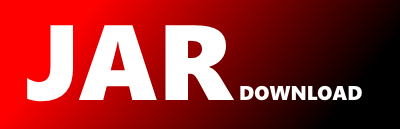
com.pulumi.azurenative.kusto.kotlin.AttachedDatabaseConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.kusto.kotlin
import com.pulumi.azurenative.kusto.kotlin.outputs.TableLevelSharingPropertiesResponse
import com.pulumi.azurenative.kusto.kotlin.outputs.TableLevelSharingPropertiesResponse.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [AttachedDatabaseConfiguration].
*/
@PulumiTagMarker
public class AttachedDatabaseConfigurationResourceBuilder internal constructor() {
public var name: String? = null
public var args: AttachedDatabaseConfigurationArgs = AttachedDatabaseConfigurationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AttachedDatabaseConfigurationArgsBuilder.() -> Unit) {
val builder = AttachedDatabaseConfigurationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AttachedDatabaseConfiguration {
val builtJavaResource =
com.pulumi.azurenative.kusto.AttachedDatabaseConfiguration(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AttachedDatabaseConfiguration(builtJavaResource)
}
}
/**
* Class representing an attached database configuration.
* Azure REST API version: 2022-12-29. Prior API version in Azure Native 1.x: 2021-01-01.
* Other available API versions: 2023-05-02, 2023-08-15.
* ## Example Usage
* ### AttachedDatabaseConfigurationsCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var attachedDatabaseConfiguration = new AzureNative.Kusto.AttachedDatabaseConfiguration("attachedDatabaseConfiguration", new()
* {
* AttachedDatabaseConfigurationName = "attachedDatabaseConfigurationsTest",
* ClusterName = "kustoCluster2",
* ClusterResourceId = "/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.Kusto/Clusters/kustoCluster2",
* DatabaseName = "kustodatabase",
* DatabaseNameOverride = "overridekustodatabase",
* DefaultPrincipalsModificationKind = AzureNative.Kusto.DefaultPrincipalsModificationKind.Union,
* Location = "westus",
* ResourceGroupName = "kustorptest",
* TableLevelSharingProperties = new AzureNative.Kusto.Inputs.TableLevelSharingPropertiesArgs
* {
* ExternalTablesToExclude = new[]
* {
* "ExternalTable2",
* },
* ExternalTablesToInclude = new[]
* {
* "ExternalTable1",
* },
* MaterializedViewsToExclude = new[]
* {
* "MaterializedViewTable2",
* },
* MaterializedViewsToInclude = new[]
* {
* "MaterializedViewTable1",
* },
* TablesToExclude = new[]
* {
* "Table2",
* },
* TablesToInclude = new[]
* {
* "Table1",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* kusto "github.com/pulumi/pulumi-azure-native-sdk/kusto/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kusto.NewAttachedDatabaseConfiguration(ctx, "attachedDatabaseConfiguration", &kusto.AttachedDatabaseConfigurationArgs{
* AttachedDatabaseConfigurationName: pulumi.String("attachedDatabaseConfigurationsTest"),
* ClusterName: pulumi.String("kustoCluster2"),
* ClusterResourceId: pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.Kusto/Clusters/kustoCluster2"),
* DatabaseName: pulumi.String("kustodatabase"),
* DatabaseNameOverride: pulumi.String("overridekustodatabase"),
* DefaultPrincipalsModificationKind: pulumi.String(kusto.DefaultPrincipalsModificationKindUnion),
* Location: pulumi.String("westus"),
* ResourceGroupName: pulumi.String("kustorptest"),
* TableLevelSharingProperties: &kusto.TableLevelSharingPropertiesArgs{
* ExternalTablesToExclude: pulumi.StringArray{
* pulumi.String("ExternalTable2"),
* },
* ExternalTablesToInclude: pulumi.StringArray{
* pulumi.String("ExternalTable1"),
* },
* MaterializedViewsToExclude: pulumi.StringArray{
* pulumi.String("MaterializedViewTable2"),
* },
* MaterializedViewsToInclude: pulumi.StringArray{
* pulumi.String("MaterializedViewTable1"),
* },
* TablesToExclude: pulumi.StringArray{
* pulumi.String("Table2"),
* },
* TablesToInclude: pulumi.StringArray{
* pulumi.String("Table1"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.AttachedDatabaseConfiguration;
* import com.pulumi.azurenative.kusto.AttachedDatabaseConfigurationArgs;
* import com.pulumi.azurenative.kusto.inputs.TableLevelSharingPropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var attachedDatabaseConfiguration = new AttachedDatabaseConfiguration("attachedDatabaseConfiguration", AttachedDatabaseConfigurationArgs.builder()
* .attachedDatabaseConfigurationName("attachedDatabaseConfigurationsTest")
* .clusterName("kustoCluster2")
* .clusterResourceId("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.Kusto/Clusters/kustoCluster2")
* .databaseName("kustodatabase")
* .databaseNameOverride("overridekustodatabase")
* .defaultPrincipalsModificationKind("Union")
* .location("westus")
* .resourceGroupName("kustorptest")
* .tableLevelSharingProperties(TableLevelSharingPropertiesArgs.builder()
* .externalTablesToExclude("ExternalTable2")
* .externalTablesToInclude("ExternalTable1")
* .materializedViewsToExclude("MaterializedViewTable2")
* .materializedViewsToInclude("MaterializedViewTable1")
* .tablesToExclude("Table2")
* .tablesToInclude("Table1")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:kusto:AttachedDatabaseConfiguration kustoCluster2/attachedDatabaseConfigurationsTest /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Kusto/clusters/{clusterName}/attachedDatabaseConfigurations/{attachedDatabaseConfigurationName}
* ```
*/
public class AttachedDatabaseConfiguration internal constructor(
override val javaResource: com.pulumi.azurenative.kusto.AttachedDatabaseConfiguration,
) : KotlinCustomResource(javaResource, AttachedDatabaseConfigurationMapper) {
/**
* The list of databases from the clusterResourceId which are currently attached to the cluster.
*/
public val attachedDatabaseNames: Output>
get() = javaResource.attachedDatabaseNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The resource id of the cluster where the databases you would like to attach reside.
*/
public val clusterResourceId: Output
get() = javaResource.clusterResourceId().applyValue({ args0 -> args0 })
/**
* The name of the database which you would like to attach, use * if you want to follow all current and future databases.
*/
public val databaseName: Output
get() = javaResource.databaseName().applyValue({ args0 -> args0 })
/**
* Overrides the original database name. Relevant only when attaching to a specific database.
*/
public val databaseNameOverride: Output?
get() = javaResource.databaseNameOverride().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Adds a prefix to the attached databases name. When following an entire cluster, that prefix would be added to all of the databases original names from leader cluster.
*/
public val databaseNamePrefix: Output?
get() = javaResource.databaseNamePrefix().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The default principals modification kind
*/
public val defaultPrincipalsModificationKind: Output
get() = javaResource.defaultPrincipalsModificationKind().applyValue({ args0 -> args0 })
/**
* Resource location.
*/
public val location: Output?
get() = javaResource.location().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The provisioned state of the resource.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* Table level sharing specifications
*/
public val tableLevelSharingProperties: Output?
get() = javaResource.tableLevelSharingProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
}).orElse(null)
})
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object AttachedDatabaseConfigurationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.kusto.AttachedDatabaseConfiguration::class == javaResource::class
override fun map(javaResource: Resource): AttachedDatabaseConfiguration =
AttachedDatabaseConfiguration(
javaResource as
com.pulumi.azurenative.kusto.AttachedDatabaseConfiguration,
)
}
/**
* @see [AttachedDatabaseConfiguration].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AttachedDatabaseConfiguration].
*/
public suspend fun attachedDatabaseConfiguration(
name: String,
block: suspend AttachedDatabaseConfigurationResourceBuilder.() -> Unit,
): AttachedDatabaseConfiguration {
val builder = AttachedDatabaseConfigurationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AttachedDatabaseConfiguration].
* @param name The _unique_ name of the resulting resource.
*/
public fun attachedDatabaseConfiguration(name: String): AttachedDatabaseConfiguration {
val builder = AttachedDatabaseConfigurationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy