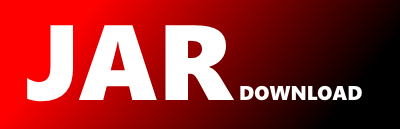
com.pulumi.azurenative.eventhub.kotlin.ApplicationGroupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.eventhub.kotlin
import com.pulumi.azurenative.eventhub.ApplicationGroupArgs.builder
import com.pulumi.azurenative.eventhub.kotlin.inputs.ThrottlingPolicyArgs
import com.pulumi.azurenative.eventhub.kotlin.inputs.ThrottlingPolicyArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The Application Group object
* Azure REST API version: 2022-10-01-preview. Prior API version in Azure Native 1.x: 2022-01-01-preview.
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
* ## Example Usage
* ### ApplicationGroupCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var applicationGroup = new AzureNative.EventHub.ApplicationGroup("applicationGroup", new()
* {
* ApplicationGroupName = "appGroup1",
* ClientAppGroupIdentifier = "SASKeyName=KeyName",
* IsEnabled = true,
* NamespaceName = "contoso-ua-test-eh-system-1",
* Policies = new[]
* {
* new AzureNative.EventHub.Inputs.ThrottlingPolicyArgs
* {
* MetricId = AzureNative.EventHub.MetricId.IncomingMessages,
* Name = "ThrottlingPolicy1",
* RateLimitThreshold = 7912,
* Type = "ThrottlingPolicy",
* },
* new AzureNative.EventHub.Inputs.ThrottlingPolicyArgs
* {
* MetricId = AzureNative.EventHub.MetricId.IncomingBytes,
* Name = "ThrottlingPolicy2",
* RateLimitThreshold = 3951729,
* Type = "ThrottlingPolicy",
* },
* new AzureNative.EventHub.Inputs.ThrottlingPolicyArgs
* {
* MetricId = AzureNative.EventHub.MetricId.OutgoingBytes,
* Name = "ThrottlingPolicy3",
* RateLimitThreshold = 245175,
* Type = "ThrottlingPolicy",
* },
* },
* ResourceGroupName = "contosotest",
* });
* });
* ```
* ```go
* package main
* import (
* eventhub "github.com/pulumi/pulumi-azure-native-sdk/eventhub/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventhub.NewApplicationGroup(ctx, "applicationGroup", &eventhub.ApplicationGroupArgs{
* ApplicationGroupName: pulumi.String("appGroup1"),
* ClientAppGroupIdentifier: pulumi.String("SASKeyName=KeyName"),
* IsEnabled: pulumi.Bool(true),
* NamespaceName: pulumi.String("contoso-ua-test-eh-system-1"),
* Policies: eventhub.ThrottlingPolicyArray{
* &eventhub.ThrottlingPolicyArgs{
* MetricId: pulumi.String(eventhub.MetricIdIncomingMessages),
* Name: pulumi.String("ThrottlingPolicy1"),
* RateLimitThreshold: pulumi.Float64(7912),
* Type: pulumi.String("ThrottlingPolicy"),
* },
* &eventhub.ThrottlingPolicyArgs{
* MetricId: pulumi.String(eventhub.MetricIdIncomingBytes),
* Name: pulumi.String("ThrottlingPolicy2"),
* RateLimitThreshold: pulumi.Float64(3951729),
* Type: pulumi.String("ThrottlingPolicy"),
* },
* &eventhub.ThrottlingPolicyArgs{
* MetricId: pulumi.String(eventhub.MetricIdOutgoingBytes),
* Name: pulumi.String("ThrottlingPolicy3"),
* RateLimitThreshold: pulumi.Float64(245175),
* Type: pulumi.String("ThrottlingPolicy"),
* },
* },
* ResourceGroupName: pulumi.String("contosotest"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventhub.ApplicationGroup;
* import com.pulumi.azurenative.eventhub.ApplicationGroupArgs;
* import com.pulumi.azurenative.eventhub.inputs.ThrottlingPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var applicationGroup = new ApplicationGroup("applicationGroup", ApplicationGroupArgs.builder()
* .applicationGroupName("appGroup1")
* .clientAppGroupIdentifier("SASKeyName=KeyName")
* .isEnabled(true)
* .namespaceName("contoso-ua-test-eh-system-1")
* .policies(
* ThrottlingPolicyArgs.builder()
* .metricId("IncomingMessages")
* .name("ThrottlingPolicy1")
* .rateLimitThreshold(7912)
* .type("ThrottlingPolicy")
* .build(),
* ThrottlingPolicyArgs.builder()
* .metricId("IncomingBytes")
* .name("ThrottlingPolicy2")
* .rateLimitThreshold(3951729)
* .type("ThrottlingPolicy")
* .build(),
* ThrottlingPolicyArgs.builder()
* .metricId("OutgoingBytes")
* .name("ThrottlingPolicy3")
* .rateLimitThreshold(245175)
* .type("ThrottlingPolicy")
* .build())
* .resourceGroupName("contosotest")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:eventhub:ApplicationGroup appGroup1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.EventHub/namespaces/{namespaceName}/applicationGroups/{applicationGroupName}
* ```
* @property applicationGroupName The Application Group name
* @property clientAppGroupIdentifier The Unique identifier for application group.Supports SAS(SASKeyName=KeyName) or AAD(AADAppID=Guid)
* @property isEnabled Determines if Application Group is allowed to create connection with namespace or not. Once the isEnabled is set to false, all the existing connections of application group gets dropped and no new connections will be allowed
* @property namespaceName The Namespace name
* @property policies List of group policies that define the behavior of application group. The policies can support resource governance scenarios such as limiting ingress or egress traffic.
* @property resourceGroupName Name of the resource group within the azure subscription.
*/
public data class ApplicationGroupArgs(
public val applicationGroupName: Output? = null,
public val clientAppGroupIdentifier: Output? = null,
public val isEnabled: Output? = null,
public val namespaceName: Output? = null,
public val policies: Output>? = null,
public val resourceGroupName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.eventhub.ApplicationGroupArgs =
com.pulumi.azurenative.eventhub.ApplicationGroupArgs.builder()
.applicationGroupName(applicationGroupName?.applyValue({ args0 -> args0 }))
.clientAppGroupIdentifier(clientAppGroupIdentifier?.applyValue({ args0 -> args0 }))
.isEnabled(isEnabled?.applyValue({ args0 -> args0 }))
.namespaceName(namespaceName?.applyValue({ args0 -> args0 }))
.policies(
policies?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApplicationGroupArgs].
*/
@PulumiTagMarker
public class ApplicationGroupArgsBuilder internal constructor() {
private var applicationGroupName: Output? = null
private var clientAppGroupIdentifier: Output? = null
private var isEnabled: Output? = null
private var namespaceName: Output? = null
private var policies: Output>? = null
private var resourceGroupName: Output? = null
/**
* @param value The Application Group name
*/
@JvmName("baprigsaaasstrxd")
public suspend fun applicationGroupName(`value`: Output) {
this.applicationGroupName = value
}
/**
* @param value The Unique identifier for application group.Supports SAS(SASKeyName=KeyName) or AAD(AADAppID=Guid)
*/
@JvmName("rlifgcahpxgkjfyn")
public suspend fun clientAppGroupIdentifier(`value`: Output) {
this.clientAppGroupIdentifier = value
}
/**
* @param value Determines if Application Group is allowed to create connection with namespace or not. Once the isEnabled is set to false, all the existing connections of application group gets dropped and no new connections will be allowed
*/
@JvmName("ycylpvnvhicjyqlp")
public suspend fun isEnabled(`value`: Output) {
this.isEnabled = value
}
/**
* @param value The Namespace name
*/
@JvmName("wneetxaqjvxunpuu")
public suspend fun namespaceName(`value`: Output) {
this.namespaceName = value
}
/**
* @param value List of group policies that define the behavior of application group. The policies can support resource governance scenarios such as limiting ingress or egress traffic.
*/
@JvmName("gqbmtovsdfepgruy")
public suspend fun policies(`value`: Output>) {
this.policies = value
}
@JvmName("pjvygcbjtntvosgn")
public suspend fun policies(vararg values: Output) {
this.policies = Output.all(values.asList())
}
/**
* @param values List of group policies that define the behavior of application group. The policies can support resource governance scenarios such as limiting ingress or egress traffic.
*/
@JvmName("rndikrklrwbriwgp")
public suspend fun policies(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy