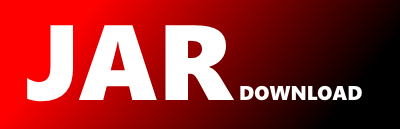
com.pulumi.azurenative.kubernetesruntime.kotlin.KubernetesruntimeFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.kubernetesruntime.kotlin
import com.pulumi.azurenative.kubernetesruntime.KubernetesruntimeFunctions.getBgpPeerPlain
import com.pulumi.azurenative.kubernetesruntime.KubernetesruntimeFunctions.getLoadBalancerPlain
import com.pulumi.azurenative.kubernetesruntime.KubernetesruntimeFunctions.getServicePlain
import com.pulumi.azurenative.kubernetesruntime.KubernetesruntimeFunctions.getStorageClassPlain
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetBgpPeerPlainArgs
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetBgpPeerPlainArgsBuilder
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetLoadBalancerPlainArgs
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetLoadBalancerPlainArgsBuilder
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetServicePlainArgs
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetServicePlainArgsBuilder
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetStorageClassPlainArgs
import com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetStorageClassPlainArgsBuilder
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetBgpPeerResult
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetLoadBalancerResult
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetServiceResult
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetStorageClassResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetBgpPeerResult.Companion.toKotlin as getBgpPeerResultToKotlin
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetLoadBalancerResult.Companion.toKotlin as getLoadBalancerResultToKotlin
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetServiceResult.Companion.toKotlin as getServiceResultToKotlin
import com.pulumi.azurenative.kubernetesruntime.kotlin.outputs.GetStorageClassResult.Companion.toKotlin as getStorageClassResultToKotlin
public object KubernetesruntimeFunctions {
/**
* Get a BgpPeer
* Azure REST API version: 2024-03-01.
* Other available API versions: 2023-10-01-preview.
* @param argument null
* @return A BgpPeer resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getBgpPeer(argument: GetBgpPeerPlainArgs): GetBgpPeerResult =
getBgpPeerResultToKotlin(getBgpPeerPlain(argument.toJava()).await())
/**
* @see [getBgpPeer].
* @param bgpPeerName The name of the BgpPeer
* @param resourceUri The fully qualified Azure Resource manager identifier of the resource.
* @return A BgpPeer resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getBgpPeer(bgpPeerName: String, resourceUri: String): GetBgpPeerResult {
val argument = GetBgpPeerPlainArgs(
bgpPeerName = bgpPeerName,
resourceUri = resourceUri,
)
return getBgpPeerResultToKotlin(getBgpPeerPlain(argument.toJava()).await())
}
/**
* @see [getBgpPeer].
* @param argument Builder for [com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetBgpPeerPlainArgs].
* @return A BgpPeer resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getBgpPeer(argument: suspend GetBgpPeerPlainArgsBuilder.() -> Unit): GetBgpPeerResult {
val builder = GetBgpPeerPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBgpPeerResultToKotlin(getBgpPeerPlain(builtArgument.toJava()).await())
}
/**
* Get a LoadBalancer
* Azure REST API version: 2024-03-01.
* Other available API versions: 2023-10-01-preview.
* @param argument null
* @return A LoadBalancer resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getLoadBalancer(argument: GetLoadBalancerPlainArgs): GetLoadBalancerResult =
getLoadBalancerResultToKotlin(getLoadBalancerPlain(argument.toJava()).await())
/**
* @see [getLoadBalancer].
* @param loadBalancerName The name of the LoadBalancer
* @param resourceUri The fully qualified Azure Resource manager identifier of the resource.
* @return A LoadBalancer resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getLoadBalancer(loadBalancerName: String, resourceUri: String): GetLoadBalancerResult {
val argument = GetLoadBalancerPlainArgs(
loadBalancerName = loadBalancerName,
resourceUri = resourceUri,
)
return getLoadBalancerResultToKotlin(getLoadBalancerPlain(argument.toJava()).await())
}
/**
* @see [getLoadBalancer].
* @param argument Builder for [com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetLoadBalancerPlainArgs].
* @return A LoadBalancer resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getLoadBalancer(argument: suspend GetLoadBalancerPlainArgsBuilder.() -> Unit): GetLoadBalancerResult {
val builder = GetLoadBalancerPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLoadBalancerResultToKotlin(getLoadBalancerPlain(builtArgument.toJava()).await())
}
/**
* Get a ServiceResource
* Azure REST API version: 2024-03-01.
* Other available API versions: 2023-10-01-preview.
* @param argument null
* @return A Service resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getService(argument: GetServicePlainArgs): GetServiceResult =
getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
/**
* @see [getService].
* @param resourceUri The fully qualified Azure Resource manager identifier of the resource.
* @param serviceName The name of the the service
* @return A Service resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getService(resourceUri: String, serviceName: String): GetServiceResult {
val argument = GetServicePlainArgs(
resourceUri = resourceUri,
serviceName = serviceName,
)
return getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
}
/**
* @see [getService].
* @param argument Builder for [com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetServicePlainArgs].
* @return A Service resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getService(argument: suspend GetServicePlainArgsBuilder.() -> Unit): GetServiceResult {
val builder = GetServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceResultToKotlin(getServicePlain(builtArgument.toJava()).await())
}
/**
* Get a StorageClassResource
* Azure REST API version: 2024-03-01.
* Other available API versions: 2023-10-01-preview.
* @param argument null
* @return A StorageClass resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getStorageClass(argument: GetStorageClassPlainArgs): GetStorageClassResult =
getStorageClassResultToKotlin(getStorageClassPlain(argument.toJava()).await())
/**
* @see [getStorageClass].
* @param resourceUri The fully qualified Azure Resource manager identifier of the resource.
* @param storageClassName The name of the the storage class
* @return A StorageClass resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getStorageClass(resourceUri: String, storageClassName: String): GetStorageClassResult {
val argument = GetStorageClassPlainArgs(
resourceUri = resourceUri,
storageClassName = storageClassName,
)
return getStorageClassResultToKotlin(getStorageClassPlain(argument.toJava()).await())
}
/**
* @see [getStorageClass].
* @param argument Builder for [com.pulumi.azurenative.kubernetesruntime.kotlin.inputs.GetStorageClassPlainArgs].
* @return A StorageClass resource for an Arc connected cluster (Microsoft.Kubernetes/connectedClusters)
*/
public suspend fun getStorageClass(argument: suspend GetStorageClassPlainArgsBuilder.() -> Unit): GetStorageClassResult {
val builder = GetStorageClassPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStorageClassResultToKotlin(getStorageClassPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy