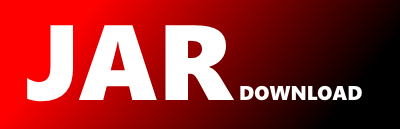
com.pulumi.azurenative.logic.kotlin.inputs.AS2ValidationSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin.inputs
import com.pulumi.azurenative.logic.inputs.AS2ValidationSettingsArgs.builder
import com.pulumi.azurenative.logic.kotlin.enums.EncryptionAlgorithm
import com.pulumi.azurenative.logic.kotlin.enums.SigningAlgorithm
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The AS2 agreement validation settings.
* @property checkCertificateRevocationListOnReceive The value indicating whether to check for certificate revocation list on receive.
* @property checkCertificateRevocationListOnSend The value indicating whether to check for certificate revocation list on send.
* @property checkDuplicateMessage The value indicating whether to check for duplicate message.
* @property compressMessage The value indicating whether the message has to be compressed.
* @property encryptMessage The value indicating whether the message has to be encrypted.
* @property encryptionAlgorithm The encryption algorithm.
* @property interchangeDuplicatesValidityDays The number of days to look back for duplicate interchange.
* @property overrideMessageProperties The value indicating whether to override incoming message properties with those in agreement.
* @property signMessage The value indicating whether the message has to be signed.
* @property signingAlgorithm The signing algorithm.
*/
public data class AS2ValidationSettingsArgs(
public val checkCertificateRevocationListOnReceive: Output,
public val checkCertificateRevocationListOnSend: Output,
public val checkDuplicateMessage: Output,
public val compressMessage: Output,
public val encryptMessage: Output,
public val encryptionAlgorithm: Output>,
public val interchangeDuplicatesValidityDays: Output,
public val overrideMessageProperties: Output,
public val signMessage: Output,
public val signingAlgorithm: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logic.inputs.AS2ValidationSettingsArgs =
com.pulumi.azurenative.logic.inputs.AS2ValidationSettingsArgs.builder()
.checkCertificateRevocationListOnReceive(
checkCertificateRevocationListOnReceive.applyValue({ args0 ->
args0
}),
)
.checkCertificateRevocationListOnSend(
checkCertificateRevocationListOnSend.applyValue({ args0 ->
args0
}),
)
.checkDuplicateMessage(checkDuplicateMessage.applyValue({ args0 -> args0 }))
.compressMessage(compressMessage.applyValue({ args0 -> args0 }))
.encryptMessage(encryptMessage.applyValue({ args0 -> args0 }))
.encryptionAlgorithm(
encryptionAlgorithm.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.interchangeDuplicatesValidityDays(interchangeDuplicatesValidityDays.applyValue({ args0 -> args0 }))
.overrideMessageProperties(overrideMessageProperties.applyValue({ args0 -> args0 }))
.signMessage(signMessage.applyValue({ args0 -> args0 }))
.signingAlgorithm(
signingAlgorithm?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
).build()
}
/**
* Builder for [AS2ValidationSettingsArgs].
*/
@PulumiTagMarker
public class AS2ValidationSettingsArgsBuilder internal constructor() {
private var checkCertificateRevocationListOnReceive: Output? = null
private var checkCertificateRevocationListOnSend: Output? = null
private var checkDuplicateMessage: Output? = null
private var compressMessage: Output? = null
private var encryptMessage: Output? = null
private var encryptionAlgorithm: Output>? = null
private var interchangeDuplicatesValidityDays: Output? = null
private var overrideMessageProperties: Output? = null
private var signMessage: Output? = null
private var signingAlgorithm: Output>? = null
/**
* @param value The value indicating whether to check for certificate revocation list on receive.
*/
@JvmName("btvkteuslanrsjlp")
public suspend fun checkCertificateRevocationListOnReceive(`value`: Output) {
this.checkCertificateRevocationListOnReceive = value
}
/**
* @param value The value indicating whether to check for certificate revocation list on send.
*/
@JvmName("ardhyjiijebtciul")
public suspend fun checkCertificateRevocationListOnSend(`value`: Output) {
this.checkCertificateRevocationListOnSend = value
}
/**
* @param value The value indicating whether to check for duplicate message.
*/
@JvmName("xcqtmqtqpjdgnbtm")
public suspend fun checkDuplicateMessage(`value`: Output) {
this.checkDuplicateMessage = value
}
/**
* @param value The value indicating whether the message has to be compressed.
*/
@JvmName("mpcasxxytmteddkm")
public suspend fun compressMessage(`value`: Output) {
this.compressMessage = value
}
/**
* @param value The value indicating whether the message has to be encrypted.
*/
@JvmName("twnpkyrtwxicimak")
public suspend fun encryptMessage(`value`: Output) {
this.encryptMessage = value
}
/**
* @param value The encryption algorithm.
*/
@JvmName("cjwqokfxfgyaebkg")
public suspend fun encryptionAlgorithm(`value`: Output>) {
this.encryptionAlgorithm = value
}
/**
* @param value The number of days to look back for duplicate interchange.
*/
@JvmName("gsdmjvfapwgvdlyv")
public suspend fun interchangeDuplicatesValidityDays(`value`: Output) {
this.interchangeDuplicatesValidityDays = value
}
/**
* @param value The value indicating whether to override incoming message properties with those in agreement.
*/
@JvmName("uxilhytxyoxkvakg")
public suspend fun overrideMessageProperties(`value`: Output) {
this.overrideMessageProperties = value
}
/**
* @param value The value indicating whether the message has to be signed.
*/
@JvmName("irtvncajlykuuqcg")
public suspend fun signMessage(`value`: Output) {
this.signMessage = value
}
/**
* @param value The signing algorithm.
*/
@JvmName("eeuuckiyddkqohds")
public suspend fun signingAlgorithm(`value`: Output>) {
this.signingAlgorithm = value
}
/**
* @param value The value indicating whether to check for certificate revocation list on receive.
*/
@JvmName("vffhmiegmsnamxgx")
public suspend fun checkCertificateRevocationListOnReceive(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.checkCertificateRevocationListOnReceive = mapped
}
/**
* @param value The value indicating whether to check for certificate revocation list on send.
*/
@JvmName("oouuxtacicevtihc")
public suspend fun checkCertificateRevocationListOnSend(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.checkCertificateRevocationListOnSend = mapped
}
/**
* @param value The value indicating whether to check for duplicate message.
*/
@JvmName("ndleivhlmpnemqyp")
public suspend fun checkDuplicateMessage(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.checkDuplicateMessage = mapped
}
/**
* @param value The value indicating whether the message has to be compressed.
*/
@JvmName("pjwxbqrfokoggbkb")
public suspend fun compressMessage(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.compressMessage = mapped
}
/**
* @param value The value indicating whether the message has to be encrypted.
*/
@JvmName("wihhighmocskyvix")
public suspend fun encryptMessage(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.encryptMessage = mapped
}
/**
* @param value The encryption algorithm.
*/
@JvmName("vqqrhvykpiteceyt")
public suspend fun encryptionAlgorithm(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.encryptionAlgorithm = mapped
}
/**
* @param value The encryption algorithm.
*/
@JvmName("pucqtpsufhdytfpa")
public fun encryptionAlgorithm(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.encryptionAlgorithm = mapped
}
/**
* @param value The encryption algorithm.
*/
@JvmName("pdbctwqcgiaulyhm")
public fun encryptionAlgorithm(`value`: EncryptionAlgorithm) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.encryptionAlgorithm = mapped
}
/**
* @param value The number of days to look back for duplicate interchange.
*/
@JvmName("qeaokejrvkdeaghv")
public suspend fun interchangeDuplicatesValidityDays(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.interchangeDuplicatesValidityDays = mapped
}
/**
* @param value The value indicating whether to override incoming message properties with those in agreement.
*/
@JvmName("eyqdxykavctblhtr")
public suspend fun overrideMessageProperties(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.overrideMessageProperties = mapped
}
/**
* @param value The value indicating whether the message has to be signed.
*/
@JvmName("sexpnashumjmqwru")
public suspend fun signMessage(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.signMessage = mapped
}
/**
* @param value The signing algorithm.
*/
@JvmName("agajsujuddgyomls")
public suspend fun signingAlgorithm(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.signingAlgorithm = mapped
}
/**
* @param value The signing algorithm.
*/
@JvmName("tvwmlcosecilgmts")
public fun signingAlgorithm(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.signingAlgorithm = mapped
}
/**
* @param value The signing algorithm.
*/
@JvmName("ckgpwfrooypsyrox")
public fun signingAlgorithm(`value`: SigningAlgorithm) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.signingAlgorithm = mapped
}
internal fun build(): AS2ValidationSettingsArgs = AS2ValidationSettingsArgs(
checkCertificateRevocationListOnReceive = checkCertificateRevocationListOnReceive ?: throw
PulumiNullFieldException("checkCertificateRevocationListOnReceive"),
checkCertificateRevocationListOnSend = checkCertificateRevocationListOnSend ?: throw
PulumiNullFieldException("checkCertificateRevocationListOnSend"),
checkDuplicateMessage = checkDuplicateMessage ?: throw
PulumiNullFieldException("checkDuplicateMessage"),
compressMessage = compressMessage ?: throw PulumiNullFieldException("compressMessage"),
encryptMessage = encryptMessage ?: throw PulumiNullFieldException("encryptMessage"),
encryptionAlgorithm = encryptionAlgorithm ?: throw PulumiNullFieldException("encryptionAlgorithm"),
interchangeDuplicatesValidityDays = interchangeDuplicatesValidityDays ?: throw
PulumiNullFieldException("interchangeDuplicatesValidityDays"),
overrideMessageProperties = overrideMessageProperties ?: throw
PulumiNullFieldException("overrideMessageProperties"),
signMessage = signMessage ?: throw PulumiNullFieldException("signMessage"),
signingAlgorithm = signingAlgorithm,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy