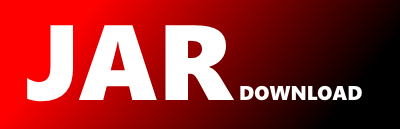
com.pulumi.azurenative.logic.kotlin.inputs.X12EnvelopeOverrideArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin.inputs
import com.pulumi.azurenative.logic.inputs.X12EnvelopeOverrideArgs.builder
import com.pulumi.azurenative.logic.kotlin.enums.X12DateFormat
import com.pulumi.azurenative.logic.kotlin.enums.X12TimeFormat
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The X12 envelope override settings.
* @property dateFormat The date format.
* @property functionalIdentifierCode The functional identifier code.
* @property headerVersion The header version.
* @property messageId The message id on which this envelope settings has to be applied.
* @property protocolVersion The protocol version on which this envelope settings has to be applied.
* @property receiverApplicationId The receiver application id.
* @property responsibleAgencyCode The responsible agency code.
* @property senderApplicationId The sender application id.
* @property targetNamespace The target namespace on which this envelope settings has to be applied.
* @property timeFormat The time format.
*/
public data class X12EnvelopeOverrideArgs(
public val dateFormat: Output>,
public val functionalIdentifierCode: Output? = null,
public val headerVersion: Output,
public val messageId: Output,
public val protocolVersion: Output,
public val receiverApplicationId: Output,
public val responsibleAgencyCode: Output,
public val senderApplicationId: Output,
public val targetNamespace: Output,
public val timeFormat: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logic.inputs.X12EnvelopeOverrideArgs =
com.pulumi.azurenative.logic.inputs.X12EnvelopeOverrideArgs.builder()
.dateFormat(
dateFormat.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.functionalIdentifierCode(functionalIdentifierCode?.applyValue({ args0 -> args0 }))
.headerVersion(headerVersion.applyValue({ args0 -> args0 }))
.messageId(messageId.applyValue({ args0 -> args0 }))
.protocolVersion(protocolVersion.applyValue({ args0 -> args0 }))
.receiverApplicationId(receiverApplicationId.applyValue({ args0 -> args0 }))
.responsibleAgencyCode(responsibleAgencyCode.applyValue({ args0 -> args0 }))
.senderApplicationId(senderApplicationId.applyValue({ args0 -> args0 }))
.targetNamespace(targetNamespace.applyValue({ args0 -> args0 }))
.timeFormat(
timeFormat.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [X12EnvelopeOverrideArgs].
*/
@PulumiTagMarker
public class X12EnvelopeOverrideArgsBuilder internal constructor() {
private var dateFormat: Output>? = null
private var functionalIdentifierCode: Output? = null
private var headerVersion: Output? = null
private var messageId: Output? = null
private var protocolVersion: Output? = null
private var receiverApplicationId: Output? = null
private var responsibleAgencyCode: Output? = null
private var senderApplicationId: Output? = null
private var targetNamespace: Output? = null
private var timeFormat: Output>? = null
/**
* @param value The date format.
*/
@JvmName("htyhsitwrjkkwoud")
public suspend fun dateFormat(`value`: Output>) {
this.dateFormat = value
}
/**
* @param value The functional identifier code.
*/
@JvmName("enxsesuxntfcxomi")
public suspend fun functionalIdentifierCode(`value`: Output) {
this.functionalIdentifierCode = value
}
/**
* @param value The header version.
*/
@JvmName("lvrtggmfwlmjxknb")
public suspend fun headerVersion(`value`: Output) {
this.headerVersion = value
}
/**
* @param value The message id on which this envelope settings has to be applied.
*/
@JvmName("xkanppscqhuneyoy")
public suspend fun messageId(`value`: Output) {
this.messageId = value
}
/**
* @param value The protocol version on which this envelope settings has to be applied.
*/
@JvmName("pogdgkjvoyumtivb")
public suspend fun protocolVersion(`value`: Output) {
this.protocolVersion = value
}
/**
* @param value The receiver application id.
*/
@JvmName("wavkrydqajingpqq")
public suspend fun receiverApplicationId(`value`: Output) {
this.receiverApplicationId = value
}
/**
* @param value The responsible agency code.
*/
@JvmName("ahyyhieomewqpcvu")
public suspend fun responsibleAgencyCode(`value`: Output) {
this.responsibleAgencyCode = value
}
/**
* @param value The sender application id.
*/
@JvmName("jreknpfvbxaovjvk")
public suspend fun senderApplicationId(`value`: Output) {
this.senderApplicationId = value
}
/**
* @param value The target namespace on which this envelope settings has to be applied.
*/
@JvmName("sjsvhypesdelrerf")
public suspend fun targetNamespace(`value`: Output) {
this.targetNamespace = value
}
/**
* @param value The time format.
*/
@JvmName("opndtarraiudtaba")
public suspend fun timeFormat(`value`: Output>) {
this.timeFormat = value
}
/**
* @param value The date format.
*/
@JvmName("wuhtfduxwwxoakli")
public suspend fun dateFormat(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.dateFormat = mapped
}
/**
* @param value The date format.
*/
@JvmName("uedgmrpmaqvbehcr")
public fun dateFormat(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.dateFormat = mapped
}
/**
* @param value The date format.
*/
@JvmName("texbaupmxpvsdmcc")
public fun dateFormat(`value`: X12DateFormat) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.dateFormat = mapped
}
/**
* @param value The functional identifier code.
*/
@JvmName("acqilfdgwjlbkigh")
public suspend fun functionalIdentifierCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.functionalIdentifierCode = mapped
}
/**
* @param value The header version.
*/
@JvmName("mlbytvgpkwmmqtax")
public suspend fun headerVersion(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.headerVersion = mapped
}
/**
* @param value The message id on which this envelope settings has to be applied.
*/
@JvmName("vaxyklsrcigosuxr")
public suspend fun messageId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.messageId = mapped
}
/**
* @param value The protocol version on which this envelope settings has to be applied.
*/
@JvmName("bodgepjydecrojcu")
public suspend fun protocolVersion(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.protocolVersion = mapped
}
/**
* @param value The receiver application id.
*/
@JvmName("knailhorygtmdiow")
public suspend fun receiverApplicationId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.receiverApplicationId = mapped
}
/**
* @param value The responsible agency code.
*/
@JvmName("pnkhlwsxxonfmagk")
public suspend fun responsibleAgencyCode(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.responsibleAgencyCode = mapped
}
/**
* @param value The sender application id.
*/
@JvmName("ecyiedvnufqqnsct")
public suspend fun senderApplicationId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.senderApplicationId = mapped
}
/**
* @param value The target namespace on which this envelope settings has to be applied.
*/
@JvmName("vjsoioeodsxximfg")
public suspend fun targetNamespace(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.targetNamespace = mapped
}
/**
* @param value The time format.
*/
@JvmName("hfcjrrynboqrbebw")
public suspend fun timeFormat(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeFormat = mapped
}
/**
* @param value The time format.
*/
@JvmName("hksdhodrxqbkqeau")
public fun timeFormat(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeFormat = mapped
}
/**
* @param value The time format.
*/
@JvmName("ogrrxmyvnlkkhicy")
public fun timeFormat(`value`: X12TimeFormat) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeFormat = mapped
}
internal fun build(): X12EnvelopeOverrideArgs = X12EnvelopeOverrideArgs(
dateFormat = dateFormat ?: throw PulumiNullFieldException("dateFormat"),
functionalIdentifierCode = functionalIdentifierCode,
headerVersion = headerVersion ?: throw PulumiNullFieldException("headerVersion"),
messageId = messageId ?: throw PulumiNullFieldException("messageId"),
protocolVersion = protocolVersion ?: throw PulumiNullFieldException("protocolVersion"),
receiverApplicationId = receiverApplicationId ?: throw
PulumiNullFieldException("receiverApplicationId"),
responsibleAgencyCode = responsibleAgencyCode ?: throw
PulumiNullFieldException("responsibleAgencyCode"),
senderApplicationId = senderApplicationId ?: throw PulumiNullFieldException("senderApplicationId"),
targetNamespace = targetNamespace ?: throw PulumiNullFieldException("targetNamespace"),
timeFormat = timeFormat ?: throw PulumiNullFieldException("timeFormat"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy