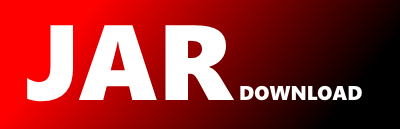
com.pulumi.azurenative.eventgrid.kotlin.inputs.TopicSpacesConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.eventgrid.kotlin.inputs
import com.pulumi.azurenative.eventgrid.inputs.TopicSpacesConfigurationArgs.builder
import com.pulumi.azurenative.eventgrid.kotlin.enums.TopicSpacesConfigurationState
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Properties of the Topic Spaces Configuration.
* @property clientAuthentication Client authentication settings for topic spaces configuration.
* @property maximumClientSessionsPerAuthenticationName The maximum number of sessions per authentication name. The property default value is 1.
* Min allowed value is 1 and max allowed value is 100.
* @property maximumSessionExpiryInHours The maximum session expiry in hours. The property default value is 1 hour.
* Min allowed value is 1 hour and max allowed value is 8 hours.
* @property routeTopicResourceId Fully qualified Azure Resource Id for the Event Grid Topic to which events will be routed to from TopicSpaces under a namespace.
* This property should be in the following format '/subscriptions/{subId}/resourcegroups/{resourceGroupName}/providers/microsoft.EventGrid/topics/{topicName}'.
* This topic should reside in the same region where namespace is located.
* @property routingEnrichments Routing enrichments for topic spaces configuration
* @property routingIdentityInfo Routing identity info for topic spaces configuration.
* @property state Indicate if Topic Spaces Configuration is enabled for the namespace. Default is Disabled.
*/
public data class TopicSpacesConfigurationArgs(
public val clientAuthentication: Output? = null,
public val maximumClientSessionsPerAuthenticationName: Output? = null,
public val maximumSessionExpiryInHours: Output? = null,
public val routeTopicResourceId: Output? = null,
public val routingEnrichments: Output? = null,
public val routingIdentityInfo: Output? = null,
public val state: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.eventgrid.inputs.TopicSpacesConfigurationArgs =
com.pulumi.azurenative.eventgrid.inputs.TopicSpacesConfigurationArgs.builder()
.clientAuthentication(
clientAuthentication?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.maximumClientSessionsPerAuthenticationName(
maximumClientSessionsPerAuthenticationName?.applyValue({ args0 ->
args0
}),
)
.maximumSessionExpiryInHours(maximumSessionExpiryInHours?.applyValue({ args0 -> args0 }))
.routeTopicResourceId(routeTopicResourceId?.applyValue({ args0 -> args0 }))
.routingEnrichments(
routingEnrichments?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.routingIdentityInfo(
routingIdentityInfo?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.state(
state?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [TopicSpacesConfigurationArgs].
*/
@PulumiTagMarker
public class TopicSpacesConfigurationArgsBuilder internal constructor() {
private var clientAuthentication: Output? = null
private var maximumClientSessionsPerAuthenticationName: Output? = null
private var maximumSessionExpiryInHours: Output? = null
private var routeTopicResourceId: Output? = null
private var routingEnrichments: Output? = null
private var routingIdentityInfo: Output? = null
private var state: Output>? = null
/**
* @param value Client authentication settings for topic spaces configuration.
*/
@JvmName("dogfiuwfrdshdldb")
public suspend fun clientAuthentication(`value`: Output) {
this.clientAuthentication = value
}
/**
* @param value The maximum number of sessions per authentication name. The property default value is 1.
* Min allowed value is 1 and max allowed value is 100.
*/
@JvmName("pqwqywfgcggabrog")
public suspend fun maximumClientSessionsPerAuthenticationName(`value`: Output) {
this.maximumClientSessionsPerAuthenticationName = value
}
/**
* @param value The maximum session expiry in hours. The property default value is 1 hour.
* Min allowed value is 1 hour and max allowed value is 8 hours.
*/
@JvmName("heomhqbavtybgbye")
public suspend fun maximumSessionExpiryInHours(`value`: Output) {
this.maximumSessionExpiryInHours = value
}
/**
* @param value Fully qualified Azure Resource Id for the Event Grid Topic to which events will be routed to from TopicSpaces under a namespace.
* This property should be in the following format '/subscriptions/{subId}/resourcegroups/{resourceGroupName}/providers/microsoft.EventGrid/topics/{topicName}'.
* This topic should reside in the same region where namespace is located.
*/
@JvmName("plrlhncpejpvfurt")
public suspend fun routeTopicResourceId(`value`: Output) {
this.routeTopicResourceId = value
}
/**
* @param value Routing enrichments for topic spaces configuration
*/
@JvmName("mbbkifjcgrurnndt")
public suspend fun routingEnrichments(`value`: Output) {
this.routingEnrichments = value
}
/**
* @param value Routing identity info for topic spaces configuration.
*/
@JvmName("crvoanwgspjuavmi")
public suspend fun routingIdentityInfo(`value`: Output) {
this.routingIdentityInfo = value
}
/**
* @param value Indicate if Topic Spaces Configuration is enabled for the namespace. Default is Disabled.
*/
@JvmName("alrgfsscifvybirg")
public suspend fun state(`value`: Output>) {
this.state = value
}
/**
* @param value Client authentication settings for topic spaces configuration.
*/
@JvmName("eampnaqhuhtcutql")
public suspend fun clientAuthentication(`value`: ClientAuthenticationSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientAuthentication = mapped
}
/**
* @param argument Client authentication settings for topic spaces configuration.
*/
@JvmName("qgmvhunryiflvhcy")
public suspend fun clientAuthentication(argument: suspend ClientAuthenticationSettingsArgsBuilder.() -> Unit) {
val toBeMapped = ClientAuthenticationSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.clientAuthentication = mapped
}
/**
* @param value The maximum number of sessions per authentication name. The property default value is 1.
* Min allowed value is 1 and max allowed value is 100.
*/
@JvmName("qfvwywqbstnshyoy")
public suspend fun maximumClientSessionsPerAuthenticationName(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumClientSessionsPerAuthenticationName = mapped
}
/**
* @param value The maximum session expiry in hours. The property default value is 1 hour.
* Min allowed value is 1 hour and max allowed value is 8 hours.
*/
@JvmName("jkxdtcewofdkgkuj")
public suspend fun maximumSessionExpiryInHours(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumSessionExpiryInHours = mapped
}
/**
* @param value Fully qualified Azure Resource Id for the Event Grid Topic to which events will be routed to from TopicSpaces under a namespace.
* This property should be in the following format '/subscriptions/{subId}/resourcegroups/{resourceGroupName}/providers/microsoft.EventGrid/topics/{topicName}'.
* This topic should reside in the same region where namespace is located.
*/
@JvmName("jjpededaflqeqisx")
public suspend fun routeTopicResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.routeTopicResourceId = mapped
}
/**
* @param value Routing enrichments for topic spaces configuration
*/
@JvmName("jrwiltknfbwypqey")
public suspend fun routingEnrichments(`value`: RoutingEnrichmentsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.routingEnrichments = mapped
}
/**
* @param argument Routing enrichments for topic spaces configuration
*/
@JvmName("owpwkfdyvfxivuty")
public suspend fun routingEnrichments(argument: suspend RoutingEnrichmentsArgsBuilder.() -> Unit) {
val toBeMapped = RoutingEnrichmentsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.routingEnrichments = mapped
}
/**
* @param value Routing identity info for topic spaces configuration.
*/
@JvmName("yffejhhrudxqphsm")
public suspend fun routingIdentityInfo(`value`: RoutingIdentityInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.routingIdentityInfo = mapped
}
/**
* @param argument Routing identity info for topic spaces configuration.
*/
@JvmName("xtqqvbannpvqrstx")
public suspend fun routingIdentityInfo(argument: suspend RoutingIdentityInfoArgsBuilder.() -> Unit) {
val toBeMapped = RoutingIdentityInfoArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.routingIdentityInfo = mapped
}
/**
* @param value Indicate if Topic Spaces Configuration is enabled for the namespace. Default is Disabled.
*/
@JvmName("xmfaifaxtnnnpcov")
public suspend fun state(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value Indicate if Topic Spaces Configuration is enabled for the namespace. Default is Disabled.
*/
@JvmName("omfyctggvqauruhc")
public fun state(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value Indicate if Topic Spaces Configuration is enabled for the namespace. Default is Disabled.
*/
@JvmName("olfxqvrjnbkxswmf")
public fun state(`value`: TopicSpacesConfigurationState) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): TopicSpacesConfigurationArgs = TopicSpacesConfigurationArgs(
clientAuthentication = clientAuthentication,
maximumClientSessionsPerAuthenticationName = maximumClientSessionsPerAuthenticationName,
maximumSessionExpiryInHours = maximumSessionExpiryInHours,
routeTopicResourceId = routeTopicResourceId,
routingEnrichments = routingEnrichments,
routingIdentityInfo = routingIdentityInfo,
state = state,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy