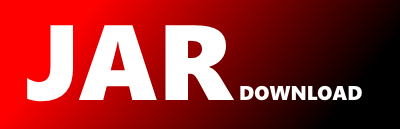
com.pulumi.azurenative.insights.kotlin.inputs.WebhookReceiverArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.insights.kotlin.inputs
import com.pulumi.azurenative.insights.inputs.WebhookReceiverArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A webhook receiver.
* @property identifierUri Indicates the identifier uri for aad auth.
* @property name The name of the webhook receiver. Names must be unique across all receivers within a tenant action group.
* @property objectId Indicates the webhook app object Id for aad auth.
* @property serviceUri The URI where webhooks should be sent.
* @property tenantId Indicates the tenant id for aad auth.
* @property useAadAuth Indicates whether or not use AAD authentication.
* @property useCommonAlertSchema Indicates whether to use common alert schema.
*/
public data class WebhookReceiverArgs(
public val identifierUri: Output? = null,
public val name: Output,
public val objectId: Output? = null,
public val serviceUri: Output,
public val tenantId: Output? = null,
public val useAadAuth: Output? = null,
public val useCommonAlertSchema: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.insights.inputs.WebhookReceiverArgs =
com.pulumi.azurenative.insights.inputs.WebhookReceiverArgs.builder()
.identifierUri(identifierUri?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.objectId(objectId?.applyValue({ args0 -> args0 }))
.serviceUri(serviceUri.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 }))
.useAadAuth(useAadAuth?.applyValue({ args0 -> args0 }))
.useCommonAlertSchema(useCommonAlertSchema?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WebhookReceiverArgs].
*/
@PulumiTagMarker
public class WebhookReceiverArgsBuilder internal constructor() {
private var identifierUri: Output? = null
private var name: Output? = null
private var objectId: Output? = null
private var serviceUri: Output? = null
private var tenantId: Output? = null
private var useAadAuth: Output? = null
private var useCommonAlertSchema: Output? = null
/**
* @param value Indicates the identifier uri for aad auth.
*/
@JvmName("qmwkhbdnbywvucec")
public suspend fun identifierUri(`value`: Output) {
this.identifierUri = value
}
/**
* @param value The name of the webhook receiver. Names must be unique across all receivers within a tenant action group.
*/
@JvmName("sdxfdnqjkhbyysok")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Indicates the webhook app object Id for aad auth.
*/
@JvmName("alllhuyidsjhdcgy")
public suspend fun objectId(`value`: Output) {
this.objectId = value
}
/**
* @param value The URI where webhooks should be sent.
*/
@JvmName("djerfngidijacpmj")
public suspend fun serviceUri(`value`: Output) {
this.serviceUri = value
}
/**
* @param value Indicates the tenant id for aad auth.
*/
@JvmName("warsjnvthwkuvory")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value Indicates whether or not use AAD authentication.
*/
@JvmName("mgdlnyecinbibmvs")
public suspend fun useAadAuth(`value`: Output) {
this.useAadAuth = value
}
/**
* @param value Indicates whether to use common alert schema.
*/
@JvmName("dorrwnuuthnkkleu")
public suspend fun useCommonAlertSchema(`value`: Output) {
this.useCommonAlertSchema = value
}
/**
* @param value Indicates the identifier uri for aad auth.
*/
@JvmName("ussspvhrhslukjeh")
public suspend fun identifierUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identifierUri = mapped
}
/**
* @param value The name of the webhook receiver. Names must be unique across all receivers within a tenant action group.
*/
@JvmName("cgcrpklkggqjncof")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Indicates the webhook app object Id for aad auth.
*/
@JvmName("ndwbjiussfpivmsc")
public suspend fun objectId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.objectId = mapped
}
/**
* @param value The URI where webhooks should be sent.
*/
@JvmName("uwudunlwqvarhovs")
public suspend fun serviceUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.serviceUri = mapped
}
/**
* @param value Indicates the tenant id for aad auth.
*/
@JvmName("ysqtsuaufqtmoqof")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
/**
* @param value Indicates whether or not use AAD authentication.
*/
@JvmName("sodhtfotrxblskfa")
public suspend fun useAadAuth(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useAadAuth = mapped
}
/**
* @param value Indicates whether to use common alert schema.
*/
@JvmName("kmjfgsktbruhgolw")
public suspend fun useCommonAlertSchema(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useCommonAlertSchema = mapped
}
internal fun build(): WebhookReceiverArgs = WebhookReceiverArgs(
identifierUri = identifierUri,
name = name ?: throw PulumiNullFieldException("name"),
objectId = objectId,
serviceUri = serviceUri ?: throw PulumiNullFieldException("serviceUri"),
tenantId = tenantId,
useAadAuth = useAadAuth,
useCommonAlertSchema = useCommonAlertSchema,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy