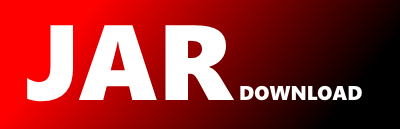
com.pulumi.azurenative.kusto.kotlin.DatabasePrincipalAssignmentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.kusto.kotlin
import com.pulumi.azurenative.kusto.DatabasePrincipalAssignmentArgs.builder
import com.pulumi.azurenative.kusto.kotlin.enums.DatabasePrincipalRole
import com.pulumi.azurenative.kusto.kotlin.enums.PrincipalType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Class representing a database principal assignment.
* Azure REST API version: 2022-12-29. Prior API version in Azure Native 1.x: 2021-01-01.
* Other available API versions: 2023-05-02, 2023-08-15.
* ## Example Usage
* ### KustoDatabasePrincipalAssignmentsCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var databasePrincipalAssignment = new AzureNative.Kusto.DatabasePrincipalAssignment("databasePrincipalAssignment", new()
* {
* ClusterName = "kustoCluster",
* DatabaseName = "Kustodatabase8",
* PrincipalAssignmentName = "kustoprincipal1",
* PrincipalId = "87654321-1234-1234-1234-123456789123",
* PrincipalType = AzureNative.Kusto.PrincipalType.App,
* ResourceGroupName = "kustorptest",
* Role = AzureNative.Kusto.DatabasePrincipalRole.Admin,
* TenantId = "12345678-1234-1234-1234-123456789123",
* });
* });
* ```
* ```go
* package main
* import (
* kusto "github.com/pulumi/pulumi-azure-native-sdk/kusto/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kusto.NewDatabasePrincipalAssignment(ctx, "databasePrincipalAssignment", &kusto.DatabasePrincipalAssignmentArgs{
* ClusterName: pulumi.String("kustoCluster"),
* DatabaseName: pulumi.String("Kustodatabase8"),
* PrincipalAssignmentName: pulumi.String("kustoprincipal1"),
* PrincipalId: pulumi.String("87654321-1234-1234-1234-123456789123"),
* PrincipalType: pulumi.String(kusto.PrincipalTypeApp),
* ResourceGroupName: pulumi.String("kustorptest"),
* Role: pulumi.String(kusto.DatabasePrincipalRoleAdmin),
* TenantId: pulumi.String("12345678-1234-1234-1234-123456789123"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kusto.DatabasePrincipalAssignment;
* import com.pulumi.azurenative.kusto.DatabasePrincipalAssignmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var databasePrincipalAssignment = new DatabasePrincipalAssignment("databasePrincipalAssignment", DatabasePrincipalAssignmentArgs.builder()
* .clusterName("kustoCluster")
* .databaseName("Kustodatabase8")
* .principalAssignmentName("kustoprincipal1")
* .principalId("87654321-1234-1234-1234-123456789123")
* .principalType("App")
* .resourceGroupName("kustorptest")
* .role("Admin")
* .tenantId("12345678-1234-1234-1234-123456789123")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:kusto:DatabasePrincipalAssignment kustoCluster/Kustodatabase8/kustoprincipal1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Kusto/clusters/{clusterName}/databases/{databaseName}/principalAssignments/{principalAssignmentName}
* ```
* @property clusterName The name of the Kusto cluster.
* @property databaseName The name of the database in the Kusto cluster.
* @property principalAssignmentName The name of the Kusto principalAssignment.
* @property principalId The principal ID assigned to the database principal. It can be a user email, application ID, or security group name.
* @property principalType Principal type.
* @property resourceGroupName The name of the resource group containing the Kusto cluster.
* @property role Database principal role.
* @property tenantId The tenant id of the principal
*/
public data class DatabasePrincipalAssignmentArgs(
public val clusterName: Output? = null,
public val databaseName: Output? = null,
public val principalAssignmentName: Output? = null,
public val principalId: Output? = null,
public val principalType: Output>? = null,
public val resourceGroupName: Output? = null,
public val role: Output>? = null,
public val tenantId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.kusto.DatabasePrincipalAssignmentArgs =
com.pulumi.azurenative.kusto.DatabasePrincipalAssignmentArgs.builder()
.clusterName(clusterName?.applyValue({ args0 -> args0 }))
.databaseName(databaseName?.applyValue({ args0 -> args0 }))
.principalAssignmentName(principalAssignmentName?.applyValue({ args0 -> args0 }))
.principalId(principalId?.applyValue({ args0 -> args0 }))
.principalType(
principalType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.role(
role?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.tenantId(tenantId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabasePrincipalAssignmentArgs].
*/
@PulumiTagMarker
public class DatabasePrincipalAssignmentArgsBuilder internal constructor() {
private var clusterName: Output? = null
private var databaseName: Output? = null
private var principalAssignmentName: Output? = null
private var principalId: Output? = null
private var principalType: Output>? = null
private var resourceGroupName: Output? = null
private var role: Output>? = null
private var tenantId: Output? = null
/**
* @param value The name of the Kusto cluster.
*/
@JvmName("fscvlodthgrueqlp")
public suspend fun clusterName(`value`: Output) {
this.clusterName = value
}
/**
* @param value The name of the database in the Kusto cluster.
*/
@JvmName("tvvpcngescmhvxpk")
public suspend fun databaseName(`value`: Output) {
this.databaseName = value
}
/**
* @param value The name of the Kusto principalAssignment.
*/
@JvmName("jmkjyovjoirlqord")
public suspend fun principalAssignmentName(`value`: Output) {
this.principalAssignmentName = value
}
/**
* @param value The principal ID assigned to the database principal. It can be a user email, application ID, or security group name.
*/
@JvmName("shrpexociimsrsuu")
public suspend fun principalId(`value`: Output) {
this.principalId = value
}
/**
* @param value Principal type.
*/
@JvmName("xjqqpaqqlxtalpce")
public suspend fun principalType(`value`: Output>) {
this.principalType = value
}
/**
* @param value The name of the resource group containing the Kusto cluster.
*/
@JvmName("tbjhojftjmnpywgj")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Database principal role.
*/
@JvmName("vmfyfomfuqwmioxa")
public suspend fun role(`value`: Output>) {
this.role = value
}
/**
* @param value The tenant id of the principal
*/
@JvmName("gdqinwkforhqoqnd")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value The name of the Kusto cluster.
*/
@JvmName("wpqwxemxntanhmfs")
public suspend fun clusterName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterName = mapped
}
/**
* @param value The name of the database in the Kusto cluster.
*/
@JvmName("ahjfqubkexmqibpy")
public suspend fun databaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseName = mapped
}
/**
* @param value The name of the Kusto principalAssignment.
*/
@JvmName("fjbsfjwagrcitlla")
public suspend fun principalAssignmentName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.principalAssignmentName = mapped
}
/**
* @param value The principal ID assigned to the database principal. It can be a user email, application ID, or security group name.
*/
@JvmName("nsdejilpcxvkqgtn")
public suspend fun principalId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.principalId = mapped
}
/**
* @param value Principal type.
*/
@JvmName("jggwjsqrimkmnaqe")
public suspend fun principalType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.principalType = mapped
}
/**
* @param value Principal type.
*/
@JvmName("cwqmcsikpqyingsw")
public fun principalType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.principalType = mapped
}
/**
* @param value Principal type.
*/
@JvmName("xnjkitkskbjwpoil")
public fun principalType(`value`: PrincipalType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.principalType = mapped
}
/**
* @param value The name of the resource group containing the Kusto cluster.
*/
@JvmName("npoodbhpspcltlmc")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Database principal role.
*/
@JvmName("xrouvmtquklcyabn")
public suspend fun role(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Database principal role.
*/
@JvmName("ldrsfiugcvposrww")
public fun role(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Database principal role.
*/
@JvmName("febktdfuuqxaltui")
public fun role(`value`: DatabasePrincipalRole) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value The tenant id of the principal
*/
@JvmName("fwhaphblrhpbbcmq")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
internal fun build(): DatabasePrincipalAssignmentArgs = DatabasePrincipalAssignmentArgs(
clusterName = clusterName,
databaseName = databaseName,
principalAssignmentName = principalAssignmentName,
principalId = principalId,
principalType = principalType,
resourceGroupName = resourceGroupName,
role = role,
tenantId = tenantId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy