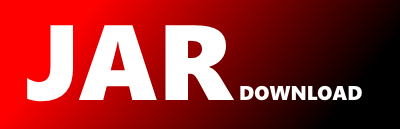
com.pulumi.azurenative.kusto.kotlin.CosmosDbDataConnectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.kusto.kotlin
import com.pulumi.azurenative.kusto.CosmosDbDataConnectionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Class representing a CosmosDb data connection.
* Azure REST API version: 2022-12-29. Prior API version in Azure Native 1.x: 2021-01-01.
* ## Example Usage
* ### KustoDataConnectionsCosmosDbCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var cosmosDbDataConnection = new AzureNative.Kusto.CosmosDbDataConnection("cosmosDbDataConnection", new()
* {
* ClusterName = "kustoCluster",
* CosmosDbAccountResourceId = "/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.DocumentDb/databaseAccounts/cosmosDbAccountTest1",
* CosmosDbContainer = "cosmosDbContainerTest",
* CosmosDbDatabase = "cosmosDbDatabaseTest",
* DataConnectionName = "dataConnectionTest",
* DatabaseName = "KustoDatabase1",
* Kind = "CosmosDb",
* Location = "westus",
* ManagedIdentityResourceId = "/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.ManagedIdentity/userAssignedIdentities/managedidentityTest1",
* MappingRuleName = "TestMapping",
* ResourceGroupName = "kustorptest",
* RetrievalStartDate = "2022-07-29T12:00:00.6554616Z",
* TableName = "TestTable",
* });
* });
* ```
* ```go
* package main
* import (
* kusto "github.com/pulumi/pulumi-azure-native-sdk/kusto/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kusto.NewCosmosDbDataConnection(ctx, "cosmosDbDataConnection", &kusto.CosmosDbDataConnectionArgs{
* ClusterName: pulumi.String("kustoCluster"),
* CosmosDbAccountResourceId: pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.DocumentDb/databaseAccounts/cosmosDbAccountTest1"),
* CosmosDbContainer: pulumi.String("cosmosDbContainerTest"),
* CosmosDbDatabase: pulumi.String("cosmosDbDatabaseTest"),
* DataConnectionName: pulumi.String("dataConnectionTest"),
* DatabaseName: pulumi.String("KustoDatabase1"),
* Kind: pulumi.String("CosmosDb"),
* Location: pulumi.String("westus"),
* ManagedIdentityResourceId: pulumi.String("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.ManagedIdentity/userAssignedIdentities/managedidentityTest1"),
* MappingRuleName: pulumi.String("TestMapping"),
* ResourceGroupName: pulumi.String("kustorptest"),
* RetrievalStartDate: pulumi.String("2022-07-29T12:00:00.6554616Z"),
* TableName: pulumi.String("TestTable"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.CosmosDbDataConnection;
* import com.pulumi.azurenative.kusto.CosmosDbDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var cosmosDbDataConnection = new CosmosDbDataConnection("cosmosDbDataConnection", CosmosDbDataConnectionArgs.builder()
* .clusterName("kustoCluster")
* .cosmosDbAccountResourceId("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.DocumentDb/databaseAccounts/cosmosDbAccountTest1")
* .cosmosDbContainer("cosmosDbContainerTest")
* .cosmosDbDatabase("cosmosDbDatabaseTest")
* .dataConnectionName("dataConnectionTest")
* .databaseName("KustoDatabase1")
* .kind("CosmosDb")
* .location("westus")
* .managedIdentityResourceId("/subscriptions/12345678-1234-1234-1234-123456789098/resourceGroups/kustorptest/providers/Microsoft.ManagedIdentity/userAssignedIdentities/managedidentityTest1")
* .mappingRuleName("TestMapping")
* .resourceGroupName("kustorptest")
* .retrievalStartDate("2022-07-29T12:00:00.6554616Z")
* .tableName("TestTable")
* .build());
* }
* }
* ```
* ### KustoDataConnectionsCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var cosmosDbDataConnection = new AzureNative.Kusto.CosmosDbDataConnection("cosmosDbDataConnection", new()
* {
* ClusterName = "kustoCluster",
* DataConnectionName = "dataConnectionTest",
* DatabaseName = "KustoDatabase8",
* ResourceGroupName = "kustorptest",
* });
* });
* ```
* ```go
* package main
* import (
* kusto "github.com/pulumi/pulumi-azure-native-sdk/kusto/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kusto.NewCosmosDbDataConnection(ctx, "cosmosDbDataConnection", &kusto.CosmosDbDataConnectionArgs{
* ClusterName: pulumi.String("kustoCluster"),
* DataConnectionName: pulumi.String("dataConnectionTest"),
* DatabaseName: pulumi.String("KustoDatabase8"),
* ResourceGroupName: pulumi.String("kustorptest"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.CosmosDbDataConnection;
* import com.pulumi.azurenative.kusto.CosmosDbDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var cosmosDbDataConnection = new CosmosDbDataConnection("cosmosDbDataConnection", CosmosDbDataConnectionArgs.builder()
* .clusterName("kustoCluster")
* .dataConnectionName("dataConnectionTest")
* .databaseName("KustoDatabase8")
* .resourceGroupName("kustorptest")
* .build());
* }
* }
* ```
* ### KustoDataConnectionsEventGridCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var cosmosDbDataConnection = new AzureNative.Kusto.CosmosDbDataConnection("cosmosDbDataConnection", new()
* {
* ClusterName = "kustoCluster",
* DataConnectionName = "dataConnectionTest",
* DatabaseName = "KustoDatabase8",
* ResourceGroupName = "kustorptest",
* });
* });
* ```
* ```go
* package main
* import (
* kusto "github.com/pulumi/pulumi-azure-native-sdk/kusto/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kusto.NewCosmosDbDataConnection(ctx, "cosmosDbDataConnection", &kusto.CosmosDbDataConnectionArgs{
* ClusterName: pulumi.String("kustoCluster"),
* DataConnectionName: pulumi.String("dataConnectionTest"),
* DatabaseName: pulumi.String("KustoDatabase8"),
* ResourceGroupName: pulumi.String("kustorptest"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.CosmosDbDataConnection;
* import com.pulumi.azurenative.kusto.CosmosDbDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var cosmosDbDataConnection = new CosmosDbDataConnection("cosmosDbDataConnection", CosmosDbDataConnectionArgs.builder()
* .clusterName("kustoCluster")
* .dataConnectionName("dataConnectionTest")
* .databaseName("KustoDatabase8")
* .resourceGroupName("kustorptest")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:kusto:CosmosDbDataConnection kustoCluster/KustoDatabase8/dataConnectionTest /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Kusto/clusters/{clusterName}/databases/{databaseName}/dataConnections/{dataConnectionName}
* ```
* @property clusterName The name of the Kusto cluster.
* @property cosmosDbAccountResourceId The resource ID of the Cosmos DB account used to create the data connection.
* @property cosmosDbContainer The name of an existing container in the Cosmos DB database.
* @property cosmosDbDatabase The name of an existing database in the Cosmos DB account.
* @property dataConnectionName The name of the data connection.
* @property databaseName The name of the database in the Kusto cluster.
* @property kind Kind of the endpoint for the data connection
* Expected value is 'CosmosDb'.
* @property location Resource location.
* @property managedIdentityResourceId The resource ID of a managed system or user-assigned identity. The identity is used to authenticate with Cosmos DB.
* @property mappingRuleName The name of an existing mapping rule to use when ingesting the retrieved data.
* @property resourceGroupName The name of the resource group containing the Kusto cluster.
* @property retrievalStartDate Optional. If defined, the data connection retrieves Cosmos DB documents created or updated after the specified retrieval start date.
* @property tableName The case-sensitive name of the existing target table in your cluster. Retrieved data is ingested into this table.
*/
public data class CosmosDbDataConnectionArgs(
public val clusterName: Output? = null,
public val cosmosDbAccountResourceId: Output? = null,
public val cosmosDbContainer: Output? = null,
public val cosmosDbDatabase: Output? = null,
public val dataConnectionName: Output? = null,
public val databaseName: Output? = null,
public val kind: Output? = null,
public val location: Output? = null,
public val managedIdentityResourceId: Output? = null,
public val mappingRuleName: Output? = null,
public val resourceGroupName: Output? = null,
public val retrievalStartDate: Output? = null,
public val tableName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.kusto.CosmosDbDataConnectionArgs =
com.pulumi.azurenative.kusto.CosmosDbDataConnectionArgs.builder()
.clusterName(clusterName?.applyValue({ args0 -> args0 }))
.cosmosDbAccountResourceId(cosmosDbAccountResourceId?.applyValue({ args0 -> args0 }))
.cosmosDbContainer(cosmosDbContainer?.applyValue({ args0 -> args0 }))
.cosmosDbDatabase(cosmosDbDatabase?.applyValue({ args0 -> args0 }))
.dataConnectionName(dataConnectionName?.applyValue({ args0 -> args0 }))
.databaseName(databaseName?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.managedIdentityResourceId(managedIdentityResourceId?.applyValue({ args0 -> args0 }))
.mappingRuleName(mappingRuleName?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.retrievalStartDate(retrievalStartDate?.applyValue({ args0 -> args0 }))
.tableName(tableName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CosmosDbDataConnectionArgs].
*/
@PulumiTagMarker
public class CosmosDbDataConnectionArgsBuilder internal constructor() {
private var clusterName: Output? = null
private var cosmosDbAccountResourceId: Output? = null
private var cosmosDbContainer: Output? = null
private var cosmosDbDatabase: Output? = null
private var dataConnectionName: Output? = null
private var databaseName: Output? = null
private var kind: Output? = null
private var location: Output? = null
private var managedIdentityResourceId: Output? = null
private var mappingRuleName: Output? = null
private var resourceGroupName: Output? = null
private var retrievalStartDate: Output? = null
private var tableName: Output? = null
/**
* @param value The name of the Kusto cluster.
*/
@JvmName("ibsdmytcykrbbjjk")
public suspend fun clusterName(`value`: Output) {
this.clusterName = value
}
/**
* @param value The resource ID of the Cosmos DB account used to create the data connection.
*/
@JvmName("eaetftdgkkqsjhki")
public suspend fun cosmosDbAccountResourceId(`value`: Output) {
this.cosmosDbAccountResourceId = value
}
/**
* @param value The name of an existing container in the Cosmos DB database.
*/
@JvmName("oulujamvkubobavs")
public suspend fun cosmosDbContainer(`value`: Output) {
this.cosmosDbContainer = value
}
/**
* @param value The name of an existing database in the Cosmos DB account.
*/
@JvmName("plmvojoaiivkcddc")
public suspend fun cosmosDbDatabase(`value`: Output) {
this.cosmosDbDatabase = value
}
/**
* @param value The name of the data connection.
*/
@JvmName("xtppsovgnurpsjlt")
public suspend fun dataConnectionName(`value`: Output) {
this.dataConnectionName = value
}
/**
* @param value The name of the database in the Kusto cluster.
*/
@JvmName("kinykhhekypefnhi")
public suspend fun databaseName(`value`: Output) {
this.databaseName = value
}
/**
* @param value Kind of the endpoint for the data connection
* Expected value is 'CosmosDb'.
*/
@JvmName("cmjrjehtbsouejqq")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Resource location.
*/
@JvmName("gafptwcnocircojp")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The resource ID of a managed system or user-assigned identity. The identity is used to authenticate with Cosmos DB.
*/
@JvmName("sbtrblqbvdgutdti")
public suspend fun managedIdentityResourceId(`value`: Output) {
this.managedIdentityResourceId = value
}
/**
* @param value The name of an existing mapping rule to use when ingesting the retrieved data.
*/
@JvmName("fkrtxstrumwqckxp")
public suspend fun mappingRuleName(`value`: Output) {
this.mappingRuleName = value
}
/**
* @param value The name of the resource group containing the Kusto cluster.
*/
@JvmName("cqxixxonxdsgjcoa")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Optional. If defined, the data connection retrieves Cosmos DB documents created or updated after the specified retrieval start date.
*/
@JvmName("snbyltmrtrtgynwv")
public suspend fun retrievalStartDate(`value`: Output) {
this.retrievalStartDate = value
}
/**
* @param value The case-sensitive name of the existing target table in your cluster. Retrieved data is ingested into this table.
*/
@JvmName("sfmvojugspbacgbi")
public suspend fun tableName(`value`: Output) {
this.tableName = value
}
/**
* @param value The name of the Kusto cluster.
*/
@JvmName("ktmatjeqtckbnjos")
public suspend fun clusterName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterName = mapped
}
/**
* @param value The resource ID of the Cosmos DB account used to create the data connection.
*/
@JvmName("cmgrxtgcmoxyncht")
public suspend fun cosmosDbAccountResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosDbAccountResourceId = mapped
}
/**
* @param value The name of an existing container in the Cosmos DB database.
*/
@JvmName("njaccjungtlkuxlq")
public suspend fun cosmosDbContainer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosDbContainer = mapped
}
/**
* @param value The name of an existing database in the Cosmos DB account.
*/
@JvmName("sckeuksflynuaefr")
public suspend fun cosmosDbDatabase(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosDbDatabase = mapped
}
/**
* @param value The name of the data connection.
*/
@JvmName("yspuyxgarmnslgld")
public suspend fun dataConnectionName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataConnectionName = mapped
}
/**
* @param value The name of the database in the Kusto cluster.
*/
@JvmName("kbmopwtwkklruvna")
public suspend fun databaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseName = mapped
}
/**
* @param value Kind of the endpoint for the data connection
* Expected value is 'CosmosDb'.
*/
@JvmName("hdlttshpdojgpaam")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Resource location.
*/
@JvmName("fibowicvtyvlhamc")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The resource ID of a managed system or user-assigned identity. The identity is used to authenticate with Cosmos DB.
*/
@JvmName("uggfwkwqfhfimhlq")
public suspend fun managedIdentityResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedIdentityResourceId = mapped
}
/**
* @param value The name of an existing mapping rule to use when ingesting the retrieved data.
*/
@JvmName("eqjfuevpmeetiylf")
public suspend fun mappingRuleName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mappingRuleName = mapped
}
/**
* @param value The name of the resource group containing the Kusto cluster.
*/
@JvmName("piufulflpyvbrruk")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Optional. If defined, the data connection retrieves Cosmos DB documents created or updated after the specified retrieval start date.
*/
@JvmName("eymcmdimulxqaehl")
public suspend fun retrievalStartDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retrievalStartDate = mapped
}
/**
* @param value The case-sensitive name of the existing target table in your cluster. Retrieved data is ingested into this table.
*/
@JvmName("fwpbyhmilmarcgjg")
public suspend fun tableName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tableName = mapped
}
internal fun build(): CosmosDbDataConnectionArgs = CosmosDbDataConnectionArgs(
clusterName = clusterName,
cosmosDbAccountResourceId = cosmosDbAccountResourceId,
cosmosDbContainer = cosmosDbContainer,
cosmosDbDatabase = cosmosDbDatabase,
dataConnectionName = dataConnectionName,
databaseName = databaseName,
kind = kind,
location = location,
managedIdentityResourceId = managedIdentityResourceId,
mappingRuleName = mappingRuleName,
resourceGroupName = resourceGroupName,
retrievalStartDate = retrievalStartDate,
tableName = tableName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy