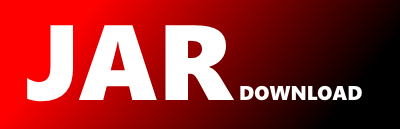
com.pulumi.azurenative.insights.kotlin.ComponentLinkedStorageAccountArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.insights.kotlin
import com.pulumi.azurenative.insights.ComponentLinkedStorageAccountArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* An Application Insights component linked storage accounts
* Azure REST API version: 2020-03-01-preview. Prior API version in Azure Native 1.x: 2020-03-01-preview.
* ## Example Usage
* ### ComponentLinkedStorageAccountsCreateAndUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var componentLinkedStorageAccount = new AzureNative.Insights.ComponentLinkedStorageAccount("componentLinkedStorageAccount", new()
* {
* LinkedStorageAccount = "/subscriptions/86dc51d3-92ed-4d7e-947a-775ea79b4918/resourceGroups/someResourceGroupName/providers/Microsoft.Storage/storageAccounts/storageaccountname",
* ResourceGroupName = "someResourceGroupName",
* ResourceName = "myComponent",
* StorageType = "ServiceProfiler",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewComponentLinkedStorageAccount(ctx, "componentLinkedStorageAccount", &insights.ComponentLinkedStorageAccountArgs{
* LinkedStorageAccount: pulumi.String("/subscriptions/86dc51d3-92ed-4d7e-947a-775ea79b4918/resourceGroups/someResourceGroupName/providers/Microsoft.Storage/storageAccounts/storageaccountname"),
* ResourceGroupName: pulumi.String("someResourceGroupName"),
* ResourceName: pulumi.String("myComponent"),
* StorageType: pulumi.String("ServiceProfiler"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.ComponentLinkedStorageAccount;
* import com.pulumi.azurenative.insights.ComponentLinkedStorageAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var componentLinkedStorageAccount = new ComponentLinkedStorageAccount("componentLinkedStorageAccount", ComponentLinkedStorageAccountArgs.builder()
* .linkedStorageAccount("/subscriptions/86dc51d3-92ed-4d7e-947a-775ea79b4918/resourceGroups/someResourceGroupName/providers/Microsoft.Storage/storageAccounts/storageaccountname")
* .resourceGroupName("someResourceGroupName")
* .resourceName("myComponent")
* .storageType("ServiceProfiler")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:insights:ComponentLinkedStorageAccount serviceprofile /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/microsoft.insights/components/{resourceName}/linkedStorageAccounts/{storageType}
* ```
* @property linkedStorageAccount Linked storage account resource ID
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property resourceName The name of the Application Insights component resource.
* @property storageType The type of the Application Insights component data source for the linked storage account.
*/
public data class ComponentLinkedStorageAccountArgs(
public val linkedStorageAccount: Output? = null,
public val resourceGroupName: Output? = null,
public val resourceName: Output? = null,
public val storageType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.insights.ComponentLinkedStorageAccountArgs =
com.pulumi.azurenative.insights.ComponentLinkedStorageAccountArgs.builder()
.linkedStorageAccount(linkedStorageAccount?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.resourceName(resourceName?.applyValue({ args0 -> args0 }))
.storageType(storageType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ComponentLinkedStorageAccountArgs].
*/
@PulumiTagMarker
public class ComponentLinkedStorageAccountArgsBuilder internal constructor() {
private var linkedStorageAccount: Output? = null
private var resourceGroupName: Output? = null
private var resourceName: Output? = null
private var storageType: Output? = null
/**
* @param value Linked storage account resource ID
*/
@JvmName("odvgjimmnbqiical")
public suspend fun linkedStorageAccount(`value`: Output) {
this.linkedStorageAccount = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("jkqlvlawhjqncgjq")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the Application Insights component resource.
*/
@JvmName("kfhxwohpfwkkopyt")
public suspend fun resourceName(`value`: Output) {
this.resourceName = value
}
/**
* @param value The type of the Application Insights component data source for the linked storage account.
*/
@JvmName("sdnooiivkygioloj")
public suspend fun storageType(`value`: Output) {
this.storageType = value
}
/**
* @param value Linked storage account resource ID
*/
@JvmName("cakokdysuliqyhfj")
public suspend fun linkedStorageAccount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkedStorageAccount = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("inplorpemisvoxtd")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The name of the Application Insights component resource.
*/
@JvmName("ctjqlemosbvgwivv")
public suspend fun resourceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceName = mapped
}
/**
* @param value The type of the Application Insights component data source for the linked storage account.
*/
@JvmName("ylrwcsmuwkpmlrxp")
public suspend fun storageType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageType = mapped
}
internal fun build(): ComponentLinkedStorageAccountArgs = ComponentLinkedStorageAccountArgs(
linkedStorageAccount = linkedStorageAccount,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
storageType = storageType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy