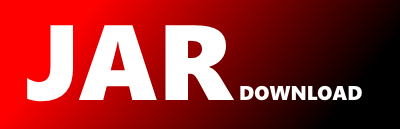
com.pulumi.azurenative.iotoperations.kotlin.inputs.ClientConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.iotoperations.kotlin.inputs
import com.pulumi.azurenative.iotoperations.inputs.ClientConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The settings of Client Config.
* @property maxKeepAliveSeconds Upper bound of a client's Keep Alive, in seconds.
* @property maxMessageExpirySeconds Upper bound of Message Expiry Interval, in seconds.
* @property maxPacketSizeBytes Max message size for a packet in Bytes.
* @property maxReceiveMaximum Upper bound of Receive Maximum that a client can request in the CONNECT packet.
* @property maxSessionExpirySeconds Upper bound of Session Expiry Interval, in seconds.
* @property subscriberQueueLimit The limit on the number of queued messages for a subscriber.
*/
public data class ClientConfigArgs(
public val maxKeepAliveSeconds: Output? = null,
public val maxMessageExpirySeconds: Output? = null,
public val maxPacketSizeBytes: Output? = null,
public val maxReceiveMaximum: Output? = null,
public val maxSessionExpirySeconds: Output? = null,
public val subscriberQueueLimit: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.iotoperations.inputs.ClientConfigArgs =
com.pulumi.azurenative.iotoperations.inputs.ClientConfigArgs.builder()
.maxKeepAliveSeconds(maxKeepAliveSeconds?.applyValue({ args0 -> args0 }))
.maxMessageExpirySeconds(maxMessageExpirySeconds?.applyValue({ args0 -> args0 }))
.maxPacketSizeBytes(maxPacketSizeBytes?.applyValue({ args0 -> args0 }))
.maxReceiveMaximum(maxReceiveMaximum?.applyValue({ args0 -> args0 }))
.maxSessionExpirySeconds(maxSessionExpirySeconds?.applyValue({ args0 -> args0 }))
.subscriberQueueLimit(
subscriberQueueLimit?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ClientConfigArgs].
*/
@PulumiTagMarker
public class ClientConfigArgsBuilder internal constructor() {
private var maxKeepAliveSeconds: Output? = null
private var maxMessageExpirySeconds: Output? = null
private var maxPacketSizeBytes: Output? = null
private var maxReceiveMaximum: Output? = null
private var maxSessionExpirySeconds: Output? = null
private var subscriberQueueLimit: Output? = null
/**
* @param value Upper bound of a client's Keep Alive, in seconds.
*/
@JvmName("xyohigsbxfmtsjvu")
public suspend fun maxKeepAliveSeconds(`value`: Output) {
this.maxKeepAliveSeconds = value
}
/**
* @param value Upper bound of Message Expiry Interval, in seconds.
*/
@JvmName("cngqgrjdkxqfapqm")
public suspend fun maxMessageExpirySeconds(`value`: Output) {
this.maxMessageExpirySeconds = value
}
/**
* @param value Max message size for a packet in Bytes.
*/
@JvmName("rhaykwdvcsemrype")
public suspend fun maxPacketSizeBytes(`value`: Output) {
this.maxPacketSizeBytes = value
}
/**
* @param value Upper bound of Receive Maximum that a client can request in the CONNECT packet.
*/
@JvmName("sgatgychfawuyqua")
public suspend fun maxReceiveMaximum(`value`: Output) {
this.maxReceiveMaximum = value
}
/**
* @param value Upper bound of Session Expiry Interval, in seconds.
*/
@JvmName("kvfskqdoiknyfggg")
public suspend fun maxSessionExpirySeconds(`value`: Output) {
this.maxSessionExpirySeconds = value
}
/**
* @param value The limit on the number of queued messages for a subscriber.
*/
@JvmName("hcrcrqswcpkmswli")
public suspend fun subscriberQueueLimit(`value`: Output) {
this.subscriberQueueLimit = value
}
/**
* @param value Upper bound of a client's Keep Alive, in seconds.
*/
@JvmName("chaepwlfclbbkgep")
public suspend fun maxKeepAliveSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxKeepAliveSeconds = mapped
}
/**
* @param value Upper bound of Message Expiry Interval, in seconds.
*/
@JvmName("oipgvvxfndgdanij")
public suspend fun maxMessageExpirySeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxMessageExpirySeconds = mapped
}
/**
* @param value Max message size for a packet in Bytes.
*/
@JvmName("fxrhpgyfvfywptuj")
public suspend fun maxPacketSizeBytes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxPacketSizeBytes = mapped
}
/**
* @param value Upper bound of Receive Maximum that a client can request in the CONNECT packet.
*/
@JvmName("jhnhkttqucgfqlvy")
public suspend fun maxReceiveMaximum(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxReceiveMaximum = mapped
}
/**
* @param value Upper bound of Session Expiry Interval, in seconds.
*/
@JvmName("jhndbuywsgefrffw")
public suspend fun maxSessionExpirySeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxSessionExpirySeconds = mapped
}
/**
* @param value The limit on the number of queued messages for a subscriber.
*/
@JvmName("pkbjrjxayolpywys")
public suspend fun subscriberQueueLimit(`value`: SubscriberQueueLimitArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriberQueueLimit = mapped
}
/**
* @param argument The limit on the number of queued messages for a subscriber.
*/
@JvmName("gvugphaspxwjlgrn")
public suspend fun subscriberQueueLimit(argument: suspend SubscriberQueueLimitArgsBuilder.() -> Unit) {
val toBeMapped = SubscriberQueueLimitArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.subscriberQueueLimit = mapped
}
internal fun build(): ClientConfigArgs = ClientConfigArgs(
maxKeepAliveSeconds = maxKeepAliveSeconds,
maxMessageExpirySeconds = maxMessageExpirySeconds,
maxPacketSizeBytes = maxPacketSizeBytes,
maxReceiveMaximum = maxReceiveMaximum,
maxSessionExpirySeconds = maxSessionExpirySeconds,
subscriberQueueLimit = subscriberQueueLimit,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy