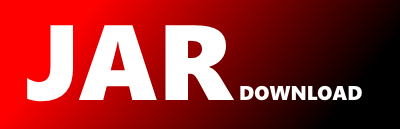
com.pulumi.azurenative.iotfirmwaredefense.kotlin.FirmwareArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.iotfirmwaredefense.kotlin
import com.pulumi.azurenative.iotfirmwaredefense.FirmwareArgs.builder
import com.pulumi.azurenative.iotfirmwaredefense.kotlin.enums.Status
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Firmware definition
* Azure REST API version: 2023-02-08-preview.
* Other available API versions: 2024-01-10.
* ## Example Usage
* ### Firmware_Create_MaximumSet_Gen
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var firmware = new AzureNative.IoTFirmwareDefense.Firmware("firmware", new()
* {
* Description = "uz",
* FileName = "wresexxulcdsdd",
* FileSize = 17,
* FirmwareId = "umrkdttp",
* Model = "f",
* ResourceGroupName = "rgworkspaces-firmwares",
* Status = AzureNative.IoTFirmwareDefense.Status.Pending,
* StatusMessages = new[]
* {
* new Dictionary
* {
* ["message"] = "ulvhmhokezathzzauiitu",
* },
* },
* Vendor = "vycmdhgtmepcptyoubztiuudpkcpd",
* Version = "s",
* WorkspaceName = "A7",
* });
* });
* ```
* ```go
* package main
* import (
* iotfirmwaredefense "github.com/pulumi/pulumi-azure-native-sdk/iotfirmwaredefense/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := iotfirmwaredefense.NewFirmware(ctx, "firmware", &iotfirmwaredefense.FirmwareArgs{
* Description: pulumi.String("uz"),
* FileName: pulumi.String("wresexxulcdsdd"),
* FileSize: pulumi.Float64(17),
* FirmwareId: pulumi.String("umrkdttp"),
* Model: pulumi.String("f"),
* ResourceGroupName: pulumi.String("rgworkspaces-firmwares"),
* Status: pulumi.String(iotfirmwaredefense.StatusPending),
* StatusMessages: pulumi.Array{
* pulumi.Any(map[string]interface{}{
* "message": "ulvhmhokezathzzauiitu",
* }),
* },
* Vendor: pulumi.String("vycmdhgtmepcptyoubztiuudpkcpd"),
* Version: pulumi.String("s"),
* WorkspaceName: pulumi.String("A7"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.iotfirmwaredefense.Firmware;
* import com.pulumi.azurenative.iotfirmwaredefense.FirmwareArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var firmware = new Firmware("firmware", FirmwareArgs.builder()
* .description("uz")
* .fileName("wresexxulcdsdd")
* .fileSize(17)
* .firmwareId("umrkdttp")
* .model("f")
* .resourceGroupName("rgworkspaces-firmwares")
* .status("Pending")
* .statusMessages(Map.of("message", "ulvhmhokezathzzauiitu"))
* .vendor("vycmdhgtmepcptyoubztiuudpkcpd")
* .version("s")
* .workspaceName("A7")
* .build());
* }
* }
* ```
* ### Firmware_Create_MinimumSet_Gen
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var firmware = new AzureNative.IoTFirmwareDefense.Firmware("firmware", new()
* {
* FirmwareId = "umrkdttp",
* ResourceGroupName = "rgworkspaces-firmwares",
* WorkspaceName = "A7",
* });
* });
* ```
* ```go
* package main
* import (
* iotfirmwaredefense "github.com/pulumi/pulumi-azure-native-sdk/iotfirmwaredefense/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := iotfirmwaredefense.NewFirmware(ctx, "firmware", &iotfirmwaredefense.FirmwareArgs{
* FirmwareId: pulumi.String("umrkdttp"),
* ResourceGroupName: pulumi.String("rgworkspaces-firmwares"),
* WorkspaceName: pulumi.String("A7"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.iotfirmwaredefense.Firmware;
* import com.pulumi.azurenative.iotfirmwaredefense.FirmwareArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var firmware = new Firmware("firmware", FirmwareArgs.builder()
* .firmwareId("umrkdttp")
* .resourceGroupName("rgworkspaces-firmwares")
* .workspaceName("A7")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:iotfirmwaredefense:Firmware brmvnojpmxsgckdviynhxhftvcvbw /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.IoTFirmwareDefense/workspaces/{workspaceName}/firmwares/{firmwareId}
* ```
* @property description User-specified description of the firmware.
* @property fileName File name for a firmware that user uploaded.
* @property fileSize File size of the uploaded firmware image.
* @property firmwareId The id of the firmware.
* @property model Firmware model.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property status The status of firmware scan.
* @property statusMessages A list of errors or other messages generated during firmware analysis
* @property vendor Firmware vendor.
* @property version Firmware version.
* @property workspaceName The name of the firmware analysis workspace.
*/
public data class FirmwareArgs(
public val description: Output? = null,
public val fileName: Output? = null,
public val fileSize: Output? = null,
public val firmwareId: Output? = null,
public val model: Output? = null,
public val resourceGroupName: Output? = null,
public val status: Output>? = null,
public val statusMessages: Output>? = null,
public val vendor: Output? = null,
public val version: Output? = null,
public val workspaceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.iotfirmwaredefense.FirmwareArgs =
com.pulumi.azurenative.iotfirmwaredefense.FirmwareArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.fileName(fileName?.applyValue({ args0 -> args0 }))
.fileSize(fileSize?.applyValue({ args0 -> args0 }))
.firmwareId(firmwareId?.applyValue({ args0 -> args0 }))
.model(model?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.status(
status?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.statusMessages(statusMessages?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.vendor(vendor?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 }))
.workspaceName(workspaceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FirmwareArgs].
*/
@PulumiTagMarker
public class FirmwareArgsBuilder internal constructor() {
private var description: Output? = null
private var fileName: Output? = null
private var fileSize: Output? = null
private var firmwareId: Output? = null
private var model: Output? = null
private var resourceGroupName: Output? = null
private var status: Output>? = null
private var statusMessages: Output>? = null
private var vendor: Output? = null
private var version: Output? = null
private var workspaceName: Output? = null
/**
* @param value User-specified description of the firmware.
*/
@JvmName("xgsmkgkdqcxvalxc")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value File name for a firmware that user uploaded.
*/
@JvmName("uxfamstnvgngrqqb")
public suspend fun fileName(`value`: Output) {
this.fileName = value
}
/**
* @param value File size of the uploaded firmware image.
*/
@JvmName("planvxparsaqrkkp")
public suspend fun fileSize(`value`: Output) {
this.fileSize = value
}
/**
* @param value The id of the firmware.
*/
@JvmName("xjqpvphatpsaaytn")
public suspend fun firmwareId(`value`: Output) {
this.firmwareId = value
}
/**
* @param value Firmware model.
*/
@JvmName("vywcbuhaljriswai")
public suspend fun model(`value`: Output) {
this.model = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("qogmetgoxqdmfbmq")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The status of firmware scan.
*/
@JvmName("lxucurvsgueyrfja")
public suspend fun status(`value`: Output>) {
this.status = value
}
/**
* @param value A list of errors or other messages generated during firmware analysis
*/
@JvmName("jbxujthqhlbfaxgk")
public suspend fun statusMessages(`value`: Output>) {
this.statusMessages = value
}
@JvmName("mewjmmyiskfsrmee")
public suspend fun statusMessages(vararg values: Output) {
this.statusMessages = Output.all(values.asList())
}
/**
* @param values A list of errors or other messages generated during firmware analysis
*/
@JvmName("lkubpcatrdrhcgna")
public suspend fun statusMessages(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy