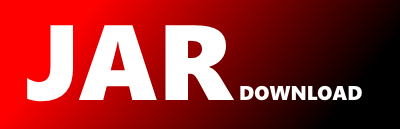
com.pulumi.azurenative.insights.kotlin.MetricAlertArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.insights.kotlin
import com.pulumi.azurenative.insights.MetricAlertArgs.builder
import com.pulumi.azurenative.insights.kotlin.inputs.MetricAlertActionArgs
import com.pulumi.azurenative.insights.kotlin.inputs.MetricAlertActionArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The metric alert resource.
* Azure REST API version: 2018-03-01. Prior API version in Azure Native 1.x: 2018-03-01.
* ## Example Usage
* ### Create or update a dynamic alert rule for Multiple Resources
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertMultipleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.DynamicMetricCriteriaArgs
* {
* AlertSensitivity = AzureNative.Insights.DynamicThresholdSensitivity.Medium,
* CriterionType = "DynamicThresholdCriterion",
* Dimensions = new() { },
* FailingPeriods = new AzureNative.Insights.Inputs.DynamicThresholdFailingPeriodsArgs
* {
* MinFailingPeriodsToAlert = 4,
* NumberOfEvaluationPeriods = 4,
* },
* MetricName = "Percentage CPU",
* MetricNamespace = "microsoft.compute/virtualmachines",
* Name = "High_CPU_80",
* Operator = AzureNative.Insights.DynamicThresholdOperator.GreaterOrLessThan,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "PT1M",
* Location = "global",
* ResourceGroupName = "gigtest",
* RuleName = "MetricAlertOnMultipleResources",
* Scopes = new[]
* {
* "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme1",
* "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme2",
* },
* Severity = 3,
* Tags = null,
* TargetResourceRegion = "southcentralus",
* TargetResourceType = "Microsoft.Compute/virtualMachines",
* WindowSize = "PT15M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertMultipleResourceMultipleMetricCriteriaArgs{
* AllOf: pulumi.Array{
* insights.DynamicMetricCriteria{
* AlertSensitivity: insights.DynamicThresholdSensitivityMedium,
* CriterionType: "DynamicThresholdCriterion",
* Dimensions: []insights.MetricDimension{},
* FailingPeriods: insights.DynamicThresholdFailingPeriods{
* MinFailingPeriodsToAlert: 4,
* NumberOfEvaluationPeriods: 4,
* },
* MetricName: "Percentage CPU",
* MetricNamespace: "microsoft.compute/virtualmachines",
* Name: "High_CPU_80",
* Operator: insights.DynamicThresholdOperatorGreaterOrLessThan,
* TimeAggregation: insights.AggregationTypeEnumAverage,
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1M"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest"),
* RuleName: pulumi.String("MetricAlertOnMultipleResources"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme1"),
* pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme2"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* TargetResourceRegion: pulumi.String("southcentralus"),
* TargetResourceType: pulumi.String("Microsoft.Compute/virtualMachines"),
* WindowSize: pulumi.String("PT15M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .alertSensitivity("Medium")
* .criterionType("DynamicThresholdCriterion")
* .dimensions()
* .failingPeriods(DynamicThresholdFailingPeriodsArgs.builder()
* .minFailingPeriodsToAlert(4)
* .numberOfEvaluationPeriods(4)
* .build())
* .metricName("Percentage CPU")
* .metricNamespace("microsoft.compute/virtualmachines")
* .name("High_CPU_80")
* .operator("GreaterOrLessThan")
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("PT1M")
* .location("global")
* .resourceGroupName("gigtest")
* .ruleName("MetricAlertOnMultipleResources")
* .scopes(
* "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme1",
* "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme2")
* .severity(3)
* .tags()
* .targetResourceRegion("southcentralus")
* .targetResourceType("Microsoft.Compute/virtualMachines")
* .windowSize("PT15M")
* .build());
* }
* }
* ```
* ### Create or update a dynamic alert rule for Single Resource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertMultipleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.DynamicMetricCriteriaArgs
* {
* AlertSensitivity = AzureNative.Insights.DynamicThresholdSensitivity.Medium,
* CriterionType = "DynamicThresholdCriterion",
* Dimensions = new() { },
* FailingPeriods = new AzureNative.Insights.Inputs.DynamicThresholdFailingPeriodsArgs
* {
* MinFailingPeriodsToAlert = 4,
* NumberOfEvaluationPeriods = 4,
* },
* IgnoreDataBefore = "2019-04-04T21:00:00.000Z",
* MetricName = "Percentage CPU",
* MetricNamespace = "microsoft.compute/virtualmachines",
* Name = "High_CPU_80",
* Operator = AzureNative.Insights.DynamicThresholdOperator.GreaterOrLessThan,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "PT1M",
* Location = "global",
* ResourceGroupName = "gigtest",
* RuleName = "chiricutin",
* Scopes = new[]
* {
* "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme",
* },
* Severity = 3,
* Tags = null,
* WindowSize = "PT15M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertMultipleResourceMultipleMetricCriteriaArgs{
* AllOf: pulumi.Array{
* insights.DynamicMetricCriteria{
* AlertSensitivity: insights.DynamicThresholdSensitivityMedium,
* CriterionType: "DynamicThresholdCriterion",
* Dimensions: []insights.MetricDimension{},
* FailingPeriods: insights.DynamicThresholdFailingPeriods{
* MinFailingPeriodsToAlert: 4,
* NumberOfEvaluationPeriods: 4,
* },
* IgnoreDataBefore: "2019-04-04T21:00:00.000Z",
* MetricName: "Percentage CPU",
* MetricNamespace: "microsoft.compute/virtualmachines",
* Name: "High_CPU_80",
* Operator: insights.DynamicThresholdOperatorGreaterOrLessThan,
* TimeAggregation: insights.AggregationTypeEnumAverage,
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1M"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest"),
* RuleName: pulumi.String("chiricutin"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* WindowSize: pulumi.String("PT15M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .alertSensitivity("Medium")
* .criterionType("DynamicThresholdCriterion")
* .dimensions()
* .failingPeriods(DynamicThresholdFailingPeriodsArgs.builder()
* .minFailingPeriodsToAlert(4)
* .numberOfEvaluationPeriods(4)
* .build())
* .ignoreDataBefore("2019-04-04T21:00:00.000Z")
* .metricName("Percentage CPU")
* .metricNamespace("microsoft.compute/virtualmachines")
* .name("High_CPU_80")
* .operator("GreaterOrLessThan")
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("PT1M")
* .location("global")
* .resourceGroupName("gigtest")
* .ruleName("chiricutin")
* .scopes("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme")
* .severity(3)
* .tags()
* .windowSize("PT15M")
* .build());
* }
* }
* ```
* ### Create or update a web test alert rule
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[] {},
* Criteria = new AzureNative.Insights.Inputs.WebtestLocationAvailabilityCriteriaArgs
* {
* ComponentId = "/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example",
* FailedLocationCount = 2,
* OdataType = "Microsoft.Azure.Monitor.WebtestLocationAvailabilityCriteria",
* WebTestId = "/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example",
* },
* Description = "Automatically created alert rule for availability test \"component-example\" a",
* Enabled = true,
* EvaluationFrequency = "PT1M",
* Location = "global",
* ResourceGroupName = "rg-example",
* RuleName = "webtest-name-example",
* Scopes = new[]
* {
* "/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example",
* "/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example",
* },
* Severity = 4,
* Tags =
* {
* { "hidden-link:/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example", "Resource" },
* { "hidden-link:/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example", "Resource" },
* },
* WindowSize = "PT15M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{},
* Criteria: &insights.WebtestLocationAvailabilityCriteriaArgs{
* ComponentId: pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example"),
* FailedLocationCount: pulumi.Float64(2),
* OdataType: pulumi.String("Microsoft.Azure.Monitor.WebtestLocationAvailabilityCriteria"),
* WebTestId: pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example"),
* },
* Description: pulumi.String("Automatically created alert rule for availability test \"component-example\" a"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1M"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("rg-example"),
* RuleName: pulumi.String("webtest-name-example"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example"),
* pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example"),
* },
* Severity: pulumi.Int(4),
* Tags: pulumi.StringMap{
* "hidden-link:/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example": pulumi.String("Resource"),
* "hidden-link:/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example": pulumi.String("Resource"),
* },
* WindowSize: pulumi.String("PT15M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions()
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .componentId("/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example")
* .failedLocationCount(2)
* .odataType("Microsoft.Azure.Monitor.WebtestLocationAvailabilityCriteria")
* .webTestId("/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example")
* .build())
* .description("Automatically created alert rule for availability test \"component-example\" a")
* .enabled(true)
* .evaluationFrequency("PT1M")
* .location("global")
* .resourceGroupName("rg-example")
* .ruleName("webtest-name-example")
* .scopes(
* "/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example",
* "/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example")
* .severity(4)
* .tags(Map.ofEntries(
* Map.entry("hidden-link:/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/components/webtest-name-example", "Resource"),
* Map.entry("hidden-link:/subscriptions/12345678-1234-1234-1234-123456789101/resourcegroups/rg-example/providers/microsoft.insights/webtests/component-example", "Resource")
* ))
* .windowSize("PT15M")
* .build());
* }
* }
* ```
* ### Create or update an alert rule for Multiple Resource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertMultipleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.MetricCriteriaArgs
* {
* CriterionType = "StaticThresholdCriterion",
* Dimensions = new() { },
* MetricName = "Percentage CPU",
* MetricNamespace = "microsoft.compute/virtualmachines",
* Name = "High_CPU_80",
* Operator = AzureNative.Insights.Operator.GreaterThan,
* Threshold = 80.5,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "PT1M",
* Location = "global",
* ResourceGroupName = "gigtest",
* RuleName = "MetricAlertOnMultipleResources",
* Scopes = new[]
* {
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme1",
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme2",
* },
* Severity = 3,
* Tags = null,
* TargetResourceRegion = "southcentralus",
* TargetResourceType = "Microsoft.Compute/virtualMachines",
* WindowSize = "PT15M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertMultipleResourceMultipleMetricCriteriaArgs{
* AllOf: pulumi.Array{
* insights.MetricCriteria{
* CriterionType: "StaticThresholdCriterion",
* Dimensions: []insights.MetricDimension{},
* MetricName: "Percentage CPU",
* MetricNamespace: "microsoft.compute/virtualmachines",
* Name: "High_CPU_80",
* Operator: insights.OperatorGreaterThan,
* Threshold: 80.5,
* TimeAggregation: insights.AggregationTypeEnumAverage,
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1M"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest"),
* RuleName: pulumi.String("MetricAlertOnMultipleResources"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme1"),
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme2"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* TargetResourceRegion: pulumi.String("southcentralus"),
* TargetResourceType: pulumi.String("Microsoft.Compute/virtualMachines"),
* WindowSize: pulumi.String("PT15M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .criterionType("StaticThresholdCriterion")
* .dimensions()
* .metricName("Percentage CPU")
* .metricNamespace("microsoft.compute/virtualmachines")
* .name("High_CPU_80")
* .operator("GreaterThan")
* .threshold(80.5)
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("PT1M")
* .location("global")
* .resourceGroupName("gigtest")
* .ruleName("MetricAlertOnMultipleResources")
* .scopes(
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme1",
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme2")
* .severity(3)
* .tags()
* .targetResourceRegion("southcentralus")
* .targetResourceType("Microsoft.Compute/virtualMachines")
* .windowSize("PT15M")
* .build());
* }
* }
* ```
* ### Create or update an alert rule for Single Resource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertSingleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.MetricCriteriaArgs
* {
* CriterionType = "StaticThresholdCriterion",
* Dimensions = new() { },
* MetricName = "\\Processor(_Total)\\% Processor Time",
* Name = "High_CPU_80",
* Operator = AzureNative.Insights.Operator.GreaterThan,
* Threshold = 80.5,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.SingleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "Pt1m",
* Location = "global",
* ResourceGroupName = "gigtest",
* RuleName = "chiricutin",
* Scopes = new[]
* {
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme",
* },
* Severity = 3,
* Tags = null,
* WindowSize = "Pt15m",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertSingleResourceMultipleMetricCriteriaArgs{
* AllOf: insights.MetricCriteriaArray{
* &insights.MetricCriteriaArgs{
* CriterionType: pulumi.String("StaticThresholdCriterion"),
* Dimensions: insights.MetricDimensionArray{},
* MetricName: pulumi.String("\\Processor(_Total)\\% Processor Time"),
* Name: pulumi.String("High_CPU_80"),
* Operator: pulumi.String(insights.OperatorGreaterThan),
* Threshold: pulumi.Float64(80.5),
* TimeAggregation: pulumi.String(insights.AggregationTypeEnumAverage),
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.SingleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("Pt1m"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest"),
* RuleName: pulumi.String("chiricutin"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* WindowSize: pulumi.String("Pt15m"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .criterionType("StaticThresholdCriterion")
* .dimensions()
* .metricName("\\Processor(_Total)\\% Processor Time")
* .name("High_CPU_80")
* .operator("GreaterThan")
* .threshold(80.5)
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.SingleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("Pt1m")
* .location("global")
* .resourceGroupName("gigtest")
* .ruleName("chiricutin")
* .scopes("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.Compute/virtualMachines/gigwadme")
* .severity(3)
* .tags()
* .windowSize("Pt15m")
* .build());
* }
* }
* ```
* ### Create or update an alert rule on Resource group(s)
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertMultipleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.MetricCriteriaArgs
* {
* CriterionType = "StaticThresholdCriterion",
* Dimensions = new() { },
* MetricName = "Percentage CPU",
* MetricNamespace = "microsoft.compute/virtualmachines",
* Name = "High_CPU_80",
* Operator = AzureNative.Insights.Operator.GreaterThan,
* Threshold = 80.5,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "PT1M",
* Location = "global",
* ResourceGroupName = "gigtest1",
* RuleName = "MetricAlertAtResourceGroupLevel",
* Scopes = new[]
* {
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest1",
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest2",
* },
* Severity = 3,
* Tags = null,
* TargetResourceRegion = "southcentralus",
* TargetResourceType = "Microsoft.Compute/virtualMachines",
* WindowSize = "PT15M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertMultipleResourceMultipleMetricCriteriaArgs{
* AllOf: pulumi.Array{
* insights.MetricCriteria{
* CriterionType: "StaticThresholdCriterion",
* Dimensions: []insights.MetricDimension{},
* MetricName: "Percentage CPU",
* MetricNamespace: "microsoft.compute/virtualmachines",
* Name: "High_CPU_80",
* Operator: insights.OperatorGreaterThan,
* Threshold: 80.5,
* TimeAggregation: insights.AggregationTypeEnumAverage,
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1M"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest1"),
* RuleName: pulumi.String("MetricAlertAtResourceGroupLevel"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest1"),
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest2"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* TargetResourceRegion: pulumi.String("southcentralus"),
* TargetResourceType: pulumi.String("Microsoft.Compute/virtualMachines"),
* WindowSize: pulumi.String("PT15M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .criterionType("StaticThresholdCriterion")
* .dimensions()
* .metricName("Percentage CPU")
* .metricNamespace("microsoft.compute/virtualmachines")
* .name("High_CPU_80")
* .operator("GreaterThan")
* .threshold(80.5)
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("PT1M")
* .location("global")
* .resourceGroupName("gigtest1")
* .ruleName("MetricAlertAtResourceGroupLevel")
* .scopes(
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest1",
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest2")
* .severity(3)
* .tags()
* .targetResourceRegion("southcentralus")
* .targetResourceType("Microsoft.Compute/virtualMachines")
* .windowSize("PT15M")
* .build());
* }
* }
* ```
* ### Create or update an alert rule on Subscription
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertMultipleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.MetricCriteriaArgs
* {
* CriterionType = "StaticThresholdCriterion",
* Dimensions = new() { },
* MetricName = "Percentage CPU",
* MetricNamespace = "microsoft.compute/virtualmachines",
* Name = "High_CPU_80",
* Operator = AzureNative.Insights.Operator.GreaterThan,
* Threshold = 80.5,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "PT1M",
* Location = "global",
* ResourceGroupName = "gigtest",
* RuleName = "MetricAlertAtSubscriptionLevel",
* Scopes = new[]
* {
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7",
* },
* Severity = 3,
* Tags = null,
* TargetResourceRegion = "southcentralus",
* TargetResourceType = "Microsoft.Compute/virtualMachines",
* WindowSize = "PT15M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertMultipleResourceMultipleMetricCriteriaArgs{
* AllOf: pulumi.Array{
* insights.MetricCriteria{
* CriterionType: "StaticThresholdCriterion",
* Dimensions: []insights.MetricDimension{},
* MetricName: "Percentage CPU",
* MetricNamespace: "microsoft.compute/virtualmachines",
* Name: "High_CPU_80",
* Operator: insights.OperatorGreaterThan,
* Threshold: 80.5,
* TimeAggregation: insights.AggregationTypeEnumAverage,
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1M"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest"),
* RuleName: pulumi.String("MetricAlertAtSubscriptionLevel"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* TargetResourceRegion: pulumi.String("southcentralus"),
* TargetResourceType: pulumi.String("Microsoft.Compute/virtualMachines"),
* WindowSize: pulumi.String("PT15M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .criterionType("StaticThresholdCriterion")
* .dimensions()
* .metricName("Percentage CPU")
* .metricNamespace("microsoft.compute/virtualmachines")
* .name("High_CPU_80")
* .operator("GreaterThan")
* .threshold(80.5)
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("PT1M")
* .location("global")
* .resourceGroupName("gigtest")
* .ruleName("MetricAlertAtSubscriptionLevel")
* .scopes("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7")
* .severity(3)
* .tags()
* .targetResourceRegion("southcentralus")
* .targetResourceType("Microsoft.Compute/virtualMachines")
* .windowSize("PT15M")
* .build());
* }
* }
* ```
* ### Create or update an alert rules with dimensions
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var metricAlert = new AzureNative.Insights.MetricAlert("metricAlert", new()
* {
* Actions = new[]
* {
* new AzureNative.Insights.Inputs.MetricAlertActionArgs
* {
* ActionGroupId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2",
* WebHookProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* },
* AutoMitigate = true,
* Criteria = new AzureNative.Insights.Inputs.MetricAlertMultipleResourceMultipleMetricCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.MetricCriteriaArgs
* {
* CriterionType = "StaticThresholdCriterion",
* Dimensions = new[]
* {
* new AzureNative.Insights.Inputs.MetricDimensionArgs
* {
* Name = "ActivityName",
* Operator = "Include",
* Values = new[]
* {
* "*",
* },
* },
* new AzureNative.Insights.Inputs.MetricDimensionArgs
* {
* Name = "StatusCode",
* Operator = "Include",
* Values = new[]
* {
* "200",
* },
* },
* },
* MetricName = "Availability",
* MetricNamespace = "Microsoft.KeyVault/vaults",
* Name = "Metric1",
* Operator = AzureNative.Insights.Operator.GreaterThan,
* Threshold = 55,
* TimeAggregation = AzureNative.Insights.AggregationTypeEnum.Average,
* },
* },
* OdataType = "Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria",
* },
* Description = "This is the description of the rule1",
* Enabled = true,
* EvaluationFrequency = "PT1H",
* Location = "global",
* ResourceGroupName = "gigtest",
* RuleName = "MetricAlertOnMultipleDimensions",
* Scopes = new[]
* {
* "/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.KeyVault/vaults/keyVaultResource",
* },
* Severity = 3,
* Tags = null,
* WindowSize = "P1D",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewMetricAlert(ctx, "metricAlert", &insights.MetricAlertArgs{
* Actions: insights.MetricAlertActionArray{
* &insights.MetricAlertActionArgs{
* ActionGroupId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2"),
* WebHookProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* },
* AutoMitigate: pulumi.Bool(true),
* Criteria: &insights.MetricAlertMultipleResourceMultipleMetricCriteriaArgs{
* AllOf: pulumi.Array{
* insights.MetricCriteria{
* CriterionType: "StaticThresholdCriterion",
* Dimensions: []insights.MetricDimension{
* {
* Name: "ActivityName",
* Operator: "Include",
* Values: []string{
* "*",
* },
* },
* {
* Name: "StatusCode",
* Operator: "Include",
* Values: []string{
* "200",
* },
* },
* },
* MetricName: "Availability",
* MetricNamespace: "Microsoft.KeyVault/vaults",
* Name: "Metric1",
* Operator: insights.OperatorGreaterThan,
* Threshold: 55,
* TimeAggregation: insights.AggregationTypeEnumAverage,
* },
* },
* OdataType: pulumi.String("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria"),
* },
* Description: pulumi.String("This is the description of the rule1"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT1H"),
* Location: pulumi.String("global"),
* ResourceGroupName: pulumi.String("gigtest"),
* RuleName: pulumi.String("MetricAlertOnMultipleDimensions"),
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.KeyVault/vaults/keyVaultResource"),
* },
* Severity: pulumi.Int(3),
* Tags: nil,
* WindowSize: pulumi.String("P1D"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.MetricAlert;
* import com.pulumi.azurenative.insights.MetricAlertArgs;
* import com.pulumi.azurenative.insights.inputs.MetricAlertActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var metricAlert = new MetricAlert("metricAlert", MetricAlertArgs.builder()
* .actions(MetricAlertActionArgs.builder()
* .actionGroupId("/subscriptions/00000000-0000-0000-0000-000000000000/resourcegroups/gigtest/providers/microsoft.insights/actiongroups/group2")
* .webHookProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .autoMitigate(true)
* .criteria(MetricAlertMultipleResourceMultipleMetricCriteriaArgs.builder()
* .allOf(DynamicMetricCriteriaArgs.builder()
* .criterionType("StaticThresholdCriterion")
* .dimensions(
* MetricDimensionArgs.builder()
* .name("ActivityName")
* .operator("Include")
* .values("*")
* .build(),
* MetricDimensionArgs.builder()
* .name("StatusCode")
* .operator("Include")
* .values("200")
* .build())
* .metricName("Availability")
* .metricNamespace("Microsoft.KeyVault/vaults")
* .name("Metric1")
* .operator("GreaterThan")
* .threshold(55)
* .timeAggregation("Average")
* .build())
* .odataType("Microsoft.Azure.Monitor.MultipleResourceMultipleMetricCriteria")
* .build())
* .description("This is the description of the rule1")
* .enabled(true)
* .evaluationFrequency("PT1H")
* .location("global")
* .resourceGroupName("gigtest")
* .ruleName("MetricAlertOnMultipleDimensions")
* .scopes("/subscriptions/14ddf0c5-77c5-4b53-84f6-e1fa43ad68f7/resourceGroups/gigtest/providers/Microsoft.KeyVault/vaults/keyVaultResource")
* .severity(3)
* .tags()
* .windowSize("P1D")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:insights:MetricAlert webtest-name-example /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Insights/metricAlerts/{ruleName}
* ```
* @property actions the array of actions that are performed when the alert rule becomes active, and when an alert condition is resolved.
* @property autoMitigate the flag that indicates whether the alert should be auto resolved or not. The default is true.
* @property criteria defines the specific alert criteria information.
* @property description the description of the metric alert that will be included in the alert email.
* @property enabled the flag that indicates whether the metric alert is enabled.
* @property evaluationFrequency how often the metric alert is evaluated represented in ISO 8601 duration format.
* @property location Resource location
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property ruleName The name of the rule.
* @property scopes the list of resource id's that this metric alert is scoped to.
* @property severity Alert severity {0, 1, 2, 3, 4}
* @property tags Resource tags
* @property targetResourceRegion the region of the target resource(s) on which the alert is created/updated. Mandatory if the scope contains a subscription, resource group, or more than one resource.
* @property targetResourceType the resource type of the target resource(s) on which the alert is created/updated. Mandatory if the scope contains a subscription, resource group, or more than one resource.
* @property windowSize the period of time (in ISO 8601 duration format) that is used to monitor alert activity based on the threshold.
*/
public data class MetricAlertArgs(
public val actions: Output>? = null,
public val autoMitigate: Output? = null,
public val criteria: Output? = null,
public val description: Output? = null,
public val enabled: Output? = null,
public val evaluationFrequency: Output? = null,
public val location: Output? = null,
public val resourceGroupName: Output? = null,
public val ruleName: Output? = null,
public val scopes: Output>? = null,
public val severity: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy