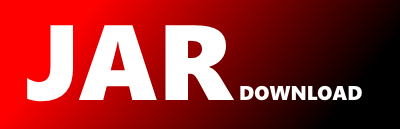
com.pulumi.azurenative.logic.kotlin.inputs.AssemblyPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin.inputs
import com.pulumi.azurenative.logic.inputs.AssemblyPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The assembly properties definition.
* @property assemblyCulture The assembly culture.
* @property assemblyName The assembly name.
* @property assemblyPublicKeyToken The assembly public key token.
* @property assemblyVersion The assembly version.
* @property changedTime The artifact changed time.
* @property content
* @property contentLink The content link.
* @property contentType The content type.
* @property createdTime The artifact creation time.
* @property metadata
*/
public data class AssemblyPropertiesArgs(
public val assemblyCulture: Output? = null,
public val assemblyName: Output,
public val assemblyPublicKeyToken: Output? = null,
public val assemblyVersion: Output? = null,
public val changedTime: Output? = null,
public val content: Output? = null,
public val contentLink: Output? = null,
public val contentType: Output? = null,
public val createdTime: Output? = null,
public val metadata: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logic.inputs.AssemblyPropertiesArgs =
com.pulumi.azurenative.logic.inputs.AssemblyPropertiesArgs.builder()
.assemblyCulture(assemblyCulture?.applyValue({ args0 -> args0 }))
.assemblyName(assemblyName.applyValue({ args0 -> args0 }))
.assemblyPublicKeyToken(assemblyPublicKeyToken?.applyValue({ args0 -> args0 }))
.assemblyVersion(assemblyVersion?.applyValue({ args0 -> args0 }))
.changedTime(changedTime?.applyValue({ args0 -> args0 }))
.content(content?.applyValue({ args0 -> args0 }))
.contentLink(contentLink?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.contentType(contentType?.applyValue({ args0 -> args0 }))
.createdTime(createdTime?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AssemblyPropertiesArgs].
*/
@PulumiTagMarker
public class AssemblyPropertiesArgsBuilder internal constructor() {
private var assemblyCulture: Output? = null
private var assemblyName: Output? = null
private var assemblyPublicKeyToken: Output? = null
private var assemblyVersion: Output? = null
private var changedTime: Output? = null
private var content: Output? = null
private var contentLink: Output? = null
private var contentType: Output? = null
private var createdTime: Output? = null
private var metadata: Output? = null
/**
* @param value The assembly culture.
*/
@JvmName("vijhphsegmevtxny")
public suspend fun assemblyCulture(`value`: Output) {
this.assemblyCulture = value
}
/**
* @param value The assembly name.
*/
@JvmName("tcxrsivatpyweepn")
public suspend fun assemblyName(`value`: Output) {
this.assemblyName = value
}
/**
* @param value The assembly public key token.
*/
@JvmName("xcdwqtustjmnjpeb")
public suspend fun assemblyPublicKeyToken(`value`: Output) {
this.assemblyPublicKeyToken = value
}
/**
* @param value The assembly version.
*/
@JvmName("uwkronqfshovvscb")
public suspend fun assemblyVersion(`value`: Output) {
this.assemblyVersion = value
}
/**
* @param value The artifact changed time.
*/
@JvmName("sfpjxqvjusubqtqf")
public suspend fun changedTime(`value`: Output) {
this.changedTime = value
}
/**
* @param value
*/
@JvmName("itrqnmfwkcgeclsf")
public suspend fun content(`value`: Output) {
this.content = value
}
/**
* @param value The content link.
*/
@JvmName("gumjtiohwckunrqj")
public suspend fun contentLink(`value`: Output) {
this.contentLink = value
}
/**
* @param value The content type.
*/
@JvmName("sxplxtbeqtkkevgg")
public suspend fun contentType(`value`: Output) {
this.contentType = value
}
/**
* @param value The artifact creation time.
*/
@JvmName("deejikswlbuiyism")
public suspend fun createdTime(`value`: Output) {
this.createdTime = value
}
/**
* @param value
*/
@JvmName("pncokojbretujrkb")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value The assembly culture.
*/
@JvmName("wppfmagbewtqgoee")
public suspend fun assemblyCulture(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.assemblyCulture = mapped
}
/**
* @param value The assembly name.
*/
@JvmName("rbljqfwcjtwfsndt")
public suspend fun assemblyName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.assemblyName = mapped
}
/**
* @param value The assembly public key token.
*/
@JvmName("ustrypowlkdekhan")
public suspend fun assemblyPublicKeyToken(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.assemblyPublicKeyToken = mapped
}
/**
* @param value The assembly version.
*/
@JvmName("yampcwlwprhbfqty")
public suspend fun assemblyVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.assemblyVersion = mapped
}
/**
* @param value The artifact changed time.
*/
@JvmName("nodvrvahkxpixcly")
public suspend fun changedTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.changedTime = mapped
}
/**
* @param value
*/
@JvmName("ityrnxafsmbfulyp")
public suspend fun content(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.content = mapped
}
/**
* @param value The content link.
*/
@JvmName("otnhlcvsqsrpotpb")
public suspend fun contentLink(`value`: ContentLinkArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentLink = mapped
}
/**
* @param argument The content link.
*/
@JvmName("ummcplyhfvmbomgp")
public suspend fun contentLink(argument: suspend ContentLinkArgsBuilder.() -> Unit) {
val toBeMapped = ContentLinkArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.contentLink = mapped
}
/**
* @param value The content type.
*/
@JvmName("pdgsqmoumdaspele")
public suspend fun contentType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value The artifact creation time.
*/
@JvmName("hkuowfynakqmnqas")
public suspend fun createdTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createdTime = mapped
}
/**
* @param value
*/
@JvmName("sjoaxvtvqoapvdld")
public suspend fun metadata(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
internal fun build(): AssemblyPropertiesArgs = AssemblyPropertiesArgs(
assemblyCulture = assemblyCulture,
assemblyName = assemblyName ?: throw PulumiNullFieldException("assemblyName"),
assemblyPublicKeyToken = assemblyPublicKeyToken,
assemblyVersion = assemblyVersion,
changedTime = changedTime,
content = content,
contentLink = contentLink,
contentType = contentType,
createdTime = createdTime,
metadata = metadata,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy