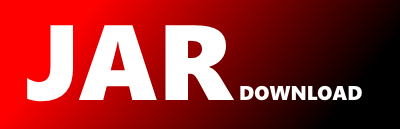
com.pulumi.azurenative.labservices.kotlin.inputs.VirtualMachineProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.labservices.kotlin.inputs
import com.pulumi.azurenative.labservices.inputs.VirtualMachineProfileArgs.builder
import com.pulumi.azurenative.labservices.kotlin.enums.CreateOption
import com.pulumi.azurenative.labservices.kotlin.enums.EnableState
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The base virtual machine configuration for a lab.
* @property additionalCapabilities Additional VM capabilities.
* @property adminUser Credentials for the admin user on the VM.
* @property createOption Indicates what lab virtual machines are created from.
* @property imageReference The image configuration for lab virtual machines.
* @property nonAdminUser Credentials for the non-admin user on the VM, if one exists.
* @property sku The SKU for the lab. Defines the type of virtual machines used in the lab.
* @property usageQuota The initial quota alloted to each lab user. Must be a time span between 0 and 9999 hours.
* @property useSharedPassword Enabling this option will use the same password for all user VMs.
*/
public data class VirtualMachineProfileArgs(
public val additionalCapabilities: Output? = null,
public val adminUser: Output,
public val createOption: Output,
public val imageReference: Output,
public val nonAdminUser: Output? = null,
public val sku: Output,
public val usageQuota: Output,
public val useSharedPassword: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.labservices.inputs.VirtualMachineProfileArgs =
com.pulumi.azurenative.labservices.inputs.VirtualMachineProfileArgs.builder()
.additionalCapabilities(
additionalCapabilities?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.adminUser(adminUser.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.createOption(createOption.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.imageReference(imageReference.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.nonAdminUser(nonAdminUser?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sku(sku.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.usageQuota(usageQuota.applyValue({ args0 -> args0 }))
.useSharedPassword(
useSharedPassword?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [VirtualMachineProfileArgs].
*/
@PulumiTagMarker
public class VirtualMachineProfileArgsBuilder internal constructor() {
private var additionalCapabilities: Output? = null
private var adminUser: Output? = null
private var createOption: Output? = null
private var imageReference: Output? = null
private var nonAdminUser: Output? = null
private var sku: Output? = null
private var usageQuota: Output? = null
private var useSharedPassword: Output? = null
/**
* @param value Additional VM capabilities.
*/
@JvmName("mlgtsguojkhmrqkp")
public suspend fun additionalCapabilities(`value`: Output) {
this.additionalCapabilities = value
}
/**
* @param value Credentials for the admin user on the VM.
*/
@JvmName("hemmxlrgviyxangb")
public suspend fun adminUser(`value`: Output) {
this.adminUser = value
}
/**
* @param value Indicates what lab virtual machines are created from.
*/
@JvmName("bukyejjjwnssqpqo")
public suspend fun createOption(`value`: Output) {
this.createOption = value
}
/**
* @param value The image configuration for lab virtual machines.
*/
@JvmName("ngdoepndlorixnic")
public suspend fun imageReference(`value`: Output) {
this.imageReference = value
}
/**
* @param value Credentials for the non-admin user on the VM, if one exists.
*/
@JvmName("lbjwjbfrgwgepyij")
public suspend fun nonAdminUser(`value`: Output) {
this.nonAdminUser = value
}
/**
* @param value The SKU for the lab. Defines the type of virtual machines used in the lab.
*/
@JvmName("acuraxtxyyxltvri")
public suspend fun sku(`value`: Output) {
this.sku = value
}
/**
* @param value The initial quota alloted to each lab user. Must be a time span between 0 and 9999 hours.
*/
@JvmName("bywcdjlgjpnrkcdq")
public suspend fun usageQuota(`value`: Output) {
this.usageQuota = value
}
/**
* @param value Enabling this option will use the same password for all user VMs.
*/
@JvmName("nixfomyhtkyqbnoh")
public suspend fun useSharedPassword(`value`: Output) {
this.useSharedPassword = value
}
/**
* @param value Additional VM capabilities.
*/
@JvmName("eslnvjfhqodwxjyq")
public suspend fun additionalCapabilities(`value`: VirtualMachineAdditionalCapabilitiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.additionalCapabilities = mapped
}
/**
* @param argument Additional VM capabilities.
*/
@JvmName("hqjigkfggssqvegs")
public suspend fun additionalCapabilities(argument: suspend VirtualMachineAdditionalCapabilitiesArgsBuilder.() -> Unit) {
val toBeMapped = VirtualMachineAdditionalCapabilitiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.additionalCapabilities = mapped
}
/**
* @param value Credentials for the admin user on the VM.
*/
@JvmName("dxgexupwfxhrfcgk")
public suspend fun adminUser(`value`: CredentialsArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.adminUser = mapped
}
/**
* @param argument Credentials for the admin user on the VM.
*/
@JvmName("kgsginntapmisjst")
public suspend fun adminUser(argument: suspend CredentialsArgsBuilder.() -> Unit) {
val toBeMapped = CredentialsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.adminUser = mapped
}
/**
* @param value Indicates what lab virtual machines are created from.
*/
@JvmName("bfsnyonotbwsonjf")
public suspend fun createOption(`value`: CreateOption) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.createOption = mapped
}
/**
* @param value The image configuration for lab virtual machines.
*/
@JvmName("mmdewmadvjavlphg")
public suspend fun imageReference(`value`: ImageReferenceArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.imageReference = mapped
}
/**
* @param argument The image configuration for lab virtual machines.
*/
@JvmName("kajfxbcnkhakqvnx")
public suspend fun imageReference(argument: suspend ImageReferenceArgsBuilder.() -> Unit) {
val toBeMapped = ImageReferenceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.imageReference = mapped
}
/**
* @param value Credentials for the non-admin user on the VM, if one exists.
*/
@JvmName("hueyxfptbusotvsj")
public suspend fun nonAdminUser(`value`: CredentialsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nonAdminUser = mapped
}
/**
* @param argument Credentials for the non-admin user on the VM, if one exists.
*/
@JvmName("wwkyemyveolwlgkf")
public suspend fun nonAdminUser(argument: suspend CredentialsArgsBuilder.() -> Unit) {
val toBeMapped = CredentialsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.nonAdminUser = mapped
}
/**
* @param value The SKU for the lab. Defines the type of virtual machines used in the lab.
*/
@JvmName("norjoteapqrgkoab")
public suspend fun sku(`value`: SkuArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sku = mapped
}
/**
* @param argument The SKU for the lab. Defines the type of virtual machines used in the lab.
*/
@JvmName("hhecekvbecvsnuut")
public suspend fun sku(argument: suspend SkuArgsBuilder.() -> Unit) {
val toBeMapped = SkuArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sku = mapped
}
/**
* @param value The initial quota alloted to each lab user. Must be a time span between 0 and 9999 hours.
*/
@JvmName("losfxdseifnksosb")
public suspend fun usageQuota(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.usageQuota = mapped
}
/**
* @param value Enabling this option will use the same password for all user VMs.
*/
@JvmName("ykpxvspcohhjfodq")
public suspend fun useSharedPassword(`value`: EnableState?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useSharedPassword = mapped
}
internal fun build(): VirtualMachineProfileArgs = VirtualMachineProfileArgs(
additionalCapabilities = additionalCapabilities,
adminUser = adminUser ?: throw PulumiNullFieldException("adminUser"),
createOption = createOption ?: throw PulumiNullFieldException("createOption"),
imageReference = imageReference ?: throw PulumiNullFieldException("imageReference"),
nonAdminUser = nonAdminUser,
sku = sku ?: throw PulumiNullFieldException("sku"),
usageQuota = usageQuota ?: throw PulumiNullFieldException("usageQuota"),
useSharedPassword = useSharedPassword,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy