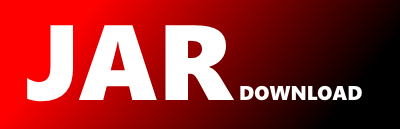
com.pulumi.azurenative.logic.kotlin.inputs.X12ProtocolSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logic.kotlin.inputs
import com.pulumi.azurenative.logic.inputs.X12ProtocolSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The X12 agreement protocol settings.
* @property acknowledgementSettings The X12 acknowledgment settings.
* @property envelopeOverrides The X12 envelope override settings.
* @property envelopeSettings The X12 envelope settings.
* @property framingSettings The X12 framing settings.
* @property messageFilter The X12 message filter.
* @property messageFilterList The X12 message filter list.
* @property processingSettings The X12 processing settings.
* @property schemaReferences The X12 schema references.
* @property securitySettings The X12 security settings.
* @property validationOverrides The X12 validation override settings.
* @property validationSettings The X12 validation settings.
* @property x12DelimiterOverrides The X12 delimiter override settings.
*/
public data class X12ProtocolSettingsArgs(
public val acknowledgementSettings: Output,
public val envelopeOverrides: Output>? = null,
public val envelopeSettings: Output,
public val framingSettings: Output,
public val messageFilter: Output,
public val messageFilterList: Output>? = null,
public val processingSettings: Output,
public val schemaReferences: Output>,
public val securitySettings: Output,
public val validationOverrides: Output>? = null,
public val validationSettings: Output,
public val x12DelimiterOverrides: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logic.inputs.X12ProtocolSettingsArgs =
com.pulumi.azurenative.logic.inputs.X12ProtocolSettingsArgs.builder()
.acknowledgementSettings(
acknowledgementSettings.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.envelopeOverrides(
envelopeOverrides?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.envelopeSettings(envelopeSettings.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.framingSettings(framingSettings.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.messageFilter(messageFilter.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.messageFilterList(
messageFilterList?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.processingSettings(
processingSettings.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.schemaReferences(
schemaReferences.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.securitySettings(securitySettings.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.validationOverrides(
validationOverrides?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.validationSettings(
validationSettings.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.x12DelimiterOverrides(
x12DelimiterOverrides?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [X12ProtocolSettingsArgs].
*/
@PulumiTagMarker
public class X12ProtocolSettingsArgsBuilder internal constructor() {
private var acknowledgementSettings: Output? = null
private var envelopeOverrides: Output>? = null
private var envelopeSettings: Output? = null
private var framingSettings: Output? = null
private var messageFilter: Output? = null
private var messageFilterList: Output>? = null
private var processingSettings: Output? = null
private var schemaReferences: Output>? = null
private var securitySettings: Output? = null
private var validationOverrides: Output>? = null
private var validationSettings: Output? = null
private var x12DelimiterOverrides: Output>? = null
/**
* @param value The X12 acknowledgment settings.
*/
@JvmName("kbysmrqkvvlmdbwx")
public suspend fun acknowledgementSettings(`value`: Output) {
this.acknowledgementSettings = value
}
/**
* @param value The X12 envelope override settings.
*/
@JvmName("inodrtdpxsqsqjlq")
public suspend fun envelopeOverrides(`value`: Output>) {
this.envelopeOverrides = value
}
@JvmName("aimwnfyvgyovcstp")
public suspend fun envelopeOverrides(vararg values: Output) {
this.envelopeOverrides = Output.all(values.asList())
}
/**
* @param values The X12 envelope override settings.
*/
@JvmName("bpkuxeeqkeglvnby")
public suspend fun envelopeOverrides(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy