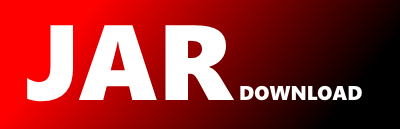
com.pulumi.azurenative.integrationspaces.kotlin.BusinessProcess.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.integrationspaces.kotlin
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.BusinessProcessIdentifierResponse
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.BusinessProcessMappingItemResponse
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.BusinessProcessStageResponse
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.BusinessProcessIdentifierResponse.Companion.toKotlin as businessProcessIdentifierResponseToKotlin
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.BusinessProcessMappingItemResponse.Companion.toKotlin as businessProcessMappingItemResponseToKotlin
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.BusinessProcessStageResponse.Companion.toKotlin as businessProcessStageResponseToKotlin
import com.pulumi.azurenative.integrationspaces.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [BusinessProcess].
*/
@PulumiTagMarker
public class BusinessProcessResourceBuilder internal constructor() {
public var name: String? = null
public var args: BusinessProcessArgs = BusinessProcessArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BusinessProcessArgsBuilder.() -> Unit) {
val builder = BusinessProcessArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BusinessProcess {
val builtJavaResource =
com.pulumi.azurenative.integrationspaces.BusinessProcess(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BusinessProcess(builtJavaResource)
}
}
/**
* A business process under application.
* Azure REST API version: 2023-11-14-preview.
* ## Example Usage
* ### CreateOrUpdateBusinessProcess
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var businessProcess = new AzureNative.IntegrationSpaces.BusinessProcess("businessProcess", new()
* {
* ApplicationName = "Application1",
* BusinessProcessMapping =
* {
* { "Completed", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessMappingItemArgs
* {
* LogicAppResourceId = "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1",
* OperationName = "CompletedPO",
* OperationType = "Action",
* WorkflowName = "Fulfillment",
* } },
* { "Denied", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessMappingItemArgs
* {
* LogicAppResourceId = "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1",
* OperationName = "DeniedPO",
* OperationType = "Action",
* WorkflowName = "Fulfillment",
* } },
* { "Processing", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessMappingItemArgs
* {
* LogicAppResourceId = "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1",
* OperationName = "ApprovedPO",
* OperationType = "Action",
* WorkflowName = "PurchaseOrder",
* } },
* { "Received", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessMappingItemArgs
* {
* LogicAppResourceId = "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1",
* OperationName = "manual",
* OperationType = "Trigger",
* WorkflowName = "PurchaseOrder",
* } },
* { "Shipped", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessMappingItemArgs
* {
* LogicAppResourceId = "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1",
* OperationName = "ShippedPO",
* OperationType = "Action",
* WorkflowName = "Fulfillment",
* } },
* },
* BusinessProcessName = "BusinessProcess1",
* BusinessProcessStages =
* {
* { "Completed", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessStageArgs
* {
* Description = "Completed",
* StagesBefore = new[]
* {
* "Shipped",
* },
* } },
* { "Denied", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessStageArgs
* {
* Description = "Denied",
* StagesBefore = new[]
* {
* "Processing",
* },
* } },
* { "Processing", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessStageArgs
* {
* Description = "Processing",
* Properties =
* {
* { "ApprovalState", "String" },
* { "ApproverName", "String" },
* { "POAmount", "Integer" },
* },
* StagesBefore = new[]
* {
* "Received",
* },
* } },
* { "Received", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessStageArgs
* {
* Description = "received",
* Properties =
* {
* { "City", "String" },
* { "Product", "String" },
* { "Quantity", "Integer" },
* { "State", "String" },
* },
* } },
* { "Shipped", new AzureNative.IntegrationSpaces.Inputs.BusinessProcessStageArgs
* {
* Description = "Shipped",
* Properties =
* {
* { "ShipPriority", "Integer" },
* { "TrackingID", "Integer" },
* },
* StagesBefore = new[]
* {
* "Denied",
* },
* } },
* },
* Description = "First Business Process",
* Identifier = new AzureNative.IntegrationSpaces.Inputs.BusinessProcessIdentifierArgs
* {
* PropertyName = "businessIdentifier-1",
* PropertyType = "String",
* },
* ResourceGroupName = "testrg",
* SpaceName = "Space1",
* TableName = "table1",
* TrackingDataStoreReferenceName = "trackingDataStoreReferenceName1",
* });
* });
* ```
* ```go
* package main
* import (
* integrationspaces "github.com/pulumi/pulumi-azure-native-sdk/integrationspaces/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := integrationspaces.NewBusinessProcess(ctx, "businessProcess", &integrationspaces.BusinessProcessArgs{
* ApplicationName: pulumi.String("Application1"),
* BusinessProcessMapping: integrationspaces.BusinessProcessMappingItemMap{
* "Completed": &integrationspaces.BusinessProcessMappingItemArgs{
* LogicAppResourceId: pulumi.String("subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* OperationName: pulumi.String("CompletedPO"),
* OperationType: pulumi.String("Action"),
* WorkflowName: pulumi.String("Fulfillment"),
* },
* "Denied": &integrationspaces.BusinessProcessMappingItemArgs{
* LogicAppResourceId: pulumi.String("subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* OperationName: pulumi.String("DeniedPO"),
* OperationType: pulumi.String("Action"),
* WorkflowName: pulumi.String("Fulfillment"),
* },
* "Processing": &integrationspaces.BusinessProcessMappingItemArgs{
* LogicAppResourceId: pulumi.String("subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* OperationName: pulumi.String("ApprovedPO"),
* OperationType: pulumi.String("Action"),
* WorkflowName: pulumi.String("PurchaseOrder"),
* },
* "Received": &integrationspaces.BusinessProcessMappingItemArgs{
* LogicAppResourceId: pulumi.String("subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* OperationName: pulumi.String("manual"),
* OperationType: pulumi.String("Trigger"),
* WorkflowName: pulumi.String("PurchaseOrder"),
* },
* "Shipped": &integrationspaces.BusinessProcessMappingItemArgs{
* LogicAppResourceId: pulumi.String("subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* OperationName: pulumi.String("ShippedPO"),
* OperationType: pulumi.String("Action"),
* WorkflowName: pulumi.String("Fulfillment"),
* },
* },
* BusinessProcessName: pulumi.String("BusinessProcess1"),
* BusinessProcessStages: integrationspaces.BusinessProcessStageMap{
* "Completed": &integrationspaces.BusinessProcessStageArgs{
* Description: pulumi.String("Completed"),
* StagesBefore: pulumi.StringArray{
* pulumi.String("Shipped"),
* },
* },
* "Denied": &integrationspaces.BusinessProcessStageArgs{
* Description: pulumi.String("Denied"),
* StagesBefore: pulumi.StringArray{
* pulumi.String("Processing"),
* },
* },
* "Processing": &integrationspaces.BusinessProcessStageArgs{
* Description: pulumi.String("Processing"),
* Properties: pulumi.StringMap{
* "ApprovalState": pulumi.String("String"),
* "ApproverName": pulumi.String("String"),
* "POAmount": pulumi.String("Integer"),
* },
* StagesBefore: pulumi.StringArray{
* pulumi.String("Received"),
* },
* },
* "Received": &integrationspaces.BusinessProcessStageArgs{
* Description: pulumi.String("received"),
* Properties: pulumi.StringMap{
* "City": pulumi.String("String"),
* "Product": pulumi.String("String"),
* "Quantity": pulumi.String("Integer"),
* "State": pulumi.String("String"),
* },
* },
* "Shipped": &integrationspaces.BusinessProcessStageArgs{
* Description: pulumi.String("Shipped"),
* Properties: pulumi.StringMap{
* "ShipPriority": pulumi.String("Integer"),
* "TrackingID": pulumi.String("Integer"),
* },
* StagesBefore: pulumi.StringArray{
* pulumi.String("Denied"),
* },
* },
* },
* Description: pulumi.String("First Business Process"),
* Identifier: &integrationspaces.BusinessProcessIdentifierArgs{
* PropertyName: pulumi.String("businessIdentifier-1"),
* PropertyType: pulumi.String("String"),
* },
* ResourceGroupName: pulumi.String("testrg"),
* SpaceName: pulumi.String("Space1"),
* TableName: pulumi.String("table1"),
* TrackingDataStoreReferenceName: pulumi.String("trackingDataStoreReferenceName1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.integrationspaces.BusinessProcess;
* import com.pulumi.azurenative.integrationspaces.BusinessProcessArgs;
* import com.pulumi.azurenative.integrationspaces.inputs.BusinessProcessIdentifierArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var businessProcess = new BusinessProcess("businessProcess", BusinessProcessArgs.builder()
* .applicationName("Application1")
* .businessProcessMapping(Map.ofEntries(
* Map.entry("Completed", Map.ofEntries(
* Map.entry("logicAppResourceId", "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* Map.entry("operationName", "CompletedPO"),
* Map.entry("operationType", "Action"),
* Map.entry("workflowName", "Fulfillment")
* )),
* Map.entry("Denied", Map.ofEntries(
* Map.entry("logicAppResourceId", "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* Map.entry("operationName", "DeniedPO"),
* Map.entry("operationType", "Action"),
* Map.entry("workflowName", "Fulfillment")
* )),
* Map.entry("Processing", Map.ofEntries(
* Map.entry("logicAppResourceId", "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* Map.entry("operationName", "ApprovedPO"),
* Map.entry("operationType", "Action"),
* Map.entry("workflowName", "PurchaseOrder")
* )),
* Map.entry("Received", Map.ofEntries(
* Map.entry("logicAppResourceId", "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* Map.entry("operationName", "manual"),
* Map.entry("operationType", "Trigger"),
* Map.entry("workflowName", "PurchaseOrder")
* )),
* Map.entry("Shipped", Map.ofEntries(
* Map.entry("logicAppResourceId", "subscriptions/sub1/resourcegroups/group1/providers/Microsoft.Web/sites/logicApp1"),
* Map.entry("operationName", "ShippedPO"),
* Map.entry("operationType", "Action"),
* Map.entry("workflowName", "Fulfillment")
* ))
* ))
* .businessProcessName("BusinessProcess1")
* .businessProcessStages(Map.ofEntries(
* Map.entry("Completed", Map.ofEntries(
* Map.entry("description", "Completed"),
* Map.entry("stagesBefore", "Shipped")
* )),
* Map.entry("Denied", Map.ofEntries(
* Map.entry("description", "Denied"),
* Map.entry("stagesBefore", "Processing")
* )),
* Map.entry("Processing", Map.ofEntries(
* Map.entry("description", "Processing"),
* Map.entry("properties", Map.ofEntries(
* Map.entry("ApprovalState", "String"),
* Map.entry("ApproverName", "String"),
* Map.entry("POAmount", "Integer")
* )),
* Map.entry("stagesBefore", "Received")
* )),
* Map.entry("Received", Map.ofEntries(
* Map.entry("description", "received"),
* Map.entry("properties", Map.ofEntries(
* Map.entry("City", "String"),
* Map.entry("Product", "String"),
* Map.entry("Quantity", "Integer"),
* Map.entry("State", "String")
* ))
* )),
* Map.entry("Shipped", Map.ofEntries(
* Map.entry("description", "Shipped"),
* Map.entry("properties", Map.ofEntries(
* Map.entry("ShipPriority", "Integer"),
* Map.entry("TrackingID", "Integer")
* )),
* Map.entry("stagesBefore", "Denied")
* ))
* ))
* .description("First Business Process")
* .identifier(BusinessProcessIdentifierArgs.builder()
* .propertyName("businessIdentifier-1")
* .propertyType("String")
* .build())
* .resourceGroupName("testrg")
* .spaceName("Space1")
* .tableName("table1")
* .trackingDataStoreReferenceName("trackingDataStoreReferenceName1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:integrationspaces:BusinessProcess BusinessProcess1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.IntegrationSpaces/spaces/{spaceName}/applications/{applicationName}/businessProcesses/{businessProcessName}
* ```
*/
public class BusinessProcess internal constructor(
override val javaResource: com.pulumi.azurenative.integrationspaces.BusinessProcess,
) : KotlinCustomResource(javaResource, BusinessProcessMapper) {
/**
* The business process mapping.
*/
public val businessProcessMapping: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy