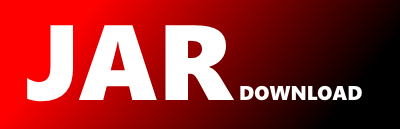
com.pulumi.azurenative.hybridnetwork.kotlin.VendorSkusArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.hybridnetwork.kotlin
import com.pulumi.azurenative.hybridnetwork.VendorSkusArgs.builder
import com.pulumi.azurenative.hybridnetwork.kotlin.enums.NetworkFunctionType
import com.pulumi.azurenative.hybridnetwork.kotlin.enums.SkuDeploymentMode
import com.pulumi.azurenative.hybridnetwork.kotlin.enums.SkuType
import com.pulumi.azurenative.hybridnetwork.kotlin.inputs.NetworkFunctionTemplateArgs
import com.pulumi.azurenative.hybridnetwork.kotlin.inputs.NetworkFunctionTemplateArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Sku sub resource.
* Azure REST API version: 2022-01-01-preview. Prior API version in Azure Native 1.x: 2020-01-01-preview.
* ## Example Usage
* ### Create or update the sku of vendor resource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var vendorSkus = new AzureNative.HybridNetwork.VendorSkus("vendorSkus", new()
* {
* DeploymentMode = AzureNative.HybridNetwork.SkuDeploymentMode.PrivateEdgeZone,
* ManagedApplicationTemplate = null,
* NetworkFunctionTemplate = new AzureNative.HybridNetwork.Inputs.NetworkFunctionTemplateArgs
* {
* NetworkFunctionRoleConfigurations = new[]
* {
* new AzureNative.HybridNetwork.Inputs.NetworkFunctionRoleConfigurationArgs
* {
* CustomProfile = new AzureNative.HybridNetwork.Inputs.CustomProfileArgs
* {
* MetadataConfigurationPath = "/var/logs/network.cfg",
* },
* NetworkInterfaces = new[]
* {
* new AzureNative.HybridNetwork.Inputs.NetworkInterfaceArgs
* {
* IpConfigurations = new[]
* {
* new AzureNative.HybridNetwork.Inputs.NetworkInterfaceIPConfigurationArgs
* {
* Gateway = "",
* IpAddress = "",
* IpAllocationMethod = AzureNative.HybridNetwork.IPAllocationMethod.Dynamic,
* IpVersion = AzureNative.HybridNetwork.IPVersion.IPv4,
* Subnet = "",
* },
* },
* MacAddress = "",
* NetworkInterfaceName = "nic1",
* VmSwitchType = AzureNative.HybridNetwork.VMSwitchType.Wan,
* },
* new AzureNative.HybridNetwork.Inputs.NetworkInterfaceArgs
* {
* IpConfigurations = new[]
* {
* new AzureNative.HybridNetwork.Inputs.NetworkInterfaceIPConfigurationArgs
* {
* Gateway = "",
* IpAddress = "",
* IpAllocationMethod = AzureNative.HybridNetwork.IPAllocationMethod.Dynamic,
* IpVersion = AzureNative.HybridNetwork.IPVersion.IPv4,
* Subnet = "",
* },
* },
* MacAddress = "",
* NetworkInterfaceName = "nic2",
* VmSwitchType = AzureNative.HybridNetwork.VMSwitchType.Management,
* },
* },
* OsProfile = new AzureNative.HybridNetwork.Inputs.OsProfileArgs
* {
* AdminUsername = "dummyuser",
* CustomData = "base-64 encoded string of custom data",
* LinuxConfiguration = new AzureNative.HybridNetwork.Inputs.LinuxConfigurationArgs
* {
* Ssh = new AzureNative.HybridNetwork.Inputs.SshConfigurationArgs
* {
* PublicKeys = new[]
* {
* new AzureNative.HybridNetwork.Inputs.SshPublicKeyArgs
* {
* KeyData = "ssh-rsa AAAAB3NzaC1yc2EAAAABIwAAAgEAwrr66r8n6B8Y0zMF3dOpXEapIQD9DiYQ6D6/zwor9o39jSkHNiMMER/GETBbzP83LOcekm02aRjo55ArO7gPPVvCXbrirJu9pkm4AC4BBre5xSLS= user@constoso-DSH",
* Path = "home/user/.ssh/authorized_keys",
* },
* },
* },
* },
* },
* RoleName = "test",
* RoleType = AzureNative.HybridNetwork.NetworkFunctionRoleConfigurationType.VirtualMachine,
* StorageProfile = new AzureNative.HybridNetwork.Inputs.StorageProfileArgs
* {
* DataDisks = new[]
* {
* new AzureNative.HybridNetwork.Inputs.DataDiskArgs
* {
* CreateOption = AzureNative.HybridNetwork.DiskCreateOptionTypes.Empty,
* DiskSizeGB = 10,
* Name = "DataDisk1",
* },
* },
* ImageReference = new AzureNative.HybridNetwork.Inputs.ImageReferenceArgs
* {
* Offer = "UbuntuServer",
* Publisher = "Canonical",
* Sku = "18.04-LTS",
* Version = "18.04.201804262",
* },
* OsDisk = new AzureNative.HybridNetwork.Inputs.OsDiskArgs
* {
* DiskSizeGB = 30,
* Name = "vhdName",
* OsType = AzureNative.HybridNetwork.OperatingSystemTypes.Linux,
* Vhd = new AzureNative.HybridNetwork.Inputs.VirtualHardDiskArgs
* {
* Uri = "https://contoso.net/link/vnd.vhd?sp=rl&st=2020-10-08T20:38:19Z&se=2020-12-09T19:38:00Z&sv=2019-12-12&sr=b&sig=7BM2f4yOw%3D",
* },
* },
* },
* VirtualMachineSize = AzureNative.HybridNetwork.VirtualMachineSizeTypes.Standard_D3_v2,
* },
* },
* },
* NetworkFunctionType = AzureNative.HybridNetwork.NetworkFunctionType.VirtualNetworkFunction,
* Preview = true,
* SkuName = "TestSku",
* VendorName = "TestVendor",
* });
* });
* ```
* ```go
* package main
* import (
* hybridnetwork "github.com/pulumi/pulumi-azure-native-sdk/hybridnetwork/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := hybridnetwork.NewVendorSkus(ctx, "vendorSkus", &hybridnetwork.VendorSkusArgs{
* DeploymentMode: pulumi.String(hybridnetwork.SkuDeploymentModePrivateEdgeZone),
* ManagedApplicationTemplate: pulumi.Any(nil),
* NetworkFunctionTemplate: &hybridnetwork.NetworkFunctionTemplateArgs{
* NetworkFunctionRoleConfigurations: hybridnetwork.NetworkFunctionRoleConfigurationArray{
* &hybridnetwork.NetworkFunctionRoleConfigurationArgs{
* CustomProfile: &hybridnetwork.CustomProfileArgs{
* MetadataConfigurationPath: pulumi.String("/var/logs/network.cfg"),
* },
* NetworkInterfaces: hybridnetwork.NetworkInterfaceArray{
* &hybridnetwork.NetworkInterfaceArgs{
* IpConfigurations: hybridnetwork.NetworkInterfaceIPConfigurationArray{
* &hybridnetwork.NetworkInterfaceIPConfigurationArgs{
* Gateway: pulumi.String(""),
* IpAddress: pulumi.String(""),
* IpAllocationMethod: pulumi.String(hybridnetwork.IPAllocationMethodDynamic),
* IpVersion: pulumi.String(hybridnetwork.IPVersionIPv4),
* Subnet: pulumi.String(""),
* },
* },
* MacAddress: pulumi.String(""),
* NetworkInterfaceName: pulumi.String("nic1"),
* VmSwitchType: pulumi.String(hybridnetwork.VMSwitchTypeWan),
* },
* &hybridnetwork.NetworkInterfaceArgs{
* IpConfigurations: hybridnetwork.NetworkInterfaceIPConfigurationArray{
* &hybridnetwork.NetworkInterfaceIPConfigurationArgs{
* Gateway: pulumi.String(""),
* IpAddress: pulumi.String(""),
* IpAllocationMethod: pulumi.String(hybridnetwork.IPAllocationMethodDynamic),
* IpVersion: pulumi.String(hybridnetwork.IPVersionIPv4),
* Subnet: pulumi.String(""),
* },
* },
* MacAddress: pulumi.String(""),
* NetworkInterfaceName: pulumi.String("nic2"),
* VmSwitchType: pulumi.String(hybridnetwork.VMSwitchTypeManagement),
* },
* },
* OsProfile: &hybridnetwork.OsProfileArgs{
* AdminUsername: pulumi.String("dummyuser"),
* CustomData: pulumi.String("base-64 encoded string of custom data"),
* LinuxConfiguration: &hybridnetwork.LinuxConfigurationArgs{
* Ssh: &hybridnetwork.SshConfigurationArgs{
* PublicKeys: hybridnetwork.SshPublicKeyArray{
* &hybridnetwork.SshPublicKeyArgs{
* KeyData: pulumi.String("ssh-rsa AAAAB3NzaC1yc2EAAAABIwAAAgEAwrr66r8n6B8Y0zMF3dOpXEapIQD9DiYQ6D6/zwor9o39jSkHNiMMER/GETBbzP83LOcekm02aRjo55ArO7gPPVvCXbrirJu9pkm4AC4BBre5xSLS= user@constoso-DSH"),
* Path: pulumi.String("home/user/.ssh/authorized_keys"),
* },
* },
* },
* },
* },
* RoleName: pulumi.String("test"),
* RoleType: pulumi.String(hybridnetwork.NetworkFunctionRoleConfigurationTypeVirtualMachine),
* StorageProfile: &hybridnetwork.StorageProfileArgs{
* DataDisks: hybridnetwork.DataDiskArray{
* &hybridnetwork.DataDiskArgs{
* CreateOption: pulumi.String(hybridnetwork.DiskCreateOptionTypesEmpty),
* DiskSizeGB: pulumi.Int(10),
* Name: pulumi.String("DataDisk1"),
* },
* },
* ImageReference: &hybridnetwork.ImageReferenceArgs{
* Offer: pulumi.String("UbuntuServer"),
* Publisher: pulumi.String("Canonical"),
* Sku: pulumi.String("18.04-LTS"),
* Version: pulumi.String("18.04.201804262"),
* },
* OsDisk: &hybridnetwork.OsDiskArgs{
* DiskSizeGB: pulumi.Int(30),
* Name: pulumi.String("vhdName"),
* OsType: pulumi.String(hybridnetwork.OperatingSystemTypesLinux),
* Vhd: &hybridnetwork.VirtualHardDiskArgs{
* Uri: pulumi.String("https://contoso.net/link/vnd.vhd?sp=rl&st=2020-10-08T20:38:19Z&se=2020-12-09T19:38:00Z&sv=2019-12-12&sr=b&sig=7BM2f4yOw%3D"),
* },
* },
* },
* VirtualMachineSize: pulumi.String(hybridnetwork.VirtualMachineSizeTypes_Standard_D3_v2),
* },
* },
* },
* NetworkFunctionType: pulumi.String(hybridnetwork.NetworkFunctionTypeVirtualNetworkFunction),
* Preview: pulumi.Bool(true),
* SkuName: pulumi.String("TestSku"),
* VendorName: pulumi.String("TestVendor"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.hybridnetwork.VendorSkus;
* import com.pulumi.azurenative.hybridnetwork.VendorSkusArgs;
* import com.pulumi.azurenative.hybridnetwork.inputs.NetworkFunctionTemplateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var vendorSkus = new VendorSkus("vendorSkus", VendorSkusArgs.builder()
* .deploymentMode("PrivateEdgeZone")
* .managedApplicationTemplate()
* .networkFunctionTemplate(NetworkFunctionTemplateArgs.builder()
* .networkFunctionRoleConfigurations(NetworkFunctionRoleConfigurationArgs.builder()
* .customProfile(CustomProfileArgs.builder()
* .metadataConfigurationPath("/var/logs/network.cfg")
* .build())
* .networkInterfaces(
* NetworkInterfaceArgs.builder()
* .ipConfigurations(NetworkInterfaceIPConfigurationArgs.builder()
* .gateway("")
* .ipAddress("")
* .ipAllocationMethod("Dynamic")
* .ipVersion("IPv4")
* .subnet("")
* .build())
* .macAddress("")
* .networkInterfaceName("nic1")
* .vmSwitchType("Wan")
* .build(),
* NetworkInterfaceArgs.builder()
* .ipConfigurations(NetworkInterfaceIPConfigurationArgs.builder()
* .gateway("")
* .ipAddress("")
* .ipAllocationMethod("Dynamic")
* .ipVersion("IPv4")
* .subnet("")
* .build())
* .macAddress("")
* .networkInterfaceName("nic2")
* .vmSwitchType("Management")
* .build())
* .osProfile(OsProfileArgs.builder()
* .adminUsername("dummyuser")
* .customData("base-64 encoded string of custom data")
* .linuxConfiguration(LinuxConfigurationArgs.builder()
* .ssh(SshConfigurationArgs.builder()
* .publicKeys(SshPublicKeyArgs.builder()
* .keyData("ssh-rsa AAAAB3NzaC1yc2EAAAABIwAAAgEAwrr66r8n6B8Y0zMF3dOpXEapIQD9DiYQ6D6/zwor9o39jSkHNiMMER/GETBbzP83LOcekm02aRjo55ArO7gPPVvCXbrirJu9pkm4AC4BBre5xSLS= user@constoso-DSH")
* .path("home/user/.ssh/authorized_keys")
* .build())
* .build())
* .build())
* .build())
* .roleName("test")
* .roleType("VirtualMachine")
* .storageProfile(StorageProfileArgs.builder()
* .dataDisks(DataDiskArgs.builder()
* .createOption("Empty")
* .diskSizeGB(10)
* .name("DataDisk1")
* .build())
* .imageReference(ImageReferenceArgs.builder()
* .offer("UbuntuServer")
* .publisher("Canonical")
* .sku("18.04-LTS")
* .version("18.04.201804262")
* .build())
* .osDisk(OsDiskArgs.builder()
* .diskSizeGB(30)
* .name("vhdName")
* .osType("Linux")
* .vhd(VirtualHardDiskArgs.builder()
* .uri("https://contoso.net/link/vnd.vhd?sp=rl&st=2020-10-08T20:38:19Z&se=2020-12-09T19:38:00Z&sv=2019-12-12&sr=b&sig=7BM2f4yOw%3D")
* .build())
* .build())
* .build())
* .virtualMachineSize("Standard_D3_v2")
* .build())
* .build())
* .networkFunctionType("VirtualNetworkFunction")
* .preview(true)
* .skuName("TestSku")
* .vendorName("TestVendor")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:hybridnetwork:VendorSkus TestSku /subscriptions/{subscriptionId}/providers/Microsoft.HybridNetwork/vendors/{vendorName}/vendorSkus/{skuName}
* ```
* @property deploymentMode The sku deployment mode.
* @property managedApplicationParameters The parameters for the managed application to be supplied by the vendor.
* @property managedApplicationTemplate The template for the managed application deployment.
* @property networkFunctionTemplate The template definition of the network function.
* @property networkFunctionType The network function type.
* @property preview Indicates if the vendor sku is in preview mode.
* @property skuName The name of the sku.
* @property skuType The sku type.
* @property vendorName The name of the vendor.
*/
public data class VendorSkusArgs(
public val deploymentMode: Output>? = null,
public val managedApplicationParameters: Output? = null,
public val managedApplicationTemplate: Output? = null,
public val networkFunctionTemplate: Output? = null,
public val networkFunctionType: Output>? = null,
public val preview: Output? = null,
public val skuName: Output? = null,
public val skuType: Output>? = null,
public val vendorName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.hybridnetwork.VendorSkusArgs =
com.pulumi.azurenative.hybridnetwork.VendorSkusArgs.builder()
.deploymentMode(
deploymentMode?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.managedApplicationParameters(managedApplicationParameters?.applyValue({ args0 -> args0 }))
.managedApplicationTemplate(managedApplicationTemplate?.applyValue({ args0 -> args0 }))
.networkFunctionTemplate(
networkFunctionTemplate?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.networkFunctionType(
networkFunctionType?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.preview(preview?.applyValue({ args0 -> args0 }))
.skuName(skuName?.applyValue({ args0 -> args0 }))
.skuType(
skuType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.vendorName(vendorName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VendorSkusArgs].
*/
@PulumiTagMarker
public class VendorSkusArgsBuilder internal constructor() {
private var deploymentMode: Output>? = null
private var managedApplicationParameters: Output? = null
private var managedApplicationTemplate: Output? = null
private var networkFunctionTemplate: Output? = null
private var networkFunctionType: Output>? = null
private var preview: Output? = null
private var skuName: Output? = null
private var skuType: Output>? = null
private var vendorName: Output? = null
/**
* @param value The sku deployment mode.
*/
@JvmName("bverslwaglxybcbo")
public suspend fun deploymentMode(`value`: Output>) {
this.deploymentMode = value
}
/**
* @param value The parameters for the managed application to be supplied by the vendor.
*/
@JvmName("outiquojiuvsbxyc")
public suspend fun managedApplicationParameters(`value`: Output) {
this.managedApplicationParameters = value
}
/**
* @param value The template for the managed application deployment.
*/
@JvmName("urvhuqovvnpthkgn")
public suspend fun managedApplicationTemplate(`value`: Output) {
this.managedApplicationTemplate = value
}
/**
* @param value The template definition of the network function.
*/
@JvmName("dkjttdlpyylcyupr")
public suspend fun networkFunctionTemplate(`value`: Output) {
this.networkFunctionTemplate = value
}
/**
* @param value The network function type.
*/
@JvmName("dtendbdikfmccyli")
public suspend fun networkFunctionType(`value`: Output>) {
this.networkFunctionType = value
}
/**
* @param value Indicates if the vendor sku is in preview mode.
*/
@JvmName("qwvlhghgoiucjnch")
public suspend fun preview(`value`: Output) {
this.preview = value
}
/**
* @param value The name of the sku.
*/
@JvmName("hayespqbkrllfage")
public suspend fun skuName(`value`: Output) {
this.skuName = value
}
/**
* @param value The sku type.
*/
@JvmName("kkjrblenegorvxde")
public suspend fun skuType(`value`: Output>) {
this.skuType = value
}
/**
* @param value The name of the vendor.
*/
@JvmName("lkisboxouwspyfdh")
public suspend fun vendorName(`value`: Output) {
this.vendorName = value
}
/**
* @param value The sku deployment mode.
*/
@JvmName("flkbdfcmimhitddo")
public suspend fun deploymentMode(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deploymentMode = mapped
}
/**
* @param value The sku deployment mode.
*/
@JvmName("qlchnfdprbwmnsyb")
public fun deploymentMode(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.deploymentMode = mapped
}
/**
* @param value The sku deployment mode.
*/
@JvmName("bwlelfuhxapalogq")
public fun deploymentMode(`value`: SkuDeploymentMode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.deploymentMode = mapped
}
/**
* @param value The parameters for the managed application to be supplied by the vendor.
*/
@JvmName("kgtncwsrepmuxuyg")
public suspend fun managedApplicationParameters(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedApplicationParameters = mapped
}
/**
* @param value The template for the managed application deployment.
*/
@JvmName("tvdpdumbhvlexftq")
public suspend fun managedApplicationTemplate(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedApplicationTemplate = mapped
}
/**
* @param value The template definition of the network function.
*/
@JvmName("bwwhfavchdmjplxy")
public suspend fun networkFunctionTemplate(`value`: NetworkFunctionTemplateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.networkFunctionTemplate = mapped
}
/**
* @param argument The template definition of the network function.
*/
@JvmName("bkujutpfgneqdrpb")
public suspend fun networkFunctionTemplate(argument: suspend NetworkFunctionTemplateArgsBuilder.() -> Unit) {
val toBeMapped = NetworkFunctionTemplateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.networkFunctionTemplate = mapped
}
/**
* @param value The network function type.
*/
@JvmName("braphlgeuexdffhc")
public suspend fun networkFunctionType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.networkFunctionType = mapped
}
/**
* @param value The network function type.
*/
@JvmName("mmnokrxxokajxqil")
public fun networkFunctionType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.networkFunctionType = mapped
}
/**
* @param value The network function type.
*/
@JvmName("tadboyjvgjtkvwkg")
public fun networkFunctionType(`value`: NetworkFunctionType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.networkFunctionType = mapped
}
/**
* @param value Indicates if the vendor sku is in preview mode.
*/
@JvmName("bgvtcmpcucxffawf")
public suspend fun preview(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preview = mapped
}
/**
* @param value The name of the sku.
*/
@JvmName("wgtyvumkfymecyfu")
public suspend fun skuName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skuName = mapped
}
/**
* @param value The sku type.
*/
@JvmName("gvnmleydivghhtkb")
public suspend fun skuType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skuType = mapped
}
/**
* @param value The sku type.
*/
@JvmName("ggxjkdwitpwocxyx")
public fun skuType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.skuType = mapped
}
/**
* @param value The sku type.
*/
@JvmName("cbmocwuurxxvdlgb")
public fun skuType(`value`: SkuType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.skuType = mapped
}
/**
* @param value The name of the vendor.
*/
@JvmName("ovqlikhlbsqqogee")
public suspend fun vendorName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vendorName = mapped
}
internal fun build(): VendorSkusArgs = VendorSkusArgs(
deploymentMode = deploymentMode,
managedApplicationParameters = managedApplicationParameters,
managedApplicationTemplate = managedApplicationTemplate,
networkFunctionTemplate = networkFunctionTemplate,
networkFunctionType = networkFunctionType,
preview = preview,
skuName = skuName,
skuType = skuType,
vendorName = vendorName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy