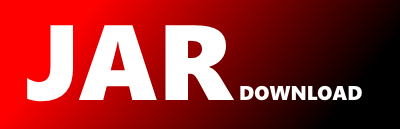
com.pulumi.azurenative.insights.kotlin.WorkbookTemplateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.insights.kotlin
import com.pulumi.azurenative.insights.WorkbookTemplateArgs.builder
import com.pulumi.azurenative.insights.kotlin.inputs.WorkbookTemplateGalleryArgs
import com.pulumi.azurenative.insights.kotlin.inputs.WorkbookTemplateGalleryArgsBuilder
import com.pulumi.azurenative.insights.kotlin.inputs.WorkbookTemplateLocalizedGalleryArgs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* An Application Insights workbook template definition.
* Azure REST API version: 2020-11-20. Prior API version in Azure Native 1.x: 2019-10-17-preview.
* ## Example Usage
* ### WorkbookTemplateAdd
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var workbookTemplate = new AzureNative.Insights.WorkbookTemplate("workbookTemplate", new()
* {
* Author = "Contoso",
* Galleries = new[]
* {
* new AzureNative.Insights.Inputs.WorkbookTemplateGalleryArgs
* {
* Category = "Failures",
* Name = "Simple Template",
* Order = 100,
* ResourceType = "microsoft.insights/components",
* Type = "tsg",
* },
* },
* Location = "west us",
* Priority = 1,
* ResourceGroupName = "my-resource-group",
* ResourceName = "testtemplate2",
* TemplateData = new Dictionary
* {
* ["$schema"] = "https://github.com/Microsoft/Application-Insights-Workbooks/blob/master/schema/workbook.json",
* ["items"] = new[]
* {
* new Dictionary
* {
* ["content"] = new Dictionary
* {
* ["json"] = @"## New workbook
* ---
* Welcome to your new workbook. This area will display text formatted as markdown.
* We've included a basic analytics query to get you started. Use the `Edit` button below each section to configure it or add more sections.",
* },
* ["name"] = "text - 2",
* ["type"] = 1,
* },
* new Dictionary
* {
* ["content"] = new Dictionary
* {
* ["exportToExcelOptions"] = "visible",
* ["query"] = @"union withsource=TableName *
* | summarize Count=count() by TableName
* | render barchart",
* ["queryType"] = 0,
* ["resourceType"] = "microsoft.operationalinsights/workspaces",
* ["size"] = 1,
* ["version"] = "KqlItem/1.0",
* },
* ["name"] = "query - 2",
* ["type"] = 3,
* },
* },
* ["styleSettings"] = new Dictionary
* {
* },
* ["version"] = "Notebook/1.0",
* },
* });
* });
* ```
* ```go
* package main
* import (
* insights "github.com/pulumi/pulumi-azure-native-sdk/insights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := insights.NewWorkbookTemplate(ctx, "workbookTemplate", &insights.WorkbookTemplateArgs{
* Author: pulumi.String("Contoso"),
* Galleries: insights.WorkbookTemplateGalleryArray{
* &insights.WorkbookTemplateGalleryArgs{
* Category: pulumi.String("Failures"),
* Name: pulumi.String("Simple Template"),
* Order: pulumi.Int(100),
* ResourceType: pulumi.String("microsoft.insights/components"),
* Type: pulumi.String("tsg"),
* },
* },
* Location: pulumi.String("west us"),
* Priority: pulumi.Int(1),
* ResourceGroupName: pulumi.String("my-resource-group"),
* ResourceName: pulumi.String("testtemplate2"),
* TemplateData: pulumi.Any(map[string]interface{}{
* "$schema": "https://github.com/Microsoft/Application-Insights-Workbooks/blob/master/schema/workbook.json",
* "items": []interface{}{
* map[string]interface{}{
* "content": map[string]interface{}{
* "json": "## New workbook\n---\n\nWelcome to your new workbook. This area will display text formatted as markdown.\n\n\nWe've included a basic analytics query to get you started. Use the `Edit` button below each section to configure it or add more sections.",
* },
* "name": "text - 2",
* "type": 1,
* },
* map[string]interface{}{
* "content": map[string]interface{}{
* "exportToExcelOptions": "visible",
* "query": "union withsource=TableName *\n| summarize Count=count() by TableName\n| render barchart",
* "queryType": 0,
* "resourceType": "microsoft.operationalinsights/workspaces",
* "size": 1,
* "version": "KqlItem/1.0",
* },
* "name": "query - 2",
* "type": 3,
* },
* },
* "styleSettings": nil,
* "version": "Notebook/1.0",
* }),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.insights.WorkbookTemplate;
* import com.pulumi.azurenative.insights.WorkbookTemplateArgs;
* import com.pulumi.azurenative.insights.inputs.WorkbookTemplateGalleryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var workbookTemplate = new WorkbookTemplate("workbookTemplate", WorkbookTemplateArgs.builder()
* .author("Contoso")
* .galleries(WorkbookTemplateGalleryArgs.builder()
* .category("Failures")
* .name("Simple Template")
* .order(100)
* .resourceType("microsoft.insights/components")
* .type("tsg")
* .build())
* .location("west us")
* .priority(1)
* .resourceGroupName("my-resource-group")
* .resourceName("testtemplate2")
* .templateData(Map.ofEntries(
* Map.entry("$schema", "https://github.com/Microsoft/Application-Insights-Workbooks/blob/master/schema/workbook.json"),
* Map.entry("items",
* Map.ofEntries(
* Map.entry("content", Map.of("json", """
* ## New workbook
* ---
* Welcome to your new workbook. This area will display text formatted as markdown.
* We've included a basic analytics query to get you started. Use the `Edit` button below each section to configure it or add more sections. """)),
* Map.entry("name", "text - 2"),
* Map.entry("type", 1)
* ),
* Map.ofEntries(
* Map.entry("content", Map.ofEntries(
* Map.entry("exportToExcelOptions", "visible"),
* Map.entry("query", """
* union withsource=TableName *
* | summarize Count=count() by TableName
* | render barchart """),
* Map.entry("queryType", 0),
* Map.entry("resourceType", "microsoft.operationalinsights/workspaces"),
* Map.entry("size", 1),
* Map.entry("version", "KqlItem/1.0")
* )),
* Map.entry("name", "query - 2"),
* Map.entry("type", 3)
* )),
* Map.entry("styleSettings", ),
* Map.entry("version", "Notebook/1.0")
* ))
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:insights:WorkbookTemplate testtemplate2 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Insights/workbooktemplates/{resourceName}
* ```
* @property author Information about the author of the workbook template.
* @property galleries Workbook galleries supported by the template.
* @property localized Key value pair of localized gallery. Each key is the locale code of languages supported by the Azure portal.
* @property location Resource location
* @property priority Priority of the template. Determines which template to open when a workbook gallery is opened in viewer mode.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property resourceName The name of the Application Insights component resource.
* @property tags Resource tags
* @property templateData Valid JSON object containing workbook template payload.
*/
public data class WorkbookTemplateArgs(
public val author: Output? = null,
public val galleries: Output>? = null,
public val localized: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy