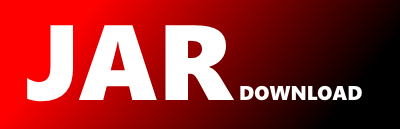
com.pulumi.azurenative.eventgrid.kotlin.EventSubscriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.eventgrid.kotlin
import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs.builder
import com.pulumi.azurenative.eventgrid.kotlin.enums.EventDeliverySchema
import com.pulumi.azurenative.eventgrid.kotlin.inputs.DeadLetterWithResourceIdentityArgs
import com.pulumi.azurenative.eventgrid.kotlin.inputs.DeadLetterWithResourceIdentityArgsBuilder
import com.pulumi.azurenative.eventgrid.kotlin.inputs.DeliveryWithResourceIdentityArgs
import com.pulumi.azurenative.eventgrid.kotlin.inputs.DeliveryWithResourceIdentityArgsBuilder
import com.pulumi.azurenative.eventgrid.kotlin.inputs.EventSubscriptionFilterArgs
import com.pulumi.azurenative.eventgrid.kotlin.inputs.EventSubscriptionFilterArgsBuilder
import com.pulumi.azurenative.eventgrid.kotlin.inputs.RetryPolicyArgs
import com.pulumi.azurenative.eventgrid.kotlin.inputs.RetryPolicyArgsBuilder
import com.pulumi.azurenative.eventgrid.kotlin.inputs.StorageBlobDeadLetterDestinationArgs
import com.pulumi.azurenative.eventgrid.kotlin.inputs.StorageBlobDeadLetterDestinationArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Event Subscription
* Azure REST API version: 2022-06-15. Prior API version in Azure Native 1.x: 2020-06-01.
* Other available API versions: 2017-06-15-preview, 2023-06-01-preview, 2023-12-15-preview, 2024-06-01-preview.
* ## Example Usage
* ### EventSubscriptions_CreateOrUpdateForCustomTopic
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* Destination = new AzureNative.EventGrid.Inputs.EventHubEventSubscriptionDestinationArgs
* {
* EndpointType = "EventHub",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.EventHub/namespaces/ContosoNamespace/eventhubs/EH1",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* Destination: &eventgrid.EventHubEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("EventHub"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.EventHub/namespaces/ContosoNamespace/eventhubs/EH1"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .destination(EventHubEventSubscriptionDestinationArgs.builder()
* .endpointType("EventHub")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.EventHub/namespaces/ContosoNamespace/eventhubs/EH1")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_AzureFunctionDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* DeadLetterDestination = new AzureNative.EventGrid.Inputs.StorageBlobDeadLetterDestinationArgs
* {
* BlobContainerName = "contosocontainer",
* EndpointType = "StorageBlob",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* Destination = new AzureNative.EventGrid.Inputs.AzureFunctionEventSubscriptionDestinationArgs
* {
* EndpointType = "AzureFunction",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Web/sites/ContosoSite/funtions/ContosoFunc",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* DeadLetterDestination: &eventgrid.StorageBlobDeadLetterDestinationArgs{
* BlobContainerName: pulumi.String("contosocontainer"),
* EndpointType: pulumi.String("StorageBlob"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* Destination: &eventgrid.AzureFunctionEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("AzureFunction"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Web/sites/ContosoSite/funtions/ContosoFunc"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.StorageBlobDeadLetterDestinationArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .deadLetterDestination(StorageBlobDeadLetterDestinationArgs.builder()
* .blobContainerName("contosocontainer")
* .endpointType("StorageBlob")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .destination(AzureFunctionEventSubscriptionDestinationArgs.builder()
* .endpointType("AzureFunction")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Web/sites/ContosoSite/funtions/ContosoFunc")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_EventHubDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* DeadLetterDestination = new AzureNative.EventGrid.Inputs.StorageBlobDeadLetterDestinationArgs
* {
* BlobContainerName = "contosocontainer",
* EndpointType = "StorageBlob",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* Destination = new AzureNative.EventGrid.Inputs.EventHubEventSubscriptionDestinationArgs
* {
* EndpointType = "EventHub",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.EventHub/namespaces/ContosoNamespace/eventhubs/EH1",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* DeadLetterDestination: &eventgrid.StorageBlobDeadLetterDestinationArgs{
* BlobContainerName: pulumi.String("contosocontainer"),
* EndpointType: pulumi.String("StorageBlob"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* Destination: &eventgrid.EventHubEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("EventHub"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.EventHub/namespaces/ContosoNamespace/eventhubs/EH1"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.StorageBlobDeadLetterDestinationArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .deadLetterDestination(StorageBlobDeadLetterDestinationArgs.builder()
* .blobContainerName("contosocontainer")
* .endpointType("StorageBlob")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .destination(EventHubEventSubscriptionDestinationArgs.builder()
* .endpointType("EventHub")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.EventHub/namespaces/ContosoNamespace/eventhubs/EH1")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_HybridConnectionDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* DeadLetterDestination = new AzureNative.EventGrid.Inputs.StorageBlobDeadLetterDestinationArgs
* {
* BlobContainerName = "contosocontainer",
* EndpointType = "StorageBlob",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* Destination = new AzureNative.EventGrid.Inputs.HybridConnectionEventSubscriptionDestinationArgs
* {
* EndpointType = "HybridConnection",
* ResourceId = "/subscriptions/d33c5f7a-02ea-40f4-bf52-07f17e84d6a8/resourceGroups/TestRG/providers/Microsoft.Relay/namespaces/ContosoNamespace/hybridConnections/HC1",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* DeadLetterDestination: &eventgrid.StorageBlobDeadLetterDestinationArgs{
* BlobContainerName: pulumi.String("contosocontainer"),
* EndpointType: pulumi.String("StorageBlob"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* Destination: &eventgrid.HybridConnectionEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("HybridConnection"),
* ResourceId: pulumi.String("/subscriptions/d33c5f7a-02ea-40f4-bf52-07f17e84d6a8/resourceGroups/TestRG/providers/Microsoft.Relay/namespaces/ContosoNamespace/hybridConnections/HC1"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.StorageBlobDeadLetterDestinationArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .deadLetterDestination(StorageBlobDeadLetterDestinationArgs.builder()
* .blobContainerName("contosocontainer")
* .endpointType("StorageBlob")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .destination(HybridConnectionEventSubscriptionDestinationArgs.builder()
* .endpointType("HybridConnection")
* .resourceId("/subscriptions/d33c5f7a-02ea-40f4-bf52-07f17e84d6a8/resourceGroups/TestRG/providers/Microsoft.Relay/namespaces/ContosoNamespace/hybridConnections/HC1")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_ServiceBusQueueDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* DeadLetterDestination = new AzureNative.EventGrid.Inputs.StorageBlobDeadLetterDestinationArgs
* {
* BlobContainerName = "contosocontainer",
* EndpointType = "StorageBlob",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* Destination = new AzureNative.EventGrid.Inputs.ServiceBusQueueEventSubscriptionDestinationArgs
* {
* EndpointType = "ServiceBusQueue",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.ServiceBus/namespaces/ContosoNamespace/queues/SBQ",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* DeadLetterDestination: &eventgrid.StorageBlobDeadLetterDestinationArgs{
* BlobContainerName: pulumi.String("contosocontainer"),
* EndpointType: pulumi.String("StorageBlob"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* Destination: &eventgrid.ServiceBusQueueEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("ServiceBusQueue"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.ServiceBus/namespaces/ContosoNamespace/queues/SBQ"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.StorageBlobDeadLetterDestinationArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .deadLetterDestination(StorageBlobDeadLetterDestinationArgs.builder()
* .blobContainerName("contosocontainer")
* .endpointType("StorageBlob")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .destination(ServiceBusQueueEventSubscriptionDestinationArgs.builder()
* .endpointType("ServiceBusQueue")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.ServiceBus/namespaces/ContosoNamespace/queues/SBQ")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_ServiceBusTopicDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* DeadLetterDestination = new AzureNative.EventGrid.Inputs.StorageBlobDeadLetterDestinationArgs
* {
* BlobContainerName = "contosocontainer",
* EndpointType = "StorageBlob",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* Destination = new AzureNative.EventGrid.Inputs.ServiceBusTopicEventSubscriptionDestinationArgs
* {
* EndpointType = "ServiceBusTopic",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.ServiceBus/namespaces/ContosoNamespace/topics/SBT",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* DeadLetterDestination: &eventgrid.StorageBlobDeadLetterDestinationArgs{
* BlobContainerName: pulumi.String("contosocontainer"),
* EndpointType: pulumi.String("StorageBlob"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* Destination: &eventgrid.ServiceBusTopicEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("ServiceBusTopic"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.ServiceBus/namespaces/ContosoNamespace/topics/SBT"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.StorageBlobDeadLetterDestinationArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .deadLetterDestination(StorageBlobDeadLetterDestinationArgs.builder()
* .blobContainerName("contosocontainer")
* .endpointType("StorageBlob")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .destination(ServiceBusTopicEventSubscriptionDestinationArgs.builder()
* .endpointType("ServiceBusTopic")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.ServiceBus/namespaces/ContosoNamespace/topics/SBT")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_StorageQueueDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* DeadLetterDestination = new AzureNative.EventGrid.Inputs.StorageBlobDeadLetterDestinationArgs
* {
* BlobContainerName = "contosocontainer",
* EndpointType = "StorageBlob",
* ResourceId = "/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* Destination = new AzureNative.EventGrid.Inputs.StorageQueueEventSubscriptionDestinationArgs
* {
* EndpointType = "StorageQueue",
* QueueName = "queue1",
* ResourceId = "/subscriptions/d33c5f7a-02ea-40f4-bf52-07f17e84d6a8/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* DeadLetterDestination: &eventgrid.StorageBlobDeadLetterDestinationArgs{
* BlobContainerName: pulumi.String("contosocontainer"),
* EndpointType: pulumi.String("StorageBlob"),
* ResourceId: pulumi.String("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* Destination: &eventgrid.StorageQueueEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("StorageQueue"),
* QueueName: pulumi.String("queue1"),
* ResourceId: pulumi.String("/subscriptions/d33c5f7a-02ea-40f4-bf52-07f17e84d6a8/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.StorageBlobDeadLetterDestinationArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .deadLetterDestination(StorageBlobDeadLetterDestinationArgs.builder()
* .blobContainerName("contosocontainer")
* .endpointType("StorageBlob")
* .resourceId("/subscriptions/55f3dcd4-cac7-43b4-990b-a139d62a1eb2/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .destination(StorageQueueEventSubscriptionDestinationArgs.builder()
* .endpointType("StorageQueue")
* .queueName("queue1")
* .resourceId("/subscriptions/d33c5f7a-02ea-40f4-bf52-07f17e84d6a8/resourceGroups/TestRG/providers/Microsoft.Storage/storageAccounts/contosostg")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForCustomTopic_WebhookDestination
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* Destination = new AzureNative.EventGrid.Inputs.WebHookEventSubscriptionDestinationArgs
* {
* EndpointType = "WebHook",
* EndpointUrl = "https://azurefunctionexample.azurewebsites.net/runtime/webhooks/EventGrid?functionName=EventGridTrigger1&code=PASSWORDCODE",
* },
* EventSubscriptionName = "examplesubscription1",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* Destination: &eventgrid.WebHookEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("WebHook"),
* EndpointUrl: pulumi.String("https://azurefunctionexample.azurewebsites.net/runtime/webhooks/EventGrid?functionName=EventGridTrigger1&code=PASSWORDCODE"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription1"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .destination(WebHookEventSubscriptionDestinationArgs.builder()
* .endpointType("WebHook")
* .endpointUrl("https://azurefunctionexample.azurewebsites.net/runtime/webhooks/EventGrid?functionName=EventGridTrigger1&code=PASSWORDCODE")
* .build())
* .eventSubscriptionName("examplesubscription1")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventGrid/topics/exampletopic1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForResource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* Destination = new AzureNative.EventGrid.Inputs.WebHookEventSubscriptionDestinationArgs
* {
* EndpointType = "WebHook",
* EndpointUrl = "https://requestb.in/15ksip71",
* },
* EventSubscriptionName = "examplesubscription10",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventHub/namespaces/examplenamespace1",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* Destination: &eventgrid.WebHookEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("WebHook"),
* EndpointUrl: pulumi.String("https://requestb.in/15ksip71"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription10"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventHub/namespaces/examplenamespace1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .destination(WebHookEventSubscriptionDestinationArgs.builder()
* .endpointType("WebHook")
* .endpointUrl("https://requestb.in/15ksip71")
* .build())
* .eventSubscriptionName("examplesubscription10")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg/providers/Microsoft.EventHub/namespaces/examplenamespace1")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForResourceGroup
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* Destination = new AzureNative.EventGrid.Inputs.WebHookEventSubscriptionDestinationArgs
* {
* EndpointType = "WebHook",
* EndpointUrl = "https://requestb.in/15ksip71",
* },
* EventSubscriptionName = "examplesubscription2",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* SubjectBeginsWith = "ExamplePrefix",
* SubjectEndsWith = "ExampleSuffix",
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* Destination: &eventgrid.WebHookEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("WebHook"),
* EndpointUrl: pulumi.String("https://requestb.in/15ksip71"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription2"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* SubjectBeginsWith: pulumi.String("ExamplePrefix"),
* SubjectEndsWith: pulumi.String("ExampleSuffix"),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .destination(WebHookEventSubscriptionDestinationArgs.builder()
* .endpointType("WebHook")
* .endpointUrl("https://requestb.in/15ksip71")
* .build())
* .eventSubscriptionName("examplesubscription2")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .subjectBeginsWith("ExamplePrefix")
* .subjectEndsWith("ExampleSuffix")
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4/resourceGroups/examplerg")
* .build());
* }
* }
* ```
* ### EventSubscriptions_CreateOrUpdateForSubscription
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventSubscription = new AzureNative.EventGrid.EventSubscription("eventSubscription", new()
* {
* Destination = new AzureNative.EventGrid.Inputs.WebHookEventSubscriptionDestinationArgs
* {
* EndpointType = "WebHook",
* EndpointUrl = "https://requestb.in/15ksip71",
* },
* EventSubscriptionName = "examplesubscription3",
* Filter = new AzureNative.EventGrid.Inputs.EventSubscriptionFilterArgs
* {
* IsSubjectCaseSensitive = false,
* },
* Scope = "subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4",
* });
* });
* ```
* ```go
* package main
* import (
* eventgrid "github.com/pulumi/pulumi-azure-native-sdk/eventgrid/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventgrid.NewEventSubscription(ctx, "eventSubscription", &eventgrid.EventSubscriptionArgs{
* Destination: &eventgrid.WebHookEventSubscriptionDestinationArgs{
* EndpointType: pulumi.String("WebHook"),
* EndpointUrl: pulumi.String("https://requestb.in/15ksip71"),
* },
* EventSubscriptionName: pulumi.String("examplesubscription3"),
* Filter: &eventgrid.EventSubscriptionFilterArgs{
* IsSubjectCaseSensitive: pulumi.Bool(false),
* },
* Scope: pulumi.String("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.EventSubscription;
* import com.pulumi.azurenative.eventgrid.EventSubscriptionArgs;
* import com.pulumi.azurenative.eventgrid.inputs.EventSubscriptionFilterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventSubscription = new EventSubscription("eventSubscription", EventSubscriptionArgs.builder()
* .destination(WebHookEventSubscriptionDestinationArgs.builder()
* .endpointType("WebHook")
* .endpointUrl("https://requestb.in/15ksip71")
* .build())
* .eventSubscriptionName("examplesubscription3")
* .filter(EventSubscriptionFilterArgs.builder()
* .isSubjectCaseSensitive(false)
* .build())
* .scope("subscriptions/5b4b650e-28b9-4790-b3ab-ddbd88d727c4")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:eventgrid:EventSubscription examplesubscription3 /{scope}/providers/Microsoft.EventGrid/eventSubscriptions/{eventSubscriptionName}
* ```
* @property deadLetterDestination The dead letter destination of the event subscription. Any event that cannot be delivered to its' destination is sent to the dead letter destination.
* Uses Azure Event Grid's identity to acquire the authentication tokens being used during delivery / dead-lettering.
* @property deadLetterWithResourceIdentity The dead letter destination of the event subscription. Any event that cannot be delivered to its' destination is sent to the dead letter destination.
* Uses the managed identity setup on the parent resource (namely, topic or domain) to acquire the authentication tokens being used during delivery / dead-lettering.
* @property deliveryWithResourceIdentity Information about the destination where events have to be delivered for the event subscription.
* Uses the managed identity setup on the parent resource (namely, topic or domain) to acquire the authentication tokens being used during delivery / dead-lettering.
* @property destination Information about the destination where events have to be delivered for the event subscription.
* Uses Azure Event Grid's identity to acquire the authentication tokens being used during delivery / dead-lettering.
* @property eventDeliverySchema The event delivery schema for the event subscription.
* @property eventSubscriptionName Name of the event subscription. Event subscription names must be between 3 and 64 characters in length and should use alphanumeric letters only.
* @property expirationTimeUtc Expiration time of the event subscription.
* @property filter Information about the filter for the event subscription.
* @property labels List of user defined labels.
* @property retryPolicy The retry policy for events. This can be used to configure maximum number of delivery attempts and time to live for events.
* @property scope The identifier of the resource to which the event subscription needs to be created or updated. The scope can be a subscription, or a resource group, or a top level resource belonging to a resource provider namespace, or an EventGrid topic. For example, use '/subscriptions/{subscriptionId}/' for a subscription, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for a resource group, and '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}' for a resource, and '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.EventGrid/topics/{topicName}' for an EventGrid topic.
*/
public data class EventSubscriptionArgs(
public val deadLetterDestination: Output? = null,
public val deadLetterWithResourceIdentity: Output? = null,
public val deliveryWithResourceIdentity: Output? = null,
public val destination: Output? = null,
public val eventDeliverySchema: Output>? = null,
public val eventSubscriptionName: Output? = null,
public val expirationTimeUtc: Output? = null,
public val filter: Output? = null,
public val labels: Output>? = null,
public val retryPolicy: Output? = null,
public val scope: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.eventgrid.EventSubscriptionArgs =
com.pulumi.azurenative.eventgrid.EventSubscriptionArgs.builder()
.deadLetterDestination(
deadLetterDestination?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.deadLetterWithResourceIdentity(
deadLetterWithResourceIdentity?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.deliveryWithResourceIdentity(
deliveryWithResourceIdentity?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.destination(destination?.applyValue({ args0 -> args0 }))
.eventDeliverySchema(
eventDeliverySchema?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.eventSubscriptionName(eventSubscriptionName?.applyValue({ args0 -> args0 }))
.expirationTimeUtc(expirationTimeUtc?.applyValue({ args0 -> args0 }))
.filter(filter?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.labels(labels?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.retryPolicy(retryPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scope(scope?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EventSubscriptionArgs].
*/
@PulumiTagMarker
public class EventSubscriptionArgsBuilder internal constructor() {
private var deadLetterDestination: Output? = null
private var deadLetterWithResourceIdentity: Output? = null
private var deliveryWithResourceIdentity: Output? = null
private var destination: Output? = null
private var eventDeliverySchema: Output>? = null
private var eventSubscriptionName: Output? = null
private var expirationTimeUtc: Output? = null
private var filter: Output? = null
private var labels: Output>? = null
private var retryPolicy: Output? = null
private var scope: Output? = null
/**
* @param value The dead letter destination of the event subscription. Any event that cannot be delivered to its' destination is sent to the dead letter destination.
* Uses Azure Event Grid's identity to acquire the authentication tokens being used during delivery / dead-lettering.
*/
@JvmName("efhlyvuraiewavxa")
public suspend fun deadLetterDestination(`value`: Output) {
this.deadLetterDestination = value
}
/**
* @param value The dead letter destination of the event subscription. Any event that cannot be delivered to its' destination is sent to the dead letter destination.
* Uses the managed identity setup on the parent resource (namely, topic or domain) to acquire the authentication tokens being used during delivery / dead-lettering.
*/
@JvmName("tngcwvvpnetwfspn")
public suspend fun deadLetterWithResourceIdentity(`value`: Output) {
this.deadLetterWithResourceIdentity = value
}
/**
* @param value Information about the destination where events have to be delivered for the event subscription.
* Uses the managed identity setup on the parent resource (namely, topic or domain) to acquire the authentication tokens being used during delivery / dead-lettering.
*/
@JvmName("hipjmrxjgihapjyp")
public suspend fun deliveryWithResourceIdentity(`value`: Output) {
this.deliveryWithResourceIdentity = value
}
/**
* @param value Information about the destination where events have to be delivered for the event subscription.
* Uses Azure Event Grid's identity to acquire the authentication tokens being used during delivery / dead-lettering.
*/
@JvmName("nmjwhwoimvucwqmm")
public suspend fun destination(`value`: Output) {
this.destination = value
}
/**
* @param value The event delivery schema for the event subscription.
*/
@JvmName("blacrybmljsxrqkt")
public suspend fun eventDeliverySchema(`value`: Output>) {
this.eventDeliverySchema = value
}
/**
* @param value Name of the event subscription. Event subscription names must be between 3 and 64 characters in length and should use alphanumeric letters only.
*/
@JvmName("fiikcgtbevdyilmo")
public suspend fun eventSubscriptionName(`value`: Output) {
this.eventSubscriptionName = value
}
/**
* @param value Expiration time of the event subscription.
*/
@JvmName("gsowdlijfdckocbw")
public suspend fun expirationTimeUtc(`value`: Output) {
this.expirationTimeUtc = value
}
/**
* @param value Information about the filter for the event subscription.
*/
@JvmName("eyyqqpvtncunbhei")
public suspend fun filter(`value`: Output) {
this.filter = value
}
/**
* @param value List of user defined labels.
*/
@JvmName("nsuicmpsfewcxbmg")
public suspend fun labels(`value`: Output>) {
this.labels = value
}
@JvmName("xclkrjfthlpippii")
public suspend fun labels(vararg values: Output) {
this.labels = Output.all(values.asList())
}
/**
* @param values List of user defined labels.
*/
@JvmName("rcqosrjbwamjnhok")
public suspend fun labels(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy