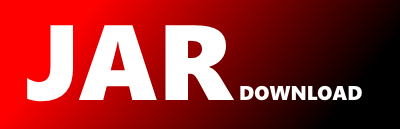
com.pulumi.azurenative.insights.kotlin.ScheduledQueryRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.insights.kotlin
import com.pulumi.azurenative.insights.ScheduledQueryRuleArgs.builder
import com.pulumi.azurenative.insights.kotlin.enums.Kind
import com.pulumi.azurenative.insights.kotlin.inputs.ActionsArgs
import com.pulumi.azurenative.insights.kotlin.inputs.ActionsArgsBuilder
import com.pulumi.azurenative.insights.kotlin.inputs.IdentityArgs
import com.pulumi.azurenative.insights.kotlin.inputs.IdentityArgsBuilder
import com.pulumi.azurenative.insights.kotlin.inputs.RuleResolveConfigurationArgs
import com.pulumi.azurenative.insights.kotlin.inputs.RuleResolveConfigurationArgsBuilder
import com.pulumi.azurenative.insights.kotlin.inputs.ScheduledQueryRuleCriteriaArgs
import com.pulumi.azurenative.insights.kotlin.inputs.ScheduledQueryRuleCriteriaArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The scheduled query rule resource.
* Azure REST API version: 2023-03-15-preview. Prior API version in Azure Native 1.x: 2018-04-16.
* Other available API versions: 2018-04-16, 2020-05-01-preview, 2022-08-01-preview, 2023-12-01.
* ## Example Usage
* ### Create or update a scheduled query rule for Single Resource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledQueryRule = new AzureNative.Insights.ScheduledQueryRule("scheduledQueryRule", new()
* {
* Actions = new AzureNative.Insights.Inputs.ActionsArgs
* {
* ActionGroups = new[]
* {
* "/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup",
* },
* CustomProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* CheckWorkspaceAlertsStorageConfigured = true,
* Criteria = new AzureNative.Insights.Inputs.ScheduledQueryRuleCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.ConditionArgs
* {
* Dimensions = new[]
* {
* new AzureNative.Insights.Inputs.DimensionArgs
* {
* Name = "ComputerIp",
* Operator = AzureNative.Insights.DimensionOperator.Exclude,
* Values = new[]
* {
* "192.168.1.1",
* },
* },
* new AzureNative.Insights.Inputs.DimensionArgs
* {
* Name = "OSType",
* Operator = AzureNative.Insights.DimensionOperator.Include,
* Values = new[]
* {
* "*",
* },
* },
* },
* FailingPeriods = new AzureNative.Insights.Inputs.ConditionFailingPeriodsArgs
* {
* MinFailingPeriodsToAlert = 1,
* NumberOfEvaluationPeriods = 1,
* },
* MetricMeasureColumn = "% Processor Time",
* Operator = AzureNative.Insights.ConditionOperator.GreaterThan,
* Query = "Perf | where ObjectName == \"Processor\"",
* ResourceIdColumn = "resourceId",
* Threshold = 70,
* TimeAggregation = AzureNative.Insights.TimeAggregation.Average,
* },
* },
* },
* Description = "Performance rule",
* Enabled = true,
* EvaluationFrequency = "PT5M",
* Location = "eastus",
* MuteActionsDuration = "PT30M",
* ResourceGroupName = "QueryResourceGroupName",
* RuleName = "perf",
* RuleResolveConfiguration = new AzureNative.Insights.Inputs.RuleResolveConfigurationArgs
* {
* AutoResolved = true,
* TimeToResolve = "PT10M",
* },
* Scopes = new[]
* {
* "/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147/resourceGroups/scopeResourceGroup1/providers/Microsoft.Compute/virtualMachines/vm1",
* },
* Severity = 4,
* SkipQueryValidation = true,
* WindowSize = "PT10M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewScheduledQueryRule(ctx, "scheduledQueryRule", &insights.ScheduledQueryRuleArgs{
* Actions: &insights.ActionsArgs{
* ActionGroups: pulumi.StringArray{
* pulumi.String("/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup"),
* },
* CustomProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* CheckWorkspaceAlertsStorageConfigured: pulumi.Bool(true),
* Criteria: &insights.ScheduledQueryRuleCriteriaArgs{
* AllOf: insights.ConditionArray{
* &insights.ConditionArgs{
* Dimensions: insights.DimensionArray{
* &insights.DimensionArgs{
* Name: pulumi.String("ComputerIp"),
* Operator: pulumi.String(insights.DimensionOperatorExclude),
* Values: pulumi.StringArray{
* pulumi.String("192.168.1.1"),
* },
* },
* &insights.DimensionArgs{
* Name: pulumi.String("OSType"),
* Operator: pulumi.String(insights.DimensionOperatorInclude),
* Values: pulumi.StringArray{
* pulumi.String("*"),
* },
* },
* },
* FailingPeriods: &insights.ConditionFailingPeriodsArgs{
* MinFailingPeriodsToAlert: pulumi.Float64(1),
* NumberOfEvaluationPeriods: pulumi.Float64(1),
* },
* MetricMeasureColumn: pulumi.String("% Processor Time"),
* Operator: pulumi.String(insights.ConditionOperatorGreaterThan),
* Query: pulumi.String("Perf | where ObjectName == \"Processor\""),
* ResourceIdColumn: pulumi.String("resourceId"),
* Threshold: pulumi.Float64(70),
* TimeAggregation: pulumi.String(insights.TimeAggregationAverage),
* },
* },
* },
* Description: pulumi.String("Performance rule"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT5M"),
* Location: pulumi.String("eastus"),
* MuteActionsDuration: pulumi.String("PT30M"),
* ResourceGroupName: pulumi.String("QueryResourceGroupName"),
* RuleName: pulumi.String("perf"),
* RuleResolveConfiguration: &insights.RuleResolveConfigurationArgs{
* AutoResolved: pulumi.Bool(true),
* TimeToResolve: pulumi.String("PT10M"),
* },
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147/resourceGroups/scopeResourceGroup1/providers/Microsoft.Compute/virtualMachines/vm1"),
* },
* Severity: pulumi.Float64(4),
* SkipQueryValidation: pulumi.Bool(true),
* WindowSize: pulumi.String("PT10M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.ScheduledQueryRule;
* import com.pulumi.azurenative.insights.ScheduledQueryRuleArgs;
* import com.pulumi.azurenative.insights.inputs.ActionsArgs;
* import com.pulumi.azurenative.insights.inputs.ScheduledQueryRuleCriteriaArgs;
* import com.pulumi.azurenative.insights.inputs.RuleResolveConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledQueryRule = new ScheduledQueryRule("scheduledQueryRule", ScheduledQueryRuleArgs.builder()
* .actions(ActionsArgs.builder()
* .actionGroups("/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup")
* .customProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .checkWorkspaceAlertsStorageConfigured(true)
* .criteria(ScheduledQueryRuleCriteriaArgs.builder()
* .allOf(ConditionArgs.builder()
* .dimensions(
* DimensionArgs.builder()
* .name("ComputerIp")
* .operator("Exclude")
* .values("192.168.1.1")
* .build(),
* DimensionArgs.builder()
* .name("OSType")
* .operator("Include")
* .values("*")
* .build())
* .failingPeriods(ConditionFailingPeriodsArgs.builder()
* .minFailingPeriodsToAlert(1)
* .numberOfEvaluationPeriods(1)
* .build())
* .metricMeasureColumn("% Processor Time")
* .operator("GreaterThan")
* .query("Perf | where ObjectName == \"Processor\"")
* .resourceIdColumn("resourceId")
* .threshold(70)
* .timeAggregation("Average")
* .build())
* .build())
* .description("Performance rule")
* .enabled(true)
* .evaluationFrequency("PT5M")
* .location("eastus")
* .muteActionsDuration("PT30M")
* .resourceGroupName("QueryResourceGroupName")
* .ruleName("perf")
* .ruleResolveConfiguration(RuleResolveConfigurationArgs.builder()
* .autoResolved(true)
* .timeToResolve("PT10M")
* .build())
* .scopes("/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147/resourceGroups/scopeResourceGroup1/providers/Microsoft.Compute/virtualMachines/vm1")
* .severity(4)
* .skipQueryValidation(true)
* .windowSize("PT10M")
* .build());
* }
* }
* ```
* ### Create or update a scheduled query rule on Resource group(s)
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledQueryRule = new AzureNative.Insights.ScheduledQueryRule("scheduledQueryRule", new()
* {
* Actions = new AzureNative.Insights.Inputs.ActionsArgs
* {
* ActionGroups = new[]
* {
* "/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup",
* },
* CustomProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* CheckWorkspaceAlertsStorageConfigured = true,
* Criteria = new AzureNative.Insights.Inputs.ScheduledQueryRuleCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.ConditionArgs
* {
* Dimensions = new() { },
* FailingPeriods = new AzureNative.Insights.Inputs.ConditionFailingPeriodsArgs
* {
* MinFailingPeriodsToAlert = 1,
* NumberOfEvaluationPeriods = 1,
* },
* Operator = AzureNative.Insights.ConditionOperator.GreaterThan,
* Query = "Heartbeat",
* Threshold = 360,
* TimeAggregation = AzureNative.Insights.TimeAggregation.Count,
* },
* },
* },
* Description = "Health check rule",
* Enabled = true,
* EvaluationFrequency = "PT5M",
* Location = "eastus",
* MuteActionsDuration = "PT30M",
* ResourceGroupName = "QueryResourceGroupName",
* RuleName = "heartbeat",
* RuleResolveConfiguration = new AzureNative.Insights.Inputs.RuleResolveConfigurationArgs
* {
* AutoResolved = true,
* TimeToResolve = "PT10M",
* },
* Scopes = new[]
* {
* "/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147/resourceGroups/scopeResourceGroup1",
* },
* Severity = 4,
* SkipQueryValidation = true,
* TargetResourceTypes = new[]
* {
* "Microsoft.Compute/virtualMachines",
* },
* WindowSize = "PT10M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewScheduledQueryRule(ctx, "scheduledQueryRule", &insights.ScheduledQueryRuleArgs{
* Actions: &insights.ActionsArgs{
* ActionGroups: pulumi.StringArray{
* pulumi.String("/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup"),
* },
* CustomProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* CheckWorkspaceAlertsStorageConfigured: pulumi.Bool(true),
* Criteria: &insights.ScheduledQueryRuleCriteriaArgs{
* AllOf: insights.ConditionArray{
* &insights.ConditionArgs{
* Dimensions: insights.DimensionArray{},
* FailingPeriods: &insights.ConditionFailingPeriodsArgs{
* MinFailingPeriodsToAlert: pulumi.Float64(1),
* NumberOfEvaluationPeriods: pulumi.Float64(1),
* },
* Operator: pulumi.String(insights.ConditionOperatorGreaterThan),
* Query: pulumi.String("Heartbeat"),
* Threshold: pulumi.Float64(360),
* TimeAggregation: pulumi.String(insights.TimeAggregationCount),
* },
* },
* },
* Description: pulumi.String("Health check rule"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT5M"),
* Location: pulumi.String("eastus"),
* MuteActionsDuration: pulumi.String("PT30M"),
* ResourceGroupName: pulumi.String("QueryResourceGroupName"),
* RuleName: pulumi.String("heartbeat"),
* RuleResolveConfiguration: &insights.RuleResolveConfigurationArgs{
* AutoResolved: pulumi.Bool(true),
* TimeToResolve: pulumi.String("PT10M"),
* },
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147/resourceGroups/scopeResourceGroup1"),
* },
* Severity: pulumi.Float64(4),
* SkipQueryValidation: pulumi.Bool(true),
* TargetResourceTypes: pulumi.StringArray{
* pulumi.String("Microsoft.Compute/virtualMachines"),
* },
* WindowSize: pulumi.String("PT10M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.ScheduledQueryRule;
* import com.pulumi.azurenative.insights.ScheduledQueryRuleArgs;
* import com.pulumi.azurenative.insights.inputs.ActionsArgs;
* import com.pulumi.azurenative.insights.inputs.ScheduledQueryRuleCriteriaArgs;
* import com.pulumi.azurenative.insights.inputs.RuleResolveConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledQueryRule = new ScheduledQueryRule("scheduledQueryRule", ScheduledQueryRuleArgs.builder()
* .actions(ActionsArgs.builder()
* .actionGroups("/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup")
* .customProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .checkWorkspaceAlertsStorageConfigured(true)
* .criteria(ScheduledQueryRuleCriteriaArgs.builder()
* .allOf(ConditionArgs.builder()
* .dimensions()
* .failingPeriods(ConditionFailingPeriodsArgs.builder()
* .minFailingPeriodsToAlert(1)
* .numberOfEvaluationPeriods(1)
* .build())
* .operator("GreaterThan")
* .query("Heartbeat")
* .threshold(360)
* .timeAggregation("Count")
* .build())
* .build())
* .description("Health check rule")
* .enabled(true)
* .evaluationFrequency("PT5M")
* .location("eastus")
* .muteActionsDuration("PT30M")
* .resourceGroupName("QueryResourceGroupName")
* .ruleName("heartbeat")
* .ruleResolveConfiguration(RuleResolveConfigurationArgs.builder()
* .autoResolved(true)
* .timeToResolve("PT10M")
* .build())
* .scopes("/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147/resourceGroups/scopeResourceGroup1")
* .severity(4)
* .skipQueryValidation(true)
* .targetResourceTypes("Microsoft.Compute/virtualMachines")
* .windowSize("PT10M")
* .build());
* }
* }
* ```
* ### Create or update a scheduled query rule on Subscription
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledQueryRule = new AzureNative.Insights.ScheduledQueryRule("scheduledQueryRule", new()
* {
* Actions = new AzureNative.Insights.Inputs.ActionsArgs
* {
* ActionGroups = new[]
* {
* "/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup",
* },
* CustomProperties =
* {
* { "key11", "value11" },
* { "key12", "value12" },
* },
* },
* CheckWorkspaceAlertsStorageConfigured = true,
* Criteria = new AzureNative.Insights.Inputs.ScheduledQueryRuleCriteriaArgs
* {
* AllOf = new[]
* {
* new AzureNative.Insights.Inputs.ConditionArgs
* {
* Dimensions = new[]
* {
* new AzureNative.Insights.Inputs.DimensionArgs
* {
* Name = "ComputerIp",
* Operator = AzureNative.Insights.DimensionOperator.Exclude,
* Values = new[]
* {
* "192.168.1.1",
* },
* },
* new AzureNative.Insights.Inputs.DimensionArgs
* {
* Name = "OSType",
* Operator = AzureNative.Insights.DimensionOperator.Include,
* Values = new[]
* {
* "*",
* },
* },
* },
* FailingPeriods = new AzureNative.Insights.Inputs.ConditionFailingPeriodsArgs
* {
* MinFailingPeriodsToAlert = 1,
* NumberOfEvaluationPeriods = 1,
* },
* MetricMeasureColumn = "% Processor Time",
* Operator = AzureNative.Insights.ConditionOperator.GreaterThan,
* Query = "Perf | where ObjectName == \"Processor\"",
* ResourceIdColumn = "resourceId",
* Threshold = 70,
* TimeAggregation = AzureNative.Insights.TimeAggregation.Average,
* },
* },
* },
* Description = "Performance rule",
* Enabled = true,
* EvaluationFrequency = "PT5M",
* Location = "eastus",
* MuteActionsDuration = "PT30M",
* ResourceGroupName = "QueryResourceGroupName",
* RuleName = "perf",
* RuleResolveConfiguration = new AzureNative.Insights.Inputs.RuleResolveConfigurationArgs
* {
* AutoResolved = true,
* TimeToResolve = "PT10M",
* },
* Scopes = new[]
* {
* "/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147",
* },
* Severity = 4,
* SkipQueryValidation = true,
* TargetResourceTypes = new[]
* {
* "Microsoft.Compute/virtualMachines",
* },
* WindowSize = "PT10M",
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewScheduledQueryRule(ctx, "scheduledQueryRule", &insights.ScheduledQueryRuleArgs{
* Actions: &insights.ActionsArgs{
* ActionGroups: pulumi.StringArray{
* pulumi.String("/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup"),
* },
* CustomProperties: pulumi.StringMap{
* "key11": pulumi.String("value11"),
* "key12": pulumi.String("value12"),
* },
* },
* CheckWorkspaceAlertsStorageConfigured: pulumi.Bool(true),
* Criteria: &insights.ScheduledQueryRuleCriteriaArgs{
* AllOf: insights.ConditionArray{
* &insights.ConditionArgs{
* Dimensions: insights.DimensionArray{
* &insights.DimensionArgs{
* Name: pulumi.String("ComputerIp"),
* Operator: pulumi.String(insights.DimensionOperatorExclude),
* Values: pulumi.StringArray{
* pulumi.String("192.168.1.1"),
* },
* },
* &insights.DimensionArgs{
* Name: pulumi.String("OSType"),
* Operator: pulumi.String(insights.DimensionOperatorInclude),
* Values: pulumi.StringArray{
* pulumi.String("*"),
* },
* },
* },
* FailingPeriods: &insights.ConditionFailingPeriodsArgs{
* MinFailingPeriodsToAlert: pulumi.Float64(1),
* NumberOfEvaluationPeriods: pulumi.Float64(1),
* },
* MetricMeasureColumn: pulumi.String("% Processor Time"),
* Operator: pulumi.String(insights.ConditionOperatorGreaterThan),
* Query: pulumi.String("Perf | where ObjectName == \"Processor\""),
* ResourceIdColumn: pulumi.String("resourceId"),
* Threshold: pulumi.Float64(70),
* TimeAggregation: pulumi.String(insights.TimeAggregationAverage),
* },
* },
* },
* Description: pulumi.String("Performance rule"),
* Enabled: pulumi.Bool(true),
* EvaluationFrequency: pulumi.String("PT5M"),
* Location: pulumi.String("eastus"),
* MuteActionsDuration: pulumi.String("PT30M"),
* ResourceGroupName: pulumi.String("QueryResourceGroupName"),
* RuleName: pulumi.String("perf"),
* RuleResolveConfiguration: &insights.RuleResolveConfigurationArgs{
* AutoResolved: pulumi.Bool(true),
* TimeToResolve: pulumi.String("PT10M"),
* },
* Scopes: pulumi.StringArray{
* pulumi.String("/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147"),
* },
* Severity: pulumi.Float64(4),
* SkipQueryValidation: pulumi.Bool(true),
* TargetResourceTypes: pulumi.StringArray{
* pulumi.String("Microsoft.Compute/virtualMachines"),
* },
* WindowSize: pulumi.String("PT10M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.ScheduledQueryRule;
* import com.pulumi.azurenative.insights.ScheduledQueryRuleArgs;
* import com.pulumi.azurenative.insights.inputs.ActionsArgs;
* import com.pulumi.azurenative.insights.inputs.ScheduledQueryRuleCriteriaArgs;
* import com.pulumi.azurenative.insights.inputs.RuleResolveConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledQueryRule = new ScheduledQueryRule("scheduledQueryRule", ScheduledQueryRuleArgs.builder()
* .actions(ActionsArgs.builder()
* .actionGroups("/subscriptions/1cf177ed-1330-4692-80ea-fd3d7783b147/resourcegroups/sqrapi/providers/microsoft.insights/actiongroups/myactiongroup")
* .customProperties(Map.ofEntries(
* Map.entry("key11", "value11"),
* Map.entry("key12", "value12")
* ))
* .build())
* .checkWorkspaceAlertsStorageConfigured(true)
* .criteria(ScheduledQueryRuleCriteriaArgs.builder()
* .allOf(ConditionArgs.builder()
* .dimensions(
* DimensionArgs.builder()
* .name("ComputerIp")
* .operator("Exclude")
* .values("192.168.1.1")
* .build(),
* DimensionArgs.builder()
* .name("OSType")
* .operator("Include")
* .values("*")
* .build())
* .failingPeriods(ConditionFailingPeriodsArgs.builder()
* .minFailingPeriodsToAlert(1)
* .numberOfEvaluationPeriods(1)
* .build())
* .metricMeasureColumn("% Processor Time")
* .operator("GreaterThan")
* .query("Perf | where ObjectName == \"Processor\"")
* .resourceIdColumn("resourceId")
* .threshold(70)
* .timeAggregation("Average")
* .build())
* .build())
* .description("Performance rule")
* .enabled(true)
* .evaluationFrequency("PT5M")
* .location("eastus")
* .muteActionsDuration("PT30M")
* .resourceGroupName("QueryResourceGroupName")
* .ruleName("perf")
* .ruleResolveConfiguration(RuleResolveConfigurationArgs.builder()
* .autoResolved(true)
* .timeToResolve("PT10M")
* .build())
* .scopes("/subscriptions/aaf177ed-1330-a9f2-80ea-fd3d7783b147")
* .severity(4)
* .skipQueryValidation(true)
* .targetResourceTypes("Microsoft.Compute/virtualMachines")
* .windowSize("PT10M")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:insights:ScheduledQueryRule perf /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Insights/scheduledQueryRules/{ruleName}
* ```
* @property actions Actions to invoke when the alert fires.
* @property autoMitigate The flag that indicates whether the alert should be automatically resolved or not. The default is true. Relevant only for rules of the kind LogAlert.
* @property checkWorkspaceAlertsStorageConfigured The flag which indicates whether this scheduled query rule should be stored in the customer's storage. The default is false. Relevant only for rules of the kind LogAlert.
* @property criteria The rule criteria that defines the conditions of the scheduled query rule.
* @property description The description of the scheduled query rule.
* @property displayName The display name of the alert rule
* @property enabled The flag which indicates whether this scheduled query rule is enabled. Value should be true or false
* @property evaluationFrequency How often the scheduled query rule is evaluated represented in ISO 8601 duration format. Relevant and required only for rules of the kind LogAlert.
* @property identity The identity of the resource.
* @property kind Indicates the type of scheduled query rule. The default is LogAlert.
* @property location The geo-location where the resource lives
* @property muteActionsDuration Mute actions for the chosen period of time (in ISO 8601 duration format) after the alert is fired. Relevant only for rules of the kind LogAlert.
* @property overrideQueryTimeRange If specified then overrides the query time range (default is WindowSize*NumberOfEvaluationPeriods). Relevant only for rules of the kind LogAlert.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property ruleName The name of the rule.
* @property ruleResolveConfiguration Defines the configuration for resolving fired alerts. Relevant only for rules of the kind LogAlert.
* @property scopes The list of resource id's that this scheduled query rule is scoped to.
* @property severity Severity of the alert. Should be an integer between [0-4]. Value of 0 is severest. Relevant and required only for rules of the kind LogAlert.
* @property skipQueryValidation The flag which indicates whether the provided query should be validated or not. The default is false. Relevant only for rules of the kind LogAlert.
* @property tags Resource tags.
* @property targetResourceTypes List of resource type of the target resource(s) on which the alert is created/updated. For example if the scope is a resource group and targetResourceTypes is Microsoft.Compute/virtualMachines, then a different alert will be fired for each virtual machine in the resource group which meet the alert criteria. Relevant only for rules of the kind LogAlert
* @property windowSize The period of time (in ISO 8601 duration format) on which the Alert query will be executed (bin size). Relevant and required only for rules of the kind LogAlert.
*/
public data class ScheduledQueryRuleArgs(
public val actions: Output? = null,
public val autoMitigate: Output? = null,
public val checkWorkspaceAlertsStorageConfigured: Output? = null,
public val criteria: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val enabled: Output? = null,
public val evaluationFrequency: Output? = null,
public val identity: Output? = null,
public val kind: Output>? = null,
public val location: Output? = null,
public val muteActionsDuration: Output? = null,
public val overrideQueryTimeRange: Output? = null,
public val resourceGroupName: Output? = null,
public val ruleName: Output? = null,
public val ruleResolveConfiguration: Output? = null,
public val scopes: Output>? = null,
public val severity: Output? = null,
public val skipQueryValidation: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy