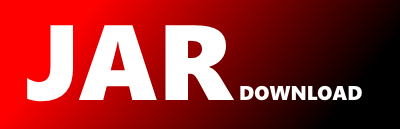
com.pulumi.azurenative.iotcentral.kotlin.IotcentralFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.iotcentral.kotlin
import com.pulumi.azurenative.iotcentral.IotcentralFunctions.getAppPlain
import com.pulumi.azurenative.iotcentral.IotcentralFunctions.getPrivateEndpointConnectionPlain
import com.pulumi.azurenative.iotcentral.kotlin.inputs.GetAppPlainArgs
import com.pulumi.azurenative.iotcentral.kotlin.inputs.GetAppPlainArgsBuilder
import com.pulumi.azurenative.iotcentral.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs
import com.pulumi.azurenative.iotcentral.kotlin.inputs.GetPrivateEndpointConnectionPlainArgsBuilder
import com.pulumi.azurenative.iotcentral.kotlin.outputs.GetAppResult
import com.pulumi.azurenative.iotcentral.kotlin.outputs.GetPrivateEndpointConnectionResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.iotcentral.kotlin.outputs.GetAppResult.Companion.toKotlin as getAppResultToKotlin
import com.pulumi.azurenative.iotcentral.kotlin.outputs.GetPrivateEndpointConnectionResult.Companion.toKotlin as getPrivateEndpointConnectionResultToKotlin
public object IotcentralFunctions {
/**
* Get the metadata of an IoT Central application.
* Azure REST API version: 2021-06-01.
* Other available API versions: 2018-09-01, 2021-11-01-preview.
* @param argument null
* @return The IoT Central application.
*/
public suspend fun getApp(argument: GetAppPlainArgs): GetAppResult =
getAppResultToKotlin(getAppPlain(argument.toJava()).await())
/**
* @see [getApp].
* @param resourceGroupName The name of the resource group that contains the IoT Central application.
* @param resourceName The ARM resource name of the IoT Central application.
* @return The IoT Central application.
*/
public suspend fun getApp(resourceGroupName: String, resourceName: String): GetAppResult {
val argument = GetAppPlainArgs(
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getAppResultToKotlin(getAppPlain(argument.toJava()).await())
}
/**
* @see [getApp].
* @param argument Builder for [com.pulumi.azurenative.iotcentral.kotlin.inputs.GetAppPlainArgs].
* @return The IoT Central application.
*/
public suspend fun getApp(argument: suspend GetAppPlainArgsBuilder.() -> Unit): GetAppResult {
val builder = GetAppPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAppResultToKotlin(getAppPlain(builtArgument.toJava()).await())
}
/**
* Get the metadata of a private endpoint connection for the IoT Central Application.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return The private endpoint connection resource.
*/
public suspend fun getPrivateEndpointConnection(argument: GetPrivateEndpointConnectionPlainArgs): GetPrivateEndpointConnectionResult =
getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
/**
* @see [getPrivateEndpointConnection].
* @param privateEndpointConnectionName The private endpoint connection name.
* @param resourceGroupName The name of the resource group that contains the IoT Central application.
* @param resourceName The ARM resource name of the IoT Central application.
* @return The private endpoint connection resource.
*/
public suspend fun getPrivateEndpointConnection(
privateEndpointConnectionName: String,
resourceGroupName: String,
resourceName: String,
): GetPrivateEndpointConnectionResult {
val argument = GetPrivateEndpointConnectionPlainArgs(
privateEndpointConnectionName = privateEndpointConnectionName,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
}
/**
* @see [getPrivateEndpointConnection].
* @param argument Builder for [com.pulumi.azurenative.iotcentral.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs].
* @return The private endpoint connection resource.
*/
public suspend fun getPrivateEndpointConnection(argument: suspend GetPrivateEndpointConnectionPlainArgsBuilder.() -> Unit): GetPrivateEndpointConnectionResult {
val builder = GetPrivateEndpointConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy