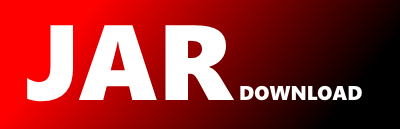
com.pulumi.azurenative.logic.kotlin.inputs.X12ProcessingSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin.inputs
import com.pulumi.azurenative.logic.inputs.X12ProcessingSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The X12 processing settings.
* @property convertImpliedDecimal The value indicating whether to convert numerical type to implied decimal.
* @property createEmptyXmlTagsForTrailingSeparators The value indicating whether to create empty xml tags for trailing separators.
* @property maskSecurityInfo The value indicating whether to mask security information.
* @property preserveInterchange The value indicating whether to preserve interchange.
* @property suspendInterchangeOnError The value indicating whether to suspend interchange on error.
* @property useDotAsDecimalSeparator The value indicating whether to use dot as decimal separator.
*/
public data class X12ProcessingSettingsArgs(
public val convertImpliedDecimal: Output,
public val createEmptyXmlTagsForTrailingSeparators: Output,
public val maskSecurityInfo: Output,
public val preserveInterchange: Output,
public val suspendInterchangeOnError: Output,
public val useDotAsDecimalSeparator: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logic.inputs.X12ProcessingSettingsArgs =
com.pulumi.azurenative.logic.inputs.X12ProcessingSettingsArgs.builder()
.convertImpliedDecimal(convertImpliedDecimal.applyValue({ args0 -> args0 }))
.createEmptyXmlTagsForTrailingSeparators(
createEmptyXmlTagsForTrailingSeparators.applyValue({ args0 ->
args0
}),
)
.maskSecurityInfo(maskSecurityInfo.applyValue({ args0 -> args0 }))
.preserveInterchange(preserveInterchange.applyValue({ args0 -> args0 }))
.suspendInterchangeOnError(suspendInterchangeOnError.applyValue({ args0 -> args0 }))
.useDotAsDecimalSeparator(useDotAsDecimalSeparator.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [X12ProcessingSettingsArgs].
*/
@PulumiTagMarker
public class X12ProcessingSettingsArgsBuilder internal constructor() {
private var convertImpliedDecimal: Output? = null
private var createEmptyXmlTagsForTrailingSeparators: Output? = null
private var maskSecurityInfo: Output? = null
private var preserveInterchange: Output? = null
private var suspendInterchangeOnError: Output? = null
private var useDotAsDecimalSeparator: Output? = null
/**
* @param value The value indicating whether to convert numerical type to implied decimal.
*/
@JvmName("spursplijmntcfot")
public suspend fun convertImpliedDecimal(`value`: Output) {
this.convertImpliedDecimal = value
}
/**
* @param value The value indicating whether to create empty xml tags for trailing separators.
*/
@JvmName("fpcqeauirrpiitpm")
public suspend fun createEmptyXmlTagsForTrailingSeparators(`value`: Output) {
this.createEmptyXmlTagsForTrailingSeparators = value
}
/**
* @param value The value indicating whether to mask security information.
*/
@JvmName("msxcawntimoauvxg")
public suspend fun maskSecurityInfo(`value`: Output) {
this.maskSecurityInfo = value
}
/**
* @param value The value indicating whether to preserve interchange.
*/
@JvmName("sfkbspticetvmlrg")
public suspend fun preserveInterchange(`value`: Output) {
this.preserveInterchange = value
}
/**
* @param value The value indicating whether to suspend interchange on error.
*/
@JvmName("egcrmpcguraviqfv")
public suspend fun suspendInterchangeOnError(`value`: Output) {
this.suspendInterchangeOnError = value
}
/**
* @param value The value indicating whether to use dot as decimal separator.
*/
@JvmName("bniccovgnoxssgum")
public suspend fun useDotAsDecimalSeparator(`value`: Output) {
this.useDotAsDecimalSeparator = value
}
/**
* @param value The value indicating whether to convert numerical type to implied decimal.
*/
@JvmName("nhvdeirmwwrqqsmq")
public suspend fun convertImpliedDecimal(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.convertImpliedDecimal = mapped
}
/**
* @param value The value indicating whether to create empty xml tags for trailing separators.
*/
@JvmName("yndcdvqtuutsmstw")
public suspend fun createEmptyXmlTagsForTrailingSeparators(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.createEmptyXmlTagsForTrailingSeparators = mapped
}
/**
* @param value The value indicating whether to mask security information.
*/
@JvmName("gwkwtheuhqxmcdex")
public suspend fun maskSecurityInfo(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.maskSecurityInfo = mapped
}
/**
* @param value The value indicating whether to preserve interchange.
*/
@JvmName("eglpiduhebswcklc")
public suspend fun preserveInterchange(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.preserveInterchange = mapped
}
/**
* @param value The value indicating whether to suspend interchange on error.
*/
@JvmName("nfkycdvhqxmsnjpy")
public suspend fun suspendInterchangeOnError(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.suspendInterchangeOnError = mapped
}
/**
* @param value The value indicating whether to use dot as decimal separator.
*/
@JvmName("aivsngbgsbhtwuli")
public suspend fun useDotAsDecimalSeparator(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.useDotAsDecimalSeparator = mapped
}
internal fun build(): X12ProcessingSettingsArgs = X12ProcessingSettingsArgs(
convertImpliedDecimal = convertImpliedDecimal ?: throw
PulumiNullFieldException("convertImpliedDecimal"),
createEmptyXmlTagsForTrailingSeparators = createEmptyXmlTagsForTrailingSeparators ?: throw
PulumiNullFieldException("createEmptyXmlTagsForTrailingSeparators"),
maskSecurityInfo = maskSecurityInfo ?: throw PulumiNullFieldException("maskSecurityInfo"),
preserveInterchange = preserveInterchange ?: throw PulumiNullFieldException("preserveInterchange"),
suspendInterchangeOnError = suspendInterchangeOnError ?: throw
PulumiNullFieldException("suspendInterchangeOnError"),
useDotAsDecimalSeparator = useDotAsDecimalSeparator ?: throw
PulumiNullFieldException("useDotAsDecimalSeparator"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy