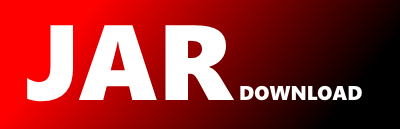
com.pulumi.azurenative.iotoperations.kotlin.inputs.BrokerPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.iotoperations.kotlin.inputs
import com.pulumi.azurenative.iotoperations.inputs.BrokerPropertiesArgs.builder
import com.pulumi.azurenative.iotoperations.kotlin.enums.BrokerMemoryProfile
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Broker Resource properties
* @property advanced Advanced settings of Broker.
* @property cardinality The cardinality details of the broker.
* @property diagnostics Spec defines the desired identities of Broker diagnostics settings.
* @property diskBackedMessageBuffer Settings of Disk Backed Message Buffer.
* @property generateResourceLimits This setting controls whether Kubernetes CPU resource limits are requested. Increasing the number of replicas or workers proportionally increases the amount of CPU resources requested. If this setting is enabled and there are insufficient CPU resources, an error will be emitted.
* @property memoryProfile Memory profile of Broker.
*/
public data class BrokerPropertiesArgs(
public val advanced: Output? = null,
public val cardinality: Output? = null,
public val diagnostics: Output? = null,
public val diskBackedMessageBuffer: Output? = null,
public val generateResourceLimits: Output? = null,
public val memoryProfile: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.iotoperations.inputs.BrokerPropertiesArgs =
com.pulumi.azurenative.iotoperations.inputs.BrokerPropertiesArgs.builder()
.advanced(advanced?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cardinality(cardinality?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.diagnostics(diagnostics?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.diskBackedMessageBuffer(
diskBackedMessageBuffer?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.generateResourceLimits(
generateResourceLimits?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.memoryProfile(
memoryProfile?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [BrokerPropertiesArgs].
*/
@PulumiTagMarker
public class BrokerPropertiesArgsBuilder internal constructor() {
private var advanced: Output? = null
private var cardinality: Output? = null
private var diagnostics: Output? = null
private var diskBackedMessageBuffer: Output? = null
private var generateResourceLimits: Output? = null
private var memoryProfile: Output>? = null
/**
* @param value Advanced settings of Broker.
*/
@JvmName("kmeducejnruiuhiq")
public suspend fun advanced(`value`: Output) {
this.advanced = value
}
/**
* @param value The cardinality details of the broker.
*/
@JvmName("rlxsghmrgdnfqsvw")
public suspend fun cardinality(`value`: Output) {
this.cardinality = value
}
/**
* @param value Spec defines the desired identities of Broker diagnostics settings.
*/
@JvmName("xslnqrfkwksnftbn")
public suspend fun diagnostics(`value`: Output) {
this.diagnostics = value
}
/**
* @param value Settings of Disk Backed Message Buffer.
*/
@JvmName("eceogpljplgkvwfc")
public suspend fun diskBackedMessageBuffer(`value`: Output) {
this.diskBackedMessageBuffer = value
}
/**
* @param value This setting controls whether Kubernetes CPU resource limits are requested. Increasing the number of replicas or workers proportionally increases the amount of CPU resources requested. If this setting is enabled and there are insufficient CPU resources, an error will be emitted.
*/
@JvmName("wopycvpyajlwotid")
public suspend fun generateResourceLimits(`value`: Output) {
this.generateResourceLimits = value
}
/**
* @param value Memory profile of Broker.
*/
@JvmName("osqqtxlfgdksnpnk")
public suspend fun memoryProfile(`value`: Output>) {
this.memoryProfile = value
}
/**
* @param value Advanced settings of Broker.
*/
@JvmName("ydeqelmjdtefqcbe")
public suspend fun advanced(`value`: AdvancedSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.advanced = mapped
}
/**
* @param argument Advanced settings of Broker.
*/
@JvmName("eaaivbvfamifkfqw")
public suspend fun advanced(argument: suspend AdvancedSettingsArgsBuilder.() -> Unit) {
val toBeMapped = AdvancedSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.advanced = mapped
}
/**
* @param value The cardinality details of the broker.
*/
@JvmName("dvrvjnfagntnvpqu")
public suspend fun cardinality(`value`: CardinalityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cardinality = mapped
}
/**
* @param argument The cardinality details of the broker.
*/
@JvmName("yoldritrrsxuabjw")
public suspend fun cardinality(argument: suspend CardinalityArgsBuilder.() -> Unit) {
val toBeMapped = CardinalityArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.cardinality = mapped
}
/**
* @param value Spec defines the desired identities of Broker diagnostics settings.
*/
@JvmName("duojlynuyrdkhonm")
public suspend fun diagnostics(`value`: BrokerDiagnosticsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diagnostics = mapped
}
/**
* @param argument Spec defines the desired identities of Broker diagnostics settings.
*/
@JvmName("nloglstlwjgtjwhj")
public suspend fun diagnostics(argument: suspend BrokerDiagnosticsArgsBuilder.() -> Unit) {
val toBeMapped = BrokerDiagnosticsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.diagnostics = mapped
}
/**
* @param value Settings of Disk Backed Message Buffer.
*/
@JvmName("rmkgrbmgatrtyiuh")
public suspend fun diskBackedMessageBuffer(`value`: DiskBackedMessageBufferArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskBackedMessageBuffer = mapped
}
/**
* @param argument Settings of Disk Backed Message Buffer.
*/
@JvmName("avaaexaxcsrochvy")
public suspend fun diskBackedMessageBuffer(argument: suspend DiskBackedMessageBufferArgsBuilder.() -> Unit) {
val toBeMapped = DiskBackedMessageBufferArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.diskBackedMessageBuffer = mapped
}
/**
* @param value This setting controls whether Kubernetes CPU resource limits are requested. Increasing the number of replicas or workers proportionally increases the amount of CPU resources requested. If this setting is enabled and there are insufficient CPU resources, an error will be emitted.
*/
@JvmName("vsbjtannoaqwdbaj")
public suspend fun generateResourceLimits(`value`: GenerateResourceLimitsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.generateResourceLimits = mapped
}
/**
* @param argument This setting controls whether Kubernetes CPU resource limits are requested. Increasing the number of replicas or workers proportionally increases the amount of CPU resources requested. If this setting is enabled and there are insufficient CPU resources, an error will be emitted.
*/
@JvmName("weclumooraxhiwss")
public suspend fun generateResourceLimits(argument: suspend GenerateResourceLimitsArgsBuilder.() -> Unit) {
val toBeMapped = GenerateResourceLimitsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.generateResourceLimits = mapped
}
/**
* @param value Memory profile of Broker.
*/
@JvmName("ehfkxaimjxnwldug")
public suspend fun memoryProfile(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.memoryProfile = mapped
}
/**
* @param value Memory profile of Broker.
*/
@JvmName("mdmtxkwevrdyiyod")
public fun memoryProfile(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.memoryProfile = mapped
}
/**
* @param value Memory profile of Broker.
*/
@JvmName("wmwtswdvracdskfi")
public fun memoryProfile(`value`: BrokerMemoryProfile) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.memoryProfile = mapped
}
internal fun build(): BrokerPropertiesArgs = BrokerPropertiesArgs(
advanced = advanced,
cardinality = cardinality,
diagnostics = diagnostics,
diskBackedMessageBuffer = diskBackedMessageBuffer,
generateResourceLimits = generateResourceLimits,
memoryProfile = memoryProfile,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy