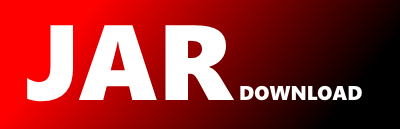
com.pulumi.azurenative.hybriddata.kotlin.JobDefinitionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.hybriddata.kotlin
import com.pulumi.azurenative.hybriddata.JobDefinitionArgs.builder
import com.pulumi.azurenative.hybriddata.kotlin.enums.RunLocation
import com.pulumi.azurenative.hybriddata.kotlin.enums.State
import com.pulumi.azurenative.hybriddata.kotlin.enums.UserConfirmation
import com.pulumi.azurenative.hybriddata.kotlin.inputs.CustomerSecretArgs
import com.pulumi.azurenative.hybriddata.kotlin.inputs.CustomerSecretArgsBuilder
import com.pulumi.azurenative.hybriddata.kotlin.inputs.ScheduleArgs
import com.pulumi.azurenative.hybriddata.kotlin.inputs.ScheduleArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Job Definition.
* Azure REST API version: 2019-06-01. Prior API version in Azure Native 1.x: 2019-06-01.
* ## Example Usage
* ### JobDefinitions_CreateOrUpdatePUT83
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var jobDefinition = new AzureNative.HybridData.JobDefinition("jobDefinition", new()
* {
* DataManagerName = "TestAzureSDKOperations",
* DataServiceInput = new Dictionary
* {
* ["AzureStorageType"] = "Blob",
* ["BackupChoice"] = "UseExistingLatest",
* ["ContainerName"] = "containerfromtest",
* ["DeviceName"] = "8600-SHG0997877L71FC",
* ["FileNameFilter"] = "*",
* ["IsDirectoryMode"] = false,
* ["RootDirectories"] = new[]
* {
* "\\",
* },
* ["VolumeNames"] = new[]
* {
* "TestAutomation",
* },
* },
* DataServiceName = "DataTransformation",
* DataSinkId = "/subscriptions/6e0219f5-327a-4365-904f-05eed4227ad7/resourceGroups/ResourceGroupForSDKTest/providers/Microsoft.HybridData/dataManagers/TestAzureSDKOperations/dataStores/TestAzureStorage1",
* DataSourceId = "/subscriptions/6e0219f5-327a-4365-904f-05eed4227ad7/resourceGroups/ResourceGroupForSDKTest/providers/Microsoft.HybridData/dataManagers/TestAzureSDKOperations/dataStores/TestStorSimpleSource1",
* JobDefinitionName = "jobdeffromtestcode1",
* ResourceGroupName = "ResourceGroupForSDKTest",
* RunLocation = AzureNative.HybridData.RunLocation.Westus,
* State = AzureNative.HybridData.State.Enabled,
* UserConfirmation = AzureNative.HybridData.UserConfirmation.Required,
* });
* });
* ```
* ```go
* package main
* import (
* hybriddata "github.com/pulumi/pulumi-azure-native-sdk/hybriddata/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := hybriddata.NewJobDefinition(ctx, "jobDefinition", &hybriddata.JobDefinitionArgs{
* DataManagerName: pulumi.String("TestAzureSDKOperations"),
* DataServiceInput: pulumi.Any(map[string]interface{}{
* "AzureStorageType": "Blob",
* "BackupChoice": "UseExistingLatest",
* "ContainerName": "containerfromtest",
* "DeviceName": "8600-SHG0997877L71FC",
* "FileNameFilter": "*",
* "IsDirectoryMode": false,
* "RootDirectories": []string{
* "\\",
* },
* "VolumeNames": []string{
* "TestAutomation",
* },
* }),
* DataServiceName: pulumi.String("DataTransformation"),
* DataSinkId: pulumi.String("/subscriptions/6e0219f5-327a-4365-904f-05eed4227ad7/resourceGroups/ResourceGroupForSDKTest/providers/Microsoft.HybridData/dataManagers/TestAzureSDKOperations/dataStores/TestAzureStorage1"),
* DataSourceId: pulumi.String("/subscriptions/6e0219f5-327a-4365-904f-05eed4227ad7/resourceGroups/ResourceGroupForSDKTest/providers/Microsoft.HybridData/dataManagers/TestAzureSDKOperations/dataStores/TestStorSimpleSource1"),
* JobDefinitionName: pulumi.String("jobdeffromtestcode1"),
* ResourceGroupName: pulumi.String("ResourceGroupForSDKTest"),
* RunLocation: hybriddata.RunLocationWestus,
* State: hybriddata.StateEnabled,
* UserConfirmation: hybriddata.UserConfirmationRequired,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.hybriddata.JobDefinition;
* import com.pulumi.azurenative.hybriddata.JobDefinitionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var jobDefinition = new JobDefinition("jobDefinition", JobDefinitionArgs.builder()
* .dataManagerName("TestAzureSDKOperations")
* .dataServiceInput(Map.ofEntries(
* Map.entry("AzureStorageType", "Blob"),
* Map.entry("BackupChoice", "UseExistingLatest"),
* Map.entry("ContainerName", "containerfromtest"),
* Map.entry("DeviceName", "8600-SHG0997877L71FC"),
* Map.entry("FileNameFilter", "*"),
* Map.entry("IsDirectoryMode", false),
* Map.entry("RootDirectories", "\\"),
* Map.entry("VolumeNames", "TestAutomation")
* ))
* .dataServiceName("DataTransformation")
* .dataSinkId("/subscriptions/6e0219f5-327a-4365-904f-05eed4227ad7/resourceGroups/ResourceGroupForSDKTest/providers/Microsoft.HybridData/dataManagers/TestAzureSDKOperations/dataStores/TestAzureStorage1")
* .dataSourceId("/subscriptions/6e0219f5-327a-4365-904f-05eed4227ad7/resourceGroups/ResourceGroupForSDKTest/providers/Microsoft.HybridData/dataManagers/TestAzureSDKOperations/dataStores/TestStorSimpleSource1")
* .jobDefinitionName("jobdeffromtestcode1")
* .resourceGroupName("ResourceGroupForSDKTest")
* .runLocation("westus")
* .state("Enabled")
* .userConfirmation("Required")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:hybriddata:JobDefinition jobdeffromtestcode1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.HybridData/dataManagers/{dataManagerName}/dataServices/{dataServiceName}/jobDefinitions/{jobDefinitionName}
* ```
* @property customerSecrets List of customer secrets containing a key identifier and key value. The key identifier is a way for the specific data source to understand the key. Value contains customer secret encrypted by the encryptionKeys.
* @property dataManagerName The name of the DataManager Resource within the specified resource group. DataManager names must be between 3 and 24 characters in length and use any alphanumeric and underscore only
* @property dataServiceInput A generic json used differently by each data service type.
* @property dataServiceName The data service type of the job definition.
* @property dataSinkId Data Sink Id associated to the job definition.
* @property dataSourceId Data Source Id associated to the job definition.
* @property jobDefinitionName The job definition name to be created or updated.
* @property lastModifiedTime Last modified time of the job definition.
* @property resourceGroupName The Resource Group Name
* @property runLocation This is the preferred geo location for the job to run.
* @property schedules Schedule for running the job definition
* @property state State of the job definition.
* @property userConfirmation Enum to detect if user confirmation is required. If not passed will default to NotRequired.
*/
public data class JobDefinitionArgs(
public val customerSecrets: Output>? = null,
public val dataManagerName: Output? = null,
public val dataServiceInput: Output? = null,
public val dataServiceName: Output? = null,
public val dataSinkId: Output? = null,
public val dataSourceId: Output? = null,
public val jobDefinitionName: Output? = null,
public val lastModifiedTime: Output? = null,
public val resourceGroupName: Output? = null,
public val runLocation: Output? = null,
public val schedules: Output>? = null,
public val state: Output? = null,
public val userConfirmation: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.hybriddata.JobDefinitionArgs =
com.pulumi.azurenative.hybriddata.JobDefinitionArgs.builder()
.customerSecrets(
customerSecrets?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.dataManagerName(dataManagerName?.applyValue({ args0 -> args0 }))
.dataServiceInput(dataServiceInput?.applyValue({ args0 -> args0 }))
.dataServiceName(dataServiceName?.applyValue({ args0 -> args0 }))
.dataSinkId(dataSinkId?.applyValue({ args0 -> args0 }))
.dataSourceId(dataSourceId?.applyValue({ args0 -> args0 }))
.jobDefinitionName(jobDefinitionName?.applyValue({ args0 -> args0 }))
.lastModifiedTime(lastModifiedTime?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.runLocation(runLocation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.schedules(
schedules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.state(state?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.userConfirmation(
userConfirmation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [JobDefinitionArgs].
*/
@PulumiTagMarker
public class JobDefinitionArgsBuilder internal constructor() {
private var customerSecrets: Output>? = null
private var dataManagerName: Output? = null
private var dataServiceInput: Output? = null
private var dataServiceName: Output? = null
private var dataSinkId: Output? = null
private var dataSourceId: Output? = null
private var jobDefinitionName: Output? = null
private var lastModifiedTime: Output? = null
private var resourceGroupName: Output? = null
private var runLocation: Output? = null
private var schedules: Output>? = null
private var state: Output? = null
private var userConfirmation: Output? = null
/**
* @param value List of customer secrets containing a key identifier and key value. The key identifier is a way for the specific data source to understand the key. Value contains customer secret encrypted by the encryptionKeys.
*/
@JvmName("ncklatkgxbewitbf")
public suspend fun customerSecrets(`value`: Output>) {
this.customerSecrets = value
}
@JvmName("snnhoairevygascv")
public suspend fun customerSecrets(vararg values: Output) {
this.customerSecrets = Output.all(values.asList())
}
/**
* @param values List of customer secrets containing a key identifier and key value. The key identifier is a way for the specific data source to understand the key. Value contains customer secret encrypted by the encryptionKeys.
*/
@JvmName("fafjjsyafwhkqvoy")
public suspend fun customerSecrets(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy