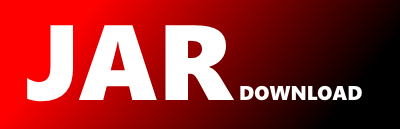
com.pulumi.azurenative.logic.kotlin.WorkflowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin
import com.pulumi.azurenative.logic.WorkflowArgs.builder
import com.pulumi.azurenative.logic.kotlin.enums.WorkflowState
import com.pulumi.azurenative.logic.kotlin.inputs.FlowAccessControlConfigurationArgs
import com.pulumi.azurenative.logic.kotlin.inputs.FlowAccessControlConfigurationArgsBuilder
import com.pulumi.azurenative.logic.kotlin.inputs.FlowEndpointsConfigurationArgs
import com.pulumi.azurenative.logic.kotlin.inputs.FlowEndpointsConfigurationArgsBuilder
import com.pulumi.azurenative.logic.kotlin.inputs.ManagedServiceIdentityArgs
import com.pulumi.azurenative.logic.kotlin.inputs.ManagedServiceIdentityArgsBuilder
import com.pulumi.azurenative.logic.kotlin.inputs.ResourceReferenceArgs
import com.pulumi.azurenative.logic.kotlin.inputs.ResourceReferenceArgsBuilder
import com.pulumi.azurenative.logic.kotlin.inputs.WorkflowParameterArgs
import com.pulumi.azurenative.logic.kotlin.inputs.WorkflowParameterArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The workflow type.
* Azure REST API version: 2019-05-01. Prior API version in Azure Native 1.x: 2019-05-01.
* Other available API versions: 2015-02-01-preview, 2016-06-01, 2018-07-01-preview.
* ## Example Usage
* ### Create or update a workflow
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var workflow = new AzureNative.Logic.Workflow("workflow", new()
* {
* Definition = new Dictionary
* {
* ["$schema"] = "https://schema.management.azure.com/providers/Microsoft.Logic/schemas/2016-06-01/workflowdefinition.json#",
* ["actions"] = new Dictionary
* {
* ["Find_pet_by_ID"] = new Dictionary
* {
* ["inputs"] = new Dictionary
* {
* ["host"] = new Dictionary
* {
* ["connection"] = new Dictionary
* {
* ["name"] = "@parameters('$connections')['test-custom-connector']['connectionId']",
* },
* },
* ["method"] = "get",
* ["path"] = "/pet/@{encodeURIComponent('1')}",
* },
* ["runAfter"] = new Dictionary
* {
* },
* ["type"] = "ApiConnection",
* },
* },
* ["contentVersion"] = "1.0.0.0",
* ["outputs"] = new Dictionary
* {
* },
* ["parameters"] = new Dictionary
* {
* ["$connections"] = new Dictionary
* {
* ["defaultValue"] = new Dictionary
* {
* },
* ["type"] = "Object",
* },
* },
* ["triggers"] = new Dictionary
* {
* ["manual"] = new Dictionary
* {
* ["inputs"] = new Dictionary
* {
* ["schema"] = new Dictionary
* {
* },
* },
* ["kind"] = "Http",
* ["type"] = "Request",
* },
* },
* },
* IntegrationAccount = new AzureNative.Logic.Inputs.ResourceReferenceArgs
* {
* Id = "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Logic/integrationAccounts/test-integration-account",
* },
* Location = "brazilsouth",
* Parameters =
* {
* { "$connections", new AzureNative.Logic.Inputs.WorkflowParameterArgs
* {
* Value = new Dictionary
* {
* ["test-custom-connector"] = new Dictionary
* {
* ["connectionId"] = "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Web/connections/test-custom-connector",
* ["connectionName"] = "test-custom-connector",
* ["id"] = "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/providers/Microsoft.Web/locations/brazilsouth/managedApis/test-custom-connector",
* },
* },
* } },
* },
* ResourceGroupName = "test-resource-group",
* Tags = null,
* WorkflowName = "test-workflow",
* });
* });
* ```
* ```go
* package main
* import (
* logic "github.com/pulumi/pulumi-azure-native-sdk/logic/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := logic.NewWorkflow(ctx, "workflow", &logic.WorkflowArgs{
* Definition: pulumi.Any(map[string]interface{}{
* "$schema": "https://schema.management.azure.com/providers/Microsoft.Logic/schemas/2016-06-01/workflowdefinition.json#",
* "actions": map[string]interface{}{
* "Find_pet_by_ID": map[string]interface{}{
* "inputs": map[string]interface{}{
* "host": map[string]interface{}{
* "connection": map[string]interface{}{
* "name": "@parameters('$connections')['test-custom-connector']['connectionId']",
* },
* },
* "method": "get",
* "path": "/pet/@{encodeURIComponent('1')}",
* },
* "runAfter": nil,
* "type": "ApiConnection",
* },
* },
* "contentVersion": "1.0.0.0",
* "outputs": nil,
* "parameters": map[string]interface{}{
* "$connections": map[string]interface{}{
* "defaultValue": nil,
* "type": "Object",
* },
* },
* "triggers": map[string]interface{}{
* "manual": map[string]interface{}{
* "inputs": map[string]interface{}{
* "schema": nil,
* },
* "kind": "Http",
* "type": "Request",
* },
* },
* }),
* IntegrationAccount: &logic.ResourceReferenceArgs{
* Id: pulumi.String("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Logic/integrationAccounts/test-integration-account"),
* },
* Location: pulumi.String("brazilsouth"),
* Parameters: logic.WorkflowParameterMap{
* "$connections": &logic.WorkflowParameterArgs{
* Value: pulumi.Any(map[string]interface{}{
* "test-custom-connector": map[string]interface{}{
* "connectionId": "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Web/connections/test-custom-connector",
* "connectionName": "test-custom-connector",
* "id": "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/providers/Microsoft.Web/locations/brazilsouth/managedApis/test-custom-connector",
* },
* }),
* },
* },
* ResourceGroupName: pulumi.String("test-resource-group"),
* Tags: nil,
* WorkflowName: pulumi.String("test-workflow"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.logic.Workflow;
* import com.pulumi.azurenative.logic.WorkflowArgs;
* import com.pulumi.azurenative.logic.inputs.ResourceReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var workflow = new Workflow("workflow", WorkflowArgs.builder()
* .definition(Map.ofEntries(
* Map.entry("$schema", "https://schema.management.azure.com/providers/Microsoft.Logic/schemas/2016-06-01/workflowdefinition.json#"),
* Map.entry("actions", Map.of("Find_pet_by_ID", Map.ofEntries(
* Map.entry("inputs", Map.ofEntries(
* Map.entry("host", Map.of("connection", Map.of("name", "@parameters('$connections')['test-custom-connector']['connectionId']"))),
* Map.entry("method", "get"),
* Map.entry("path", "/pet/@{encodeURIComponent('1')}")
* )),
* Map.entry("runAfter", ),
* Map.entry("type", "ApiConnection")
* ))),
* Map.entry("contentVersion", "1.0.0.0"),
* Map.entry("outputs", ),
* Map.entry("parameters", Map.of("$connections", Map.ofEntries(
* Map.entry("defaultValue", ),
* Map.entry("type", "Object")
* ))),
* Map.entry("triggers", Map.of("manual", Map.ofEntries(
* Map.entry("inputs", Map.of("schema", )),
* Map.entry("kind", "Http"),
* Map.entry("type", "Request")
* )))
* ))
* .integrationAccount(ResourceReferenceArgs.builder()
* .id("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Logic/integrationAccounts/test-integration-account")
* .build())
* .location("brazilsouth")
* .parameters(Map.of("$connections", Map.of("value", Map.of("test-custom-connector", Map.ofEntries(
* Map.entry("connectionId", "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Web/connections/test-custom-connector"),
* Map.entry("connectionName", "test-custom-connector"),
* Map.entry("id", "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/providers/Microsoft.Web/locations/brazilsouth/managedApis/test-custom-connector")
* )))))
* .resourceGroupName("test-resource-group")
* .tags()
* .workflowName("test-workflow")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:logic:Workflow myresource1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Logic/workflows/{workflowName}
* ```
* @property accessControl The access control configuration.
* @property definition The definition.
* @property endpointsConfiguration The endpoints configuration.
* @property identity Managed service identity properties.
* @property integrationAccount The integration account.
* @property integrationServiceEnvironment The integration service environment.
* @property location The resource location.
* @property parameters The parameters.
* @property resourceGroupName The resource group name.
* @property state The state.
* @property tags The resource tags.
* @property workflowName The workflow name.
*/
public data class WorkflowArgs(
public val accessControl: Output? = null,
public val definition: Output? = null,
public val endpointsConfiguration: Output? = null,
public val identity: Output? = null,
public val integrationAccount: Output? = null,
public val integrationServiceEnvironment: Output? = null,
public val location: Output? = null,
public val parameters: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy