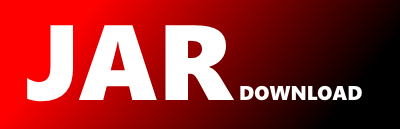
com.pulumi.azurenative.labservices.kotlin.outputs.GetLabPlanResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.labservices.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Lab Plans act as a permission container for creating labs via labs.azure.com. Additionally, they can provide a set of default configurations that will apply at the time of creating a lab, but these defaults can still be overwritten.
* @property allowedRegions The allowed regions for the lab creator to use when creating labs using this lab plan.
* @property defaultAutoShutdownProfile The default lab shutdown profile. This can be changed on a lab resource and only provides a default profile.
* @property defaultConnectionProfile The default lab connection profile. This can be changed on a lab resource and only provides a default profile.
* @property defaultNetworkProfile The lab plan network profile. To enforce lab network policies they must be defined here and cannot be changed when there are existing labs associated with this lab plan.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property identity Managed Identity Information
* @property linkedLmsInstance Base Url of the lms instance this lab plan can link lab rosters against.
* @property location The geo-location where the resource lives
* @property name The name of the resource
* @property provisioningState Current provisioning state of the lab plan.
* @property sharedGalleryId Resource ID of the Shared Image Gallery attached to this lab plan. When saving a lab template virtual machine image it will be persisted in this gallery. Shared images from the gallery can be made available to use when creating new labs.
* @property supportInfo Support contact information and instructions for users of the lab plan. This information is displayed to lab owners and virtual machine users for all labs in the lab plan.
* @property systemData Metadata pertaining to creation and last modification of the lab plan.
* @property tags Resource tags.
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public data class GetLabPlanResult(
public val allowedRegions: List? = null,
public val defaultAutoShutdownProfile: AutoShutdownProfileResponse? = null,
public val defaultConnectionProfile: ConnectionProfileResponse? = null,
public val defaultNetworkProfile: LabPlanNetworkProfileResponse? = null,
public val id: String,
public val identity: IdentityResponse? = null,
public val linkedLmsInstance: String? = null,
public val location: String,
public val name: String,
public val provisioningState: String,
public val sharedGalleryId: String? = null,
public val supportInfo: SupportInfoResponse? = null,
public val systemData: SystemDataResponse,
public val tags: Map? = null,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.labservices.outputs.GetLabPlanResult): GetLabPlanResult = GetLabPlanResult(
allowedRegions = javaType.allowedRegions().map({ args0 -> args0 }),
defaultAutoShutdownProfile = javaType.defaultAutoShutdownProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.labservices.kotlin.outputs.AutoShutdownProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
defaultConnectionProfile = javaType.defaultConnectionProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.labservices.kotlin.outputs.ConnectionProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
defaultNetworkProfile = javaType.defaultNetworkProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.labservices.kotlin.outputs.LabPlanNetworkProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.labservices.kotlin.outputs.IdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
linkedLmsInstance = javaType.linkedLmsInstance().map({ args0 -> args0 }).orElse(null),
location = javaType.location(),
name = javaType.name(),
provisioningState = javaType.provisioningState(),
sharedGalleryId = javaType.sharedGalleryId().map({ args0 -> args0 }).orElse(null),
supportInfo = javaType.supportInfo().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.labservices.kotlin.outputs.SupportInfoResponse.Companion.toKotlin(args0)
})
}).orElse(null),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.labservices.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy