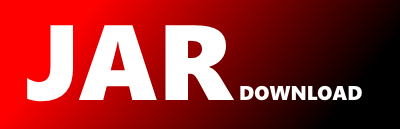
com.pulumi.azurenative.insights.kotlin.inputs.ConditionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.insights.kotlin.inputs
import com.pulumi.azurenative.insights.inputs.ConditionArgs.builder
import com.pulumi.azurenative.insights.kotlin.enums.ConditionOperator
import com.pulumi.azurenative.insights.kotlin.enums.TimeAggregation
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A condition of the scheduled query rule.
* @property dimensions List of Dimensions conditions
* @property failingPeriods The minimum number of violations required within the selected lookback time window required to raise an alert. Relevant only for rules of the kind LogAlert.
* @property metricMeasureColumn The column containing the metric measure number. Relevant only for rules of the kind LogAlert.
* @property metricName The name of the metric to be sent. Relevant and required only for rules of the kind LogToMetric.
* @property operator The criteria operator. Relevant and required only for rules of the kind LogAlert.
* @property query Log query alert
* @property resourceIdColumn The column containing the resource id. The content of the column must be a uri formatted as resource id. Relevant only for rules of the kind LogAlert.
* @property threshold the criteria threshold value that activates the alert. Relevant and required only for rules of the kind LogAlert.
* @property timeAggregation Aggregation type. Relevant and required only for rules of the kind LogAlert.
*/
public data class ConditionArgs(
public val dimensions: Output>? = null,
public val failingPeriods: Output? = null,
public val metricMeasureColumn: Output? = null,
public val metricName: Output? = null,
public val `operator`: Output>? = null,
public val query: Output? = null,
public val resourceIdColumn: Output? = null,
public val threshold: Output? = null,
public val timeAggregation: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.insights.inputs.ConditionArgs =
com.pulumi.azurenative.insights.inputs.ConditionArgs.builder()
.dimensions(
dimensions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.failingPeriods(failingPeriods?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.metricMeasureColumn(metricMeasureColumn?.applyValue({ args0 -> args0 }))
.metricName(metricName?.applyValue({ args0 -> args0 }))
.`operator`(
`operator`?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.query(query?.applyValue({ args0 -> args0 }))
.resourceIdColumn(resourceIdColumn?.applyValue({ args0 -> args0 }))
.threshold(threshold?.applyValue({ args0 -> args0 }))
.timeAggregation(
timeAggregation?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [ConditionArgs].
*/
@PulumiTagMarker
public class ConditionArgsBuilder internal constructor() {
private var dimensions: Output>? = null
private var failingPeriods: Output? = null
private var metricMeasureColumn: Output? = null
private var metricName: Output? = null
private var `operator`: Output>? = null
private var query: Output? = null
private var resourceIdColumn: Output? = null
private var threshold: Output? = null
private var timeAggregation: Output>? = null
/**
* @param value List of Dimensions conditions
*/
@JvmName("ihngamkshkrvyodv")
public suspend fun dimensions(`value`: Output>) {
this.dimensions = value
}
@JvmName("xpggymmsgrnferar")
public suspend fun dimensions(vararg values: Output) {
this.dimensions = Output.all(values.asList())
}
/**
* @param values List of Dimensions conditions
*/
@JvmName("msyqauvhfemcmyiy")
public suspend fun dimensions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy