currency = "USD",
* Value = 100,
* },
* Email = "[email protected]",
* ExpirationDate = "2021-11-09T22:13:21.795Z",
* FirstName = "test",
* InvoiceSectionName = "{invoiceSectionName}",
* LastName = "user",
* Role = AzureNative.Education.StudentRole.Student,
* StudentAlias = "{studentAlias}",
* });
* });
* ```
* ```go
* package main
* import (
* education "github.com/pulumi/pulumi-azure-native-sdk/education/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := education.NewStudent(ctx, "student", &education.StudentArgs{
* BillingAccountName: pulumi.String("{billingAccountName}"),
* BillingProfileName: pulumi.String("{billingProfileName}"),
* Budget: &education.AmountArgs{
* Currency: pulumi.String("USD"),
* Value: pulumi.Float64(100),
* },
* Email: pulumi.String("[email protected]"),
* ExpirationDate: pulumi.String("2021-11-09T22:13:21.795Z"),
* FirstName: pulumi.String("test"),
* InvoiceSectionName: pulumi.String("{invoiceSectionName}"),
* LastName: pulumi.String("user"),
* Role: pulumi.String(education.StudentRoleStudent),
* StudentAlias: pulumi.String("{studentAlias}"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.education.Student;
* import com.pulumi.azurenative.education.StudentArgs;
* import com.pulumi.azurenative.education.inputs.AmountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var student = new Student("student", StudentArgs.builder()
* .billingAccountName("{billingAccountName}")
* .billingProfileName("{billingProfileName}")
* .budget(AmountArgs.builder()
* .currency("USD")
* .value(100)
* .build())
* .email("[email protected]")
* .expirationDate("2021-11-09T22:13:21.795Z")
* .firstName("test")
* .invoiceSectionName("{invoiceSectionName}")
* .lastName("user")
* .role("Student")
* .studentAlias("{studentAlias}")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:education:Student {studentAlias} /providers/Microsoft.Billing/billingAccounts/{billingAccountName}/billingProfiles/{billingProfileName}/invoiceSections/{invoiceSectionName}/providers/Microsoft.Education/labs/default/students/{studentAlias}
* ```
* @property billingAccountName The ID that uniquely identifies a billing account.
* @property billingProfileName The ID that uniquely identifies a billing profile.
* @property budget Student Budget
* @property email Student Email
* @property expirationDate Date this student is set to expire from the lab.
* @property firstName First Name
* @property invoiceSectionName The ID that uniquely identifies an invoice section.
* @property lastName Last Name
* @property role Student Role
* @property studentAlias Student alias.
* @property subscriptionAlias Subscription alias
* @property subscriptionInviteLastSentDate subscription invite last sent date
*/
public data class StudentArgs(
public val billingAccountName: Output? = null,
public val billingProfileName: Output? = null,
public val budget: Output? = null,
public val email: Output? = null,
public val expirationDate: Output? = null,
public val firstName: Output? = null,
public val invoiceSectionName: Output? = null,
public val lastName: Output? = null,
public val role: Output>? = null,
public val studentAlias: Output? = null,
public val subscriptionAlias: Output? = null,
public val subscriptionInviteLastSentDate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.education.StudentArgs =
com.pulumi.azurenative.education.StudentArgs.builder()
.billingAccountName(billingAccountName?.applyValue({ args0 -> args0 }))
.billingProfileName(billingProfileName?.applyValue({ args0 -> args0 }))
.budget(budget?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.email(email?.applyValue({ args0 -> args0 }))
.expirationDate(expirationDate?.applyValue({ args0 -> args0 }))
.firstName(firstName?.applyValue({ args0 -> args0 }))
.invoiceSectionName(invoiceSectionName?.applyValue({ args0 -> args0 }))
.lastName(lastName?.applyValue({ args0 -> args0 }))
.role(
role?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.studentAlias(studentAlias?.applyValue({ args0 -> args0 }))
.subscriptionAlias(subscriptionAlias?.applyValue({ args0 -> args0 }))
.subscriptionInviteLastSentDate(
subscriptionInviteLastSentDate?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [StudentArgs].
*/
@PulumiTagMarker
public class StudentArgsBuilder internal constructor() {
private var billingAccountName: Output? = null
private var billingProfileName: Output? = null
private var budget: Output? = null
private var email: Output? = null
private var expirationDate: Output? = null
private var firstName: Output? = null
private var invoiceSectionName: Output? = null
private var lastName: Output? = null
private var role: Output>? = null
private var studentAlias: Output? = null
private var subscriptionAlias: Output? = null
private var subscriptionInviteLastSentDate: Output? = null
/**
* @param value The ID that uniquely identifies a billing account.
*/
@JvmName("wsuaboleqbjnnoow")
public suspend fun billingAccountName(`value`: Output) {
this.billingAccountName = value
}
/**
* @param value The ID that uniquely identifies a billing profile.
*/
@JvmName("mdbcfydsgasipywb")
public suspend fun billingProfileName(`value`: Output) {
this.billingProfileName = value
}
/**
* @param value Student Budget
*/
@JvmName("ykgsuokyjubgofcp")
public suspend fun budget(`value`: Output) {
this.budget = value
}
/**
* @param value Student Email
*/
@JvmName("ndakqfyvuvmyrunj")
public suspend fun email(`value`: Output) {
this.email = value
}
/**
* @param value Date this student is set to expire from the lab.
*/
@JvmName("eboglqwypumpsotf")
public suspend fun expirationDate(`value`: Output) {
this.expirationDate = value
}
/**
* @param value First Name
*/
@JvmName("xapycilvmlpsutkn")
public suspend fun firstName(`value`: Output) {
this.firstName = value
}
/**
* @param value The ID that uniquely identifies an invoice section.
*/
@JvmName("ewuogccjtnmhnmqs")
public suspend fun invoiceSectionName(`value`: Output) {
this.invoiceSectionName = value
}
/**
* @param value Last Name
*/
@JvmName("kfxyewcbvdqcaknj")
public suspend fun lastName(`value`: Output) {
this.lastName = value
}
/**
* @param value Student Role
*/
@JvmName("otqncgerymnnvjdg")
public suspend fun role(`value`: Output>) {
this.role = value
}
/**
* @param value Student alias.
*/
@JvmName("gitipfonvmsgdgxf")
public suspend fun studentAlias(`value`: Output) {
this.studentAlias = value
}
/**
* @param value Subscription alias
*/
@JvmName("irmdsrejpdjbbtru")
public suspend fun subscriptionAlias(`value`: Output) {
this.subscriptionAlias = value
}
/**
* @param value subscription invite last sent date
*/
@JvmName("ekrjdtubektnxsrn")
public suspend fun subscriptionInviteLastSentDate(`value`: Output) {
this.subscriptionInviteLastSentDate = value
}
/**
* @param value The ID that uniquely identifies a billing account.
*/
@JvmName("emjoetlbbenawnqu")
public suspend fun billingAccountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.billingAccountName = mapped
}
/**
* @param value The ID that uniquely identifies a billing profile.
*/
@JvmName("ubknmlnfkythylsj")
public suspend fun billingProfileName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.billingProfileName = mapped
}
/**
* @param value Student Budget
*/
@JvmName("xjpqdpgvvcjflrmw")
public suspend fun budget(`value`: AmountArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.budget = mapped
}
/**
* @param argument Student Budget
*/
@JvmName("vpxssnmarkycwvfh")
public suspend fun budget(argument: suspend AmountArgsBuilder.() -> Unit) {
val toBeMapped = AmountArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.budget = mapped
}
/**
* @param value Student Email
*/
@JvmName("vqssxphmffwohfjp")
public suspend fun email(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.email = mapped
}
/**
* @param value Date this student is set to expire from the lab.
*/
@JvmName("vkofgcipudoyvcxh")
public suspend fun expirationDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expirationDate = mapped
}
/**
* @param value First Name
*/
@JvmName("nmnaggnjemckcnkr")
public suspend fun firstName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.firstName = mapped
}
/**
* @param value The ID that uniquely identifies an invoice section.
*/
@JvmName("wuwctwrawnsjbxwe")
public suspend fun invoiceSectionName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.invoiceSectionName = mapped
}
/**
* @param value Last Name
*/
@JvmName("eorenjneroctxakl")
public suspend fun lastName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastName = mapped
}
/**
* @param value Student Role
*/
@JvmName("jchblmkrlapcwnhe")
public suspend fun role(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Student Role
*/
@JvmName("yoqixmglcguheolg")
public fun role(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Student Role
*/
@JvmName("msvcrrayjmialnhk")
public fun role(`value`: StudentRole) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Student alias.
*/
@JvmName("aghonqhjqxwbwlxi")
public suspend fun studentAlias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.studentAlias = mapped
}
/**
* @param value Subscription alias
*/
@JvmName("qjqbugfnwfqbxxre")
public suspend fun subscriptionAlias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriptionAlias = mapped
}
/**
* @param value subscription invite last sent date
*/
@JvmName("fdilrtxykesrmdbs")
public suspend fun subscriptionInviteLastSentDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriptionInviteLastSentDate = mapped
}
internal fun build(): StudentArgs = StudentArgs(
billingAccountName = billingAccountName,
billingProfileName = billingProfileName,
budget = budget,
email = email,
expirationDate = expirationDate,
firstName = firstName,
invoiceSectionName = invoiceSectionName,
lastName = lastName,
role = role,
studentAlias = studentAlias,
subscriptionAlias = subscriptionAlias,
subscriptionInviteLastSentDate = subscriptionInviteLastSentDate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy