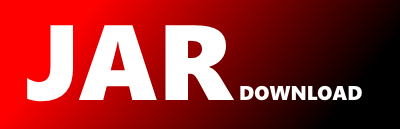
com.pulumi.azurenative.hdinsight.kotlin.outputs.ClusterGetPropertiesResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.hdinsight.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* The properties of cluster.
* @property clusterDefinition The cluster definition.
* @property clusterHdpVersion The hdp version of the cluster.
* @property clusterId The cluster id.
* @property clusterState The state of the cluster.
* @property clusterVersion The version of the cluster.
* @property computeIsolationProperties The compute isolation properties.
* @property computeProfile The compute profile.
* @property connectivityEndpoints The list of connectivity endpoints.
* @property createdDate The date on which the cluster was created.
* @property diskEncryptionProperties The disk encryption properties.
* @property encryptionInTransitProperties The encryption-in-transit properties.
* @property errors The list of errors.
* @property excludedServicesConfig The excluded services config.
* @property kafkaRestProperties The cluster kafka rest proxy configuration.
* @property minSupportedTlsVersion The minimal supported tls version.
* @property networkProperties The network properties.
* @property osType The type of operating system.
* @property privateEndpointConnections The list of private endpoint connections.
* @property privateLinkConfigurations The private link configurations.
* @property provisioningState The provisioning state, which only appears in the response.
* @property quotaInfo The quota information.
* @property securityProfile The security profile.
* @property storageProfile The storage profile.
* @property tier The cluster tier.
*/
public data class ClusterGetPropertiesResponse(
public val clusterDefinition: ClusterDefinitionResponse,
public val clusterHdpVersion: String? = null,
public val clusterId: String? = null,
public val clusterState: String? = null,
public val clusterVersion: String? = null,
public val computeIsolationProperties: ComputeIsolationPropertiesResponse? = null,
public val computeProfile: ComputeProfileResponse? = null,
public val connectivityEndpoints: List? = null,
public val createdDate: String? = null,
public val diskEncryptionProperties: DiskEncryptionPropertiesResponse? = null,
public val encryptionInTransitProperties: EncryptionInTransitPropertiesResponse? = null,
public val errors: List? = null,
public val excludedServicesConfig: ExcludedServicesConfigResponse? = null,
public val kafkaRestProperties: KafkaRestPropertiesResponse? = null,
public val minSupportedTlsVersion: String? = null,
public val networkProperties: NetworkPropertiesResponse? = null,
public val osType: String? = null,
public val privateEndpointConnections: List,
public val privateLinkConfigurations: List? = null,
public val provisioningState: String? = null,
public val quotaInfo: QuotaInfoResponse? = null,
public val securityProfile: SecurityProfileResponse? = null,
public val storageProfile: StorageProfileResponse? = null,
public val tier: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.hdinsight.outputs.ClusterGetPropertiesResponse): ClusterGetPropertiesResponse = ClusterGetPropertiesResponse(
clusterDefinition = javaType.clusterDefinition().let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.ClusterDefinitionResponse.Companion.toKotlin(args0)
}),
clusterHdpVersion = javaType.clusterHdpVersion().map({ args0 -> args0 }).orElse(null),
clusterId = javaType.clusterId().map({ args0 -> args0 }).orElse(null),
clusterState = javaType.clusterState().map({ args0 -> args0 }).orElse(null),
clusterVersion = javaType.clusterVersion().map({ args0 -> args0 }).orElse(null),
computeIsolationProperties = javaType.computeIsolationProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.ComputeIsolationPropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
computeProfile = javaType.computeProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.ComputeProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
connectivityEndpoints = javaType.connectivityEndpoints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.ConnectivityEndpointResponse.Companion.toKotlin(args0)
})
}),
createdDate = javaType.createdDate().map({ args0 -> args0 }).orElse(null),
diskEncryptionProperties = javaType.diskEncryptionProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.DiskEncryptionPropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
encryptionInTransitProperties = javaType.encryptionInTransitProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.EncryptionInTransitPropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
errors = javaType.errors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.ErrorsResponse.Companion.toKotlin(args0)
})
}),
excludedServicesConfig = javaType.excludedServicesConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.ExcludedServicesConfigResponse.Companion.toKotlin(args0)
})
}).orElse(null),
kafkaRestProperties = javaType.kafkaRestProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.KafkaRestPropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
minSupportedTlsVersion = javaType.minSupportedTlsVersion().map({ args0 -> args0 }).orElse(null),
networkProperties = javaType.networkProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.NetworkPropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
osType = javaType.osType().map({ args0 -> args0 }).orElse(null),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.PrivateEndpointConnectionResponse.Companion.toKotlin(args0)
})
}),
privateLinkConfigurations = javaType.privateLinkConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.PrivateLinkConfigurationResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState().map({ args0 -> args0 }).orElse(null),
quotaInfo = javaType.quotaInfo().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.QuotaInfoResponse.Companion.toKotlin(args0)
})
}).orElse(null),
securityProfile = javaType.securityProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.SecurityProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
storageProfile = javaType.storageProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hdinsight.kotlin.outputs.StorageProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
tier = javaType.tier().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy