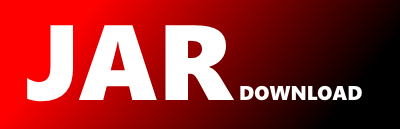
com.pulumi.azurenative.hybridcompute.kotlin.outputs.GetMachineRunCommandResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.hybridcompute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Describes a Run Command
* @property asyncExecution Optional. If set to true, provisioning will complete as soon as script starts and will not wait for script to complete.
* @property errorBlobManagedIdentity User-assigned managed identity that has access to errorBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
* @property errorBlobUri Specifies the Azure storage blob where script error stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer errorBlobManagedIdentity parameter.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property instanceView The machine run command instance view.
* @property location The geo-location where the resource lives
* @property name The name of the resource
* @property outputBlobManagedIdentity User-assigned managed identity that has access to outputBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
* @property outputBlobUri Specifies the Azure storage blob where script output stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer outputBlobManagedIdentity parameter.
* @property parameters The parameters used by the script.
* @property protectedParameters The parameters used by the script.
* @property provisioningState The provisioning state, which only appears in the response.
* @property runAsPassword Specifies the user account password on the machine when executing the run command.
* @property runAsUser Specifies the user account on the machine when executing the run command.
* @property source The source of the run command script.
* @property systemData Azure Resource Manager metadata containing createdBy and modifiedBy information.
* @property tags Resource tags.
* @property timeoutInSeconds The timeout in seconds to execute the run command.
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public data class GetMachineRunCommandResult(
public val asyncExecution: Boolean? = null,
public val errorBlobManagedIdentity: RunCommandManagedIdentityResponse? = null,
public val errorBlobUri: String? = null,
public val id: String,
public val instanceView: MachineRunCommandInstanceViewResponse,
public val location: String,
public val name: String,
public val outputBlobManagedIdentity: RunCommandManagedIdentityResponse? = null,
public val outputBlobUri: String? = null,
public val parameters: List? = null,
public val protectedParameters: List? = null,
public val provisioningState: String,
public val runAsPassword: String? = null,
public val runAsUser: String? = null,
public val source: MachineRunCommandScriptSourceResponse? = null,
public val systemData: SystemDataResponse,
public val tags: Map? = null,
public val timeoutInSeconds: Int? = null,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.hybridcompute.outputs.GetMachineRunCommandResult): GetMachineRunCommandResult = GetMachineRunCommandResult(
asyncExecution = javaType.asyncExecution().map({ args0 -> args0 }).orElse(null),
errorBlobManagedIdentity = javaType.errorBlobManagedIdentity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.RunCommandManagedIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
errorBlobUri = javaType.errorBlobUri().map({ args0 -> args0 }).orElse(null),
id = javaType.id(),
instanceView = javaType.instanceView().let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.MachineRunCommandInstanceViewResponse.Companion.toKotlin(args0)
}),
location = javaType.location(),
name = javaType.name(),
outputBlobManagedIdentity = javaType.outputBlobManagedIdentity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.RunCommandManagedIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
outputBlobUri = javaType.outputBlobUri().map({ args0 -> args0 }).orElse(null),
parameters = javaType.parameters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.RunCommandInputParameterResponse.Companion.toKotlin(args0)
})
}),
protectedParameters = javaType.protectedParameters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.RunCommandInputParameterResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
runAsPassword = javaType.runAsPassword().map({ args0 -> args0 }).orElse(null),
runAsUser = javaType.runAsUser().map({ args0 -> args0 }).orElse(null),
source = javaType.source().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.MachineRunCommandScriptSourceResponse.Companion.toKotlin(args0)
})
}).orElse(null),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.hybridcompute.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
timeoutInSeconds = javaType.timeoutInSeconds().map({ args0 -> args0 }).orElse(null),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy