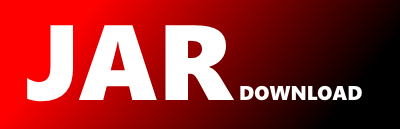
com.pulumi.azurenative.eventhub.kotlin.ConsumerGroupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.eventhub.kotlin
import com.pulumi.azurenative.eventhub.ConsumerGroupArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Single item in List or Get Consumer group operation
* Azure REST API version: 2022-10-01-preview. Prior API version in Azure Native 1.x: 2017-04-01.
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
* ## Example Usage
* ### ConsumerGroupCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var consumerGroup = new AzureNative.EventHub.ConsumerGroup("consumerGroup", new()
* {
* ConsumerGroupName = "sdk-ConsumerGroup-5563",
* EventHubName = "sdk-EventHub-6681",
* NamespaceName = "sdk-Namespace-2661",
* ResourceGroupName = "ArunMonocle",
* UserMetadata = "New consumergroup",
* });
* });
* ```
* ```go
* package main
* import (
* eventhub "github.com/pulumi/pulumi-azure-native-sdk/eventhub/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := eventhub.NewConsumerGroup(ctx, "consumerGroup", &eventhub.ConsumerGroupArgs{
* ConsumerGroupName: pulumi.String("sdk-ConsumerGroup-5563"),
* EventHubName: pulumi.String("sdk-EventHub-6681"),
* NamespaceName: pulumi.String("sdk-Namespace-2661"),
* ResourceGroupName: pulumi.String("ArunMonocle"),
* UserMetadata: pulumi.String("New consumergroup"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventhub.ConsumerGroup;
* import com.pulumi.azurenative.eventhub.ConsumerGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var consumerGroup = new ConsumerGroup("consumerGroup", ConsumerGroupArgs.builder()
* .consumerGroupName("sdk-ConsumerGroup-5563")
* .eventHubName("sdk-EventHub-6681")
* .namespaceName("sdk-Namespace-2661")
* .resourceGroupName("ArunMonocle")
* .userMetadata("New consumergroup")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:eventhub:ConsumerGroup sdk-ConsumerGroup-5563 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.EventHub/namespaces/{namespaceName}/eventhubs/{eventHubName}/consumergroups/{consumerGroupName}
* ```
* @property consumerGroupName The consumer group name
* @property eventHubName The Event Hub name
* @property namespaceName The Namespace name
* @property resourceGroupName Name of the resource group within the azure subscription.
* @property userMetadata User Metadata is a placeholder to store user-defined string data with maximum length 1024. e.g. it can be used to store descriptive data, such as list of teams and their contact information also user-defined configuration settings can be stored.
*/
public data class ConsumerGroupArgs(
public val consumerGroupName: Output? = null,
public val eventHubName: Output? = null,
public val namespaceName: Output? = null,
public val resourceGroupName: Output? = null,
public val userMetadata: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.eventhub.ConsumerGroupArgs =
com.pulumi.azurenative.eventhub.ConsumerGroupArgs.builder()
.consumerGroupName(consumerGroupName?.applyValue({ args0 -> args0 }))
.eventHubName(eventHubName?.applyValue({ args0 -> args0 }))
.namespaceName(namespaceName?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.userMetadata(userMetadata?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConsumerGroupArgs].
*/
@PulumiTagMarker
public class ConsumerGroupArgsBuilder internal constructor() {
private var consumerGroupName: Output? = null
private var eventHubName: Output? = null
private var namespaceName: Output? = null
private var resourceGroupName: Output? = null
private var userMetadata: Output? = null
/**
* @param value The consumer group name
*/
@JvmName("kpvwosnsixttyqto")
public suspend fun consumerGroupName(`value`: Output) {
this.consumerGroupName = value
}
/**
* @param value The Event Hub name
*/
@JvmName("ndreamwrenacoiku")
public suspend fun eventHubName(`value`: Output) {
this.eventHubName = value
}
/**
* @param value The Namespace name
*/
@JvmName("jyxqkidrprqtrxey")
public suspend fun namespaceName(`value`: Output) {
this.namespaceName = value
}
/**
* @param value Name of the resource group within the azure subscription.
*/
@JvmName("awrcyahywcxtiwpa")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value User Metadata is a placeholder to store user-defined string data with maximum length 1024. e.g. it can be used to store descriptive data, such as list of teams and their contact information also user-defined configuration settings can be stored.
*/
@JvmName("edlphjckcuvkxjbd")
public suspend fun userMetadata(`value`: Output) {
this.userMetadata = value
}
/**
* @param value The consumer group name
*/
@JvmName("hqkrcwvklcnvtejs")
public suspend fun consumerGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consumerGroupName = mapped
}
/**
* @param value The Event Hub name
*/
@JvmName("oxtrrervwuwkjfjy")
public suspend fun eventHubName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventHubName = mapped
}
/**
* @param value The Namespace name
*/
@JvmName("sdlxnbueqvvndyvc")
public suspend fun namespaceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespaceName = mapped
}
/**
* @param value Name of the resource group within the azure subscription.
*/
@JvmName("dngcpvevxsfbpuel")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value User Metadata is a placeholder to store user-defined string data with maximum length 1024. e.g. it can be used to store descriptive data, such as list of teams and their contact information also user-defined configuration settings can be stored.
*/
@JvmName("cseibwbpyxxmwgrs")
public suspend fun userMetadata(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userMetadata = mapped
}
internal fun build(): ConsumerGroupArgs = ConsumerGroupArgs(
consumerGroupName = consumerGroupName,
eventHubName = eventHubName,
namespaceName = namespaceName,
resourceGroupName = resourceGroupName,
userMetadata = userMetadata,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy