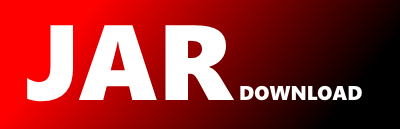
com.pulumi.azurenative.labservices.kotlin.inputs.RosterProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.labservices.kotlin.inputs
import com.pulumi.azurenative.labservices.inputs.RosterProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The lab user list management profile.
* @property activeDirectoryGroupId The AAD group ID which this lab roster is populated from. Having this set enables AAD sync mode.
* @property lmsInstance The base URI identifying the lms instance.
* @property ltiClientId The unique id of the azure lab services tool in the lms.
* @property ltiContextId The unique context identifier for the lab in the lms.
* @property ltiRosterEndpoint The uri of the names and roles service endpoint on the lms for the class attached to this lab.
*/
public data class RosterProfileArgs(
public val activeDirectoryGroupId: Output? = null,
public val lmsInstance: Output? = null,
public val ltiClientId: Output? = null,
public val ltiContextId: Output? = null,
public val ltiRosterEndpoint: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.labservices.inputs.RosterProfileArgs =
com.pulumi.azurenative.labservices.inputs.RosterProfileArgs.builder()
.activeDirectoryGroupId(activeDirectoryGroupId?.applyValue({ args0 -> args0 }))
.lmsInstance(lmsInstance?.applyValue({ args0 -> args0 }))
.ltiClientId(ltiClientId?.applyValue({ args0 -> args0 }))
.ltiContextId(ltiContextId?.applyValue({ args0 -> args0 }))
.ltiRosterEndpoint(ltiRosterEndpoint?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RosterProfileArgs].
*/
@PulumiTagMarker
public class RosterProfileArgsBuilder internal constructor() {
private var activeDirectoryGroupId: Output? = null
private var lmsInstance: Output? = null
private var ltiClientId: Output? = null
private var ltiContextId: Output? = null
private var ltiRosterEndpoint: Output? = null
/**
* @param value The AAD group ID which this lab roster is populated from. Having this set enables AAD sync mode.
*/
@JvmName("khgcqpkcbcbvqtnb")
public suspend fun activeDirectoryGroupId(`value`: Output) {
this.activeDirectoryGroupId = value
}
/**
* @param value The base URI identifying the lms instance.
*/
@JvmName("ijnnnwgnvdydqihq")
public suspend fun lmsInstance(`value`: Output) {
this.lmsInstance = value
}
/**
* @param value The unique id of the azure lab services tool in the lms.
*/
@JvmName("ikwehttxnfwvjrif")
public suspend fun ltiClientId(`value`: Output) {
this.ltiClientId = value
}
/**
* @param value The unique context identifier for the lab in the lms.
*/
@JvmName("puyttbhgysvgqyog")
public suspend fun ltiContextId(`value`: Output) {
this.ltiContextId = value
}
/**
* @param value The uri of the names and roles service endpoint on the lms for the class attached to this lab.
*/
@JvmName("eiwmskihglmbflae")
public suspend fun ltiRosterEndpoint(`value`: Output) {
this.ltiRosterEndpoint = value
}
/**
* @param value The AAD group ID which this lab roster is populated from. Having this set enables AAD sync mode.
*/
@JvmName("msajjueaiclwncma")
public suspend fun activeDirectoryGroupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.activeDirectoryGroupId = mapped
}
/**
* @param value The base URI identifying the lms instance.
*/
@JvmName("inlgrpvugnxwoxbs")
public suspend fun lmsInstance(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lmsInstance = mapped
}
/**
* @param value The unique id of the azure lab services tool in the lms.
*/
@JvmName("wrjlfcjgwxyjkwwe")
public suspend fun ltiClientId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ltiClientId = mapped
}
/**
* @param value The unique context identifier for the lab in the lms.
*/
@JvmName("mfpnikrmuujjcwtv")
public suspend fun ltiContextId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ltiContextId = mapped
}
/**
* @param value The uri of the names and roles service endpoint on the lms for the class attached to this lab.
*/
@JvmName("eblotdiyisliehmk")
public suspend fun ltiRosterEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ltiRosterEndpoint = mapped
}
internal fun build(): RosterProfileArgs = RosterProfileArgs(
activeDirectoryGroupId = activeDirectoryGroupId,
lmsInstance = lmsInstance,
ltiClientId = ltiClientId,
ltiContextId = ltiContextId,
ltiRosterEndpoint = ltiRosterEndpoint,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy