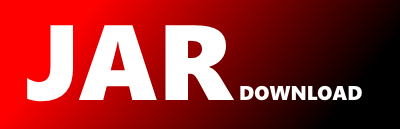
com.pulumi.azurenative.logic.kotlin.inputs.AS2SecuritySettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin.inputs
import com.pulumi.azurenative.logic.inputs.AS2SecuritySettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The AS2 agreement security settings.
* @property enableNRRForInboundDecodedMessages The value indicating whether to enable NRR for inbound decoded messages.
* @property enableNRRForInboundEncodedMessages The value indicating whether to enable NRR for inbound encoded messages.
* @property enableNRRForInboundMDN The value indicating whether to enable NRR for inbound MDN.
* @property enableNRRForOutboundDecodedMessages The value indicating whether to enable NRR for outbound decoded messages.
* @property enableNRRForOutboundEncodedMessages The value indicating whether to enable NRR for outbound encoded messages.
* @property enableNRRForOutboundMDN The value indicating whether to enable NRR for outbound MDN.
* @property encryptionCertificateName The name of the encryption certificate.
* @property overrideGroupSigningCertificate The value indicating whether to send or request a MDN.
* @property sha2AlgorithmFormat The Sha2 algorithm format. Valid values are Sha2, ShaHashSize, ShaHyphenHashSize, Sha2UnderscoreHashSize.
* @property signingCertificateName The name of the signing certificate.
*/
public data class AS2SecuritySettingsArgs(
public val enableNRRForInboundDecodedMessages: Output,
public val enableNRRForInboundEncodedMessages: Output,
public val enableNRRForInboundMDN: Output,
public val enableNRRForOutboundDecodedMessages: Output,
public val enableNRRForOutboundEncodedMessages: Output,
public val enableNRRForOutboundMDN: Output,
public val encryptionCertificateName: Output? = null,
public val overrideGroupSigningCertificate: Output,
public val sha2AlgorithmFormat: Output? = null,
public val signingCertificateName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logic.inputs.AS2SecuritySettingsArgs =
com.pulumi.azurenative.logic.inputs.AS2SecuritySettingsArgs.builder()
.enableNRRForInboundDecodedMessages(
enableNRRForInboundDecodedMessages.applyValue({ args0 ->
args0
}),
)
.enableNRRForInboundEncodedMessages(
enableNRRForInboundEncodedMessages.applyValue({ args0 ->
args0
}),
)
.enableNRRForInboundMDN(enableNRRForInboundMDN.applyValue({ args0 -> args0 }))
.enableNRRForOutboundDecodedMessages(
enableNRRForOutboundDecodedMessages.applyValue({ args0 ->
args0
}),
)
.enableNRRForOutboundEncodedMessages(
enableNRRForOutboundEncodedMessages.applyValue({ args0 ->
args0
}),
)
.enableNRRForOutboundMDN(enableNRRForOutboundMDN.applyValue({ args0 -> args0 }))
.encryptionCertificateName(encryptionCertificateName?.applyValue({ args0 -> args0 }))
.overrideGroupSigningCertificate(overrideGroupSigningCertificate.applyValue({ args0 -> args0 }))
.sha2AlgorithmFormat(sha2AlgorithmFormat?.applyValue({ args0 -> args0 }))
.signingCertificateName(signingCertificateName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AS2SecuritySettingsArgs].
*/
@PulumiTagMarker
public class AS2SecuritySettingsArgsBuilder internal constructor() {
private var enableNRRForInboundDecodedMessages: Output? = null
private var enableNRRForInboundEncodedMessages: Output? = null
private var enableNRRForInboundMDN: Output? = null
private var enableNRRForOutboundDecodedMessages: Output? = null
private var enableNRRForOutboundEncodedMessages: Output? = null
private var enableNRRForOutboundMDN: Output? = null
private var encryptionCertificateName: Output? = null
private var overrideGroupSigningCertificate: Output? = null
private var sha2AlgorithmFormat: Output? = null
private var signingCertificateName: Output? = null
/**
* @param value The value indicating whether to enable NRR for inbound decoded messages.
*/
@JvmName("yajkkuajjdroirej")
public suspend fun enableNRRForInboundDecodedMessages(`value`: Output) {
this.enableNRRForInboundDecodedMessages = value
}
/**
* @param value The value indicating whether to enable NRR for inbound encoded messages.
*/
@JvmName("mywhmlrchaavyhwm")
public suspend fun enableNRRForInboundEncodedMessages(`value`: Output) {
this.enableNRRForInboundEncodedMessages = value
}
/**
* @param value The value indicating whether to enable NRR for inbound MDN.
*/
@JvmName("ymasqncckyxbispq")
public suspend fun enableNRRForInboundMDN(`value`: Output) {
this.enableNRRForInboundMDN = value
}
/**
* @param value The value indicating whether to enable NRR for outbound decoded messages.
*/
@JvmName("ypumvknvgpgkgkes")
public suspend fun enableNRRForOutboundDecodedMessages(`value`: Output) {
this.enableNRRForOutboundDecodedMessages = value
}
/**
* @param value The value indicating whether to enable NRR for outbound encoded messages.
*/
@JvmName("phxhruejcdfcpodu")
public suspend fun enableNRRForOutboundEncodedMessages(`value`: Output) {
this.enableNRRForOutboundEncodedMessages = value
}
/**
* @param value The value indicating whether to enable NRR for outbound MDN.
*/
@JvmName("mikvblxxwkixlykb")
public suspend fun enableNRRForOutboundMDN(`value`: Output) {
this.enableNRRForOutboundMDN = value
}
/**
* @param value The name of the encryption certificate.
*/
@JvmName("hjfauyohmwpgchut")
public suspend fun encryptionCertificateName(`value`: Output) {
this.encryptionCertificateName = value
}
/**
* @param value The value indicating whether to send or request a MDN.
*/
@JvmName("mcvcsrsceppmjwah")
public suspend fun overrideGroupSigningCertificate(`value`: Output) {
this.overrideGroupSigningCertificate = value
}
/**
* @param value The Sha2 algorithm format. Valid values are Sha2, ShaHashSize, ShaHyphenHashSize, Sha2UnderscoreHashSize.
*/
@JvmName("bmaemxddeyhvkdxm")
public suspend fun sha2AlgorithmFormat(`value`: Output) {
this.sha2AlgorithmFormat = value
}
/**
* @param value The name of the signing certificate.
*/
@JvmName("jypbylvpambwurna")
public suspend fun signingCertificateName(`value`: Output) {
this.signingCertificateName = value
}
/**
* @param value The value indicating whether to enable NRR for inbound decoded messages.
*/
@JvmName("xipcybvrkyxcwadw")
public suspend fun enableNRRForInboundDecodedMessages(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enableNRRForInboundDecodedMessages = mapped
}
/**
* @param value The value indicating whether to enable NRR for inbound encoded messages.
*/
@JvmName("sxrrfbluvxvoajfb")
public suspend fun enableNRRForInboundEncodedMessages(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enableNRRForInboundEncodedMessages = mapped
}
/**
* @param value The value indicating whether to enable NRR for inbound MDN.
*/
@JvmName("xaheubbkugaxqmpu")
public suspend fun enableNRRForInboundMDN(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enableNRRForInboundMDN = mapped
}
/**
* @param value The value indicating whether to enable NRR for outbound decoded messages.
*/
@JvmName("xxmkgpjnxbwkokec")
public suspend fun enableNRRForOutboundDecodedMessages(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enableNRRForOutboundDecodedMessages = mapped
}
/**
* @param value The value indicating whether to enable NRR for outbound encoded messages.
*/
@JvmName("srqpisjpmpnkybgo")
public suspend fun enableNRRForOutboundEncodedMessages(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enableNRRForOutboundEncodedMessages = mapped
}
/**
* @param value The value indicating whether to enable NRR for outbound MDN.
*/
@JvmName("wdexqyllwgpeocuk")
public suspend fun enableNRRForOutboundMDN(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enableNRRForOutboundMDN = mapped
}
/**
* @param value The name of the encryption certificate.
*/
@JvmName("vccqvqqbprsmgmnu")
public suspend fun encryptionCertificateName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionCertificateName = mapped
}
/**
* @param value The value indicating whether to send or request a MDN.
*/
@JvmName("yonijjophaaqkupu")
public suspend fun overrideGroupSigningCertificate(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.overrideGroupSigningCertificate = mapped
}
/**
* @param value The Sha2 algorithm format. Valid values are Sha2, ShaHashSize, ShaHyphenHashSize, Sha2UnderscoreHashSize.
*/
@JvmName("yvajeofrspphrudc")
public suspend fun sha2AlgorithmFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sha2AlgorithmFormat = mapped
}
/**
* @param value The name of the signing certificate.
*/
@JvmName("fukxviwklfeenoti")
public suspend fun signingCertificateName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.signingCertificateName = mapped
}
internal fun build(): AS2SecuritySettingsArgs = AS2SecuritySettingsArgs(
enableNRRForInboundDecodedMessages = enableNRRForInboundDecodedMessages ?: throw
PulumiNullFieldException("enableNRRForInboundDecodedMessages"),
enableNRRForInboundEncodedMessages = enableNRRForInboundEncodedMessages ?: throw
PulumiNullFieldException("enableNRRForInboundEncodedMessages"),
enableNRRForInboundMDN = enableNRRForInboundMDN ?: throw
PulumiNullFieldException("enableNRRForInboundMDN"),
enableNRRForOutboundDecodedMessages = enableNRRForOutboundDecodedMessages ?: throw
PulumiNullFieldException("enableNRRForOutboundDecodedMessages"),
enableNRRForOutboundEncodedMessages = enableNRRForOutboundEncodedMessages ?: throw
PulumiNullFieldException("enableNRRForOutboundEncodedMessages"),
enableNRRForOutboundMDN = enableNRRForOutboundMDN ?: throw
PulumiNullFieldException("enableNRRForOutboundMDN"),
encryptionCertificateName = encryptionCertificateName,
overrideGroupSigningCertificate = overrideGroupSigningCertificate ?: throw
PulumiNullFieldException("overrideGroupSigningCertificate"),
sha2AlgorithmFormat = sha2AlgorithmFormat,
signingCertificateName = signingCertificateName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy