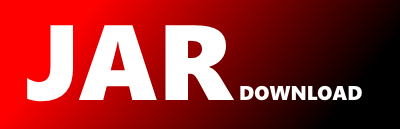
com.pulumi.azurenative.iotoperationsmq.kotlin.inputs.AutomaticCertMethodArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.iotoperationsmq.kotlin.inputs
import com.pulumi.azurenative.iotoperationsmq.inputs.AutomaticCertMethodArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Automatic TLS server certificate management with cert-manager
* @property duration Lifetime of automatically-managed certificate.
* @property issuerRef cert-manager issuerRef.
* @property privateKey Cert Manager private key.
* @property renewBefore When to begin renewing automatically-managed certificate.
* @property san Additional SANs to include in the certificate.
* @property secretName Secret for storing server certificate. Any existing data will be overwritten.
* @property secretNamespace Certificate K8S namespace. Omit to use default namespace.
*/
public data class AutomaticCertMethodArgs(
public val duration: Output? = null,
public val issuerRef: Output,
public val privateKey: Output? = null,
public val renewBefore: Output? = null,
public val san: Output? = null,
public val secretName: Output? = null,
public val secretNamespace: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.iotoperationsmq.inputs.AutomaticCertMethodArgs =
com.pulumi.azurenative.iotoperationsmq.inputs.AutomaticCertMethodArgs.builder()
.duration(duration?.applyValue({ args0 -> args0 }))
.issuerRef(issuerRef.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.privateKey(privateKey?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.renewBefore(renewBefore?.applyValue({ args0 -> args0 }))
.san(san?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secretName(secretName?.applyValue({ args0 -> args0 }))
.secretNamespace(secretNamespace?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AutomaticCertMethodArgs].
*/
@PulumiTagMarker
public class AutomaticCertMethodArgsBuilder internal constructor() {
private var duration: Output? = null
private var issuerRef: Output? = null
private var privateKey: Output? = null
private var renewBefore: Output? = null
private var san: Output? = null
private var secretName: Output? = null
private var secretNamespace: Output? = null
/**
* @param value Lifetime of automatically-managed certificate.
*/
@JvmName("vtlpladxpaiseyvf")
public suspend fun duration(`value`: Output) {
this.duration = value
}
/**
* @param value cert-manager issuerRef.
*/
@JvmName("tcwsfpentcxsrkml")
public suspend fun issuerRef(`value`: Output) {
this.issuerRef = value
}
/**
* @param value Cert Manager private key.
*/
@JvmName("guxtniyhjkihdure")
public suspend fun privateKey(`value`: Output) {
this.privateKey = value
}
/**
* @param value When to begin renewing automatically-managed certificate.
*/
@JvmName("juqsehuapunohoxo")
public suspend fun renewBefore(`value`: Output) {
this.renewBefore = value
}
/**
* @param value Additional SANs to include in the certificate.
*/
@JvmName("nyscmqruwuusklwd")
public suspend fun san(`value`: Output) {
this.san = value
}
/**
* @param value Secret for storing server certificate. Any existing data will be overwritten.
*/
@JvmName("jhfuchgwpvttlgsw")
public suspend fun secretName(`value`: Output) {
this.secretName = value
}
/**
* @param value Certificate K8S namespace. Omit to use default namespace.
*/
@JvmName("hxjxrlmioukhgroo")
public suspend fun secretNamespace(`value`: Output) {
this.secretNamespace = value
}
/**
* @param value Lifetime of automatically-managed certificate.
*/
@JvmName("smvviymltvotkhyu")
public suspend fun duration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.duration = mapped
}
/**
* @param value cert-manager issuerRef.
*/
@JvmName("morlrrghdsjstwvu")
public suspend fun issuerRef(`value`: CertManagerIssuerRefArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.issuerRef = mapped
}
/**
* @param argument cert-manager issuerRef.
*/
@JvmName("breaqagpeulnjiml")
public suspend fun issuerRef(argument: suspend CertManagerIssuerRefArgsBuilder.() -> Unit) {
val toBeMapped = CertManagerIssuerRefArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.issuerRef = mapped
}
/**
* @param value Cert Manager private key.
*/
@JvmName("hpxssoyfmiblyqmc")
public suspend fun privateKey(`value`: CertManagerPrivateKeyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateKey = mapped
}
/**
* @param argument Cert Manager private key.
*/
@JvmName("olvpjngypfijjled")
public suspend fun privateKey(argument: suspend CertManagerPrivateKeyArgsBuilder.() -> Unit) {
val toBeMapped = CertManagerPrivateKeyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.privateKey = mapped
}
/**
* @param value When to begin renewing automatically-managed certificate.
*/
@JvmName("djdgmuextqempdgg")
public suspend fun renewBefore(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.renewBefore = mapped
}
/**
* @param value Additional SANs to include in the certificate.
*/
@JvmName("hopcfdlncvobjrfb")
public suspend fun san(`value`: SanForCertArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.san = mapped
}
/**
* @param argument Additional SANs to include in the certificate.
*/
@JvmName("eoujojftebpaytdg")
public suspend fun san(argument: suspend SanForCertArgsBuilder.() -> Unit) {
val toBeMapped = SanForCertArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.san = mapped
}
/**
* @param value Secret for storing server certificate. Any existing data will be overwritten.
*/
@JvmName("ogocrjifrkfpvniq")
public suspend fun secretName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretName = mapped
}
/**
* @param value Certificate K8S namespace. Omit to use default namespace.
*/
@JvmName("rtqtkblmlvthcuub")
public suspend fun secretNamespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretNamespace = mapped
}
internal fun build(): AutomaticCertMethodArgs = AutomaticCertMethodArgs(
duration = duration,
issuerRef = issuerRef ?: throw PulumiNullFieldException("issuerRef"),
privateKey = privateKey,
renewBefore = renewBefore,
san = san,
secretName = secretName,
secretNamespace = secretNamespace,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy