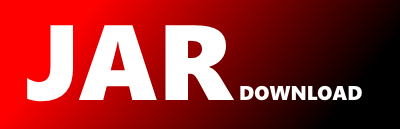
com.pulumi.azurenative.kusto.kotlin.outputs.GetClusterResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.kusto.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Class representing a Kusto cluster.
* @property acceptedAudiences The cluster's accepted audiences.
* @property allowedFqdnList List of allowed FQDNs(Fully Qualified Domain Name) for egress from Cluster.
* @property allowedIpRangeList The list of ips in the format of CIDR allowed to connect to the cluster.
* @property dataIngestionUri The cluster data ingestion URI.
* @property enableAutoStop A boolean value that indicates if the cluster could be automatically stopped (due to lack of data or no activity for many days).
* @property enableDiskEncryption A boolean value that indicates if the cluster's disks are encrypted.
* @property enableDoubleEncryption A boolean value that indicates if double encryption is enabled.
* @property enablePurge A boolean value that indicates if the purge operations are enabled.
* @property enableStreamingIngest A boolean value that indicates if the streaming ingest is enabled.
* @property engineType The engine type
* @property etag A unique read-only string that changes whenever the resource is updated.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property identity The identity of the cluster, if configured.
* @property keyVaultProperties KeyVault properties for the cluster encryption.
* @property languageExtensions List of the cluster's language extensions.
* @property location The geo-location where the resource lives
* @property name The name of the resource
* @property optimizedAutoscale Optimized auto scale definition.
* @property privateEndpointConnections A list of private endpoint connections.
* @property provisioningState The provisioned state of the resource.
* @property publicIPType Indicates what public IP type to create - IPv4 (default), or DualStack (both IPv4 and IPv6)
* @property publicNetworkAccess Public network access to the cluster is enabled by default. When disabled, only private endpoint connection to the cluster is allowed
* @property restrictOutboundNetworkAccess Whether or not to restrict outbound network access. Value is optional but if passed in, must be 'Enabled' or 'Disabled'
* @property sku The SKU of the cluster.
* @property state The state of the resource.
* @property stateReason The reason for the cluster's current state.
* @property systemData Metadata pertaining to creation and last modification of the resource.
* @property tags Resource tags.
* @property trustedExternalTenants The cluster's external tenants.
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
* @property uri The cluster URI.
* @property virtualNetworkConfiguration Virtual network definition.
* @property zones The availability zones of the cluster.
*/
public data class GetClusterResult(
public val acceptedAudiences: List? = null,
public val allowedFqdnList: List? = null,
public val allowedIpRangeList: List? = null,
public val dataIngestionUri: String,
public val enableAutoStop: Boolean? = null,
public val enableDiskEncryption: Boolean? = null,
public val enableDoubleEncryption: Boolean? = null,
public val enablePurge: Boolean? = null,
public val enableStreamingIngest: Boolean? = null,
public val engineType: String? = null,
public val etag: String,
public val id: String,
public val identity: IdentityResponse? = null,
public val keyVaultProperties: KeyVaultPropertiesResponse? = null,
public val languageExtensions: LanguageExtensionsListResponse? = null,
public val location: String,
public val name: String,
public val optimizedAutoscale: OptimizedAutoscaleResponse? = null,
public val privateEndpointConnections: List,
public val provisioningState: String,
public val publicIPType: String? = null,
public val publicNetworkAccess: String? = null,
public val restrictOutboundNetworkAccess: String? = null,
public val sku: AzureSkuResponse,
public val state: String,
public val stateReason: String,
public val systemData: SystemDataResponse,
public val tags: Map? = null,
public val trustedExternalTenants: List? = null,
public val type: String,
public val uri: String,
public val virtualNetworkConfiguration: VirtualNetworkConfigurationResponse? = null,
public val zones: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.kusto.outputs.GetClusterResult): GetClusterResult = GetClusterResult(
acceptedAudiences = javaType.acceptedAudiences().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.AcceptedAudiencesResponse.Companion.toKotlin(args0)
})
}),
allowedFqdnList = javaType.allowedFqdnList().map({ args0 -> args0 }),
allowedIpRangeList = javaType.allowedIpRangeList().map({ args0 -> args0 }),
dataIngestionUri = javaType.dataIngestionUri(),
enableAutoStop = javaType.enableAutoStop().map({ args0 -> args0 }).orElse(null),
enableDiskEncryption = javaType.enableDiskEncryption().map({ args0 -> args0 }).orElse(null),
enableDoubleEncryption = javaType.enableDoubleEncryption().map({ args0 -> args0 }).orElse(null),
enablePurge = javaType.enablePurge().map({ args0 -> args0 }).orElse(null),
enableStreamingIngest = javaType.enableStreamingIngest().map({ args0 -> args0 }).orElse(null),
engineType = javaType.engineType().map({ args0 -> args0 }).orElse(null),
etag = javaType.etag(),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.IdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
keyVaultProperties = javaType.keyVaultProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.KeyVaultPropertiesResponse.Companion.toKotlin(args0)
})
}).orElse(null),
languageExtensions = javaType.languageExtensions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.LanguageExtensionsListResponse.Companion.toKotlin(args0)
})
}).orElse(null),
location = javaType.location(),
name = javaType.name(),
optimizedAutoscale = javaType.optimizedAutoscale().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.OptimizedAutoscaleResponse.Companion.toKotlin(args0)
})
}).orElse(null),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.PrivateEndpointConnectionResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
publicIPType = javaType.publicIPType().map({ args0 -> args0 }).orElse(null),
publicNetworkAccess = javaType.publicNetworkAccess().map({ args0 -> args0 }).orElse(null),
restrictOutboundNetworkAccess = javaType.restrictOutboundNetworkAccess().map({ args0 ->
args0
}).orElse(null),
sku = javaType.sku().let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.AzureSkuResponse.Companion.toKotlin(args0)
}),
state = javaType.state(),
stateReason = javaType.stateReason(),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
trustedExternalTenants = javaType.trustedExternalTenants().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.TrustedExternalTenantResponse.Companion.toKotlin(args0)
})
}),
type = javaType.type(),
uri = javaType.uri(),
virtualNetworkConfiguration = javaType.virtualNetworkConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.kusto.kotlin.outputs.VirtualNetworkConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
zones = javaType.zones().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy