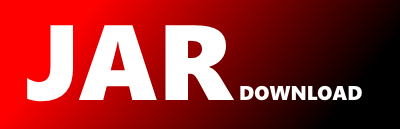
com.pulumi.azurenative.logz.kotlin.inputs.MonitorPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.logz.kotlin.inputs
import com.pulumi.azurenative.logz.inputs.MonitorPropertiesArgs.builder
import com.pulumi.azurenative.logz.kotlin.enums.MarketplaceSubscriptionStatus
import com.pulumi.azurenative.logz.kotlin.enums.MonitoringStatus
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Properties specific to the monitor resource.
* @property logzOrganizationProperties
* @property marketplaceSubscriptionStatus Flag specifying the Marketplace Subscription Status of the resource. If payment is not made in time, the resource will go in Suspended state.
* @property monitoringStatus Flag specifying if the resource monitoring is enabled or disabled.
* @property planData
* @property userInfo
*/
public data class MonitorPropertiesArgs(
public val logzOrganizationProperties: Output? = null,
public val marketplaceSubscriptionStatus: Output>? =
null,
public val monitoringStatus: Output>? = null,
public val planData: Output? = null,
public val userInfo: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.logz.inputs.MonitorPropertiesArgs =
com.pulumi.azurenative.logz.inputs.MonitorPropertiesArgs.builder()
.logzOrganizationProperties(
logzOrganizationProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.marketplaceSubscriptionStatus(
marketplaceSubscriptionStatus?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.monitoringStatus(
monitoringStatus?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.planData(planData?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.userInfo(userInfo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [MonitorPropertiesArgs].
*/
@PulumiTagMarker
public class MonitorPropertiesArgsBuilder internal constructor() {
private var logzOrganizationProperties: Output? = null
private var marketplaceSubscriptionStatus: Output>? =
null
private var monitoringStatus: Output>? = null
private var planData: Output? = null
private var userInfo: Output? = null
/**
* @param value
*/
@JvmName("ajivjogrrtluqevm")
public suspend fun logzOrganizationProperties(`value`: Output) {
this.logzOrganizationProperties = value
}
/**
* @param value Flag specifying the Marketplace Subscription Status of the resource. If payment is not made in time, the resource will go in Suspended state.
*/
@JvmName("edoyknsyltihudsi")
public suspend fun marketplaceSubscriptionStatus(`value`: Output>) {
this.marketplaceSubscriptionStatus = value
}
/**
* @param value Flag specifying if the resource monitoring is enabled or disabled.
*/
@JvmName("qtayxckhbeefsykx")
public suspend fun monitoringStatus(`value`: Output>) {
this.monitoringStatus = value
}
/**
* @param value
*/
@JvmName("qttqfietsiddocie")
public suspend fun planData(`value`: Output) {
this.planData = value
}
/**
* @param value
*/
@JvmName("ygeavxvkyaicjsjv")
public suspend fun userInfo(`value`: Output) {
this.userInfo = value
}
/**
* @param value
*/
@JvmName("tamrrinlpawyrmtv")
public suspend fun logzOrganizationProperties(`value`: LogzOrganizationPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logzOrganizationProperties = mapped
}
/**
* @param argument
*/
@JvmName("ainnmqewblhrfcfy")
public suspend fun logzOrganizationProperties(argument: suspend LogzOrganizationPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = LogzOrganizationPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.logzOrganizationProperties = mapped
}
/**
* @param value Flag specifying the Marketplace Subscription Status of the resource. If payment is not made in time, the resource will go in Suspended state.
*/
@JvmName("lpvepwyllqgwfhvj")
public suspend fun marketplaceSubscriptionStatus(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.marketplaceSubscriptionStatus = mapped
}
/**
* @param value Flag specifying the Marketplace Subscription Status of the resource. If payment is not made in time, the resource will go in Suspended state.
*/
@JvmName("pcqbdchgofrfjdcm")
public fun marketplaceSubscriptionStatus(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.marketplaceSubscriptionStatus = mapped
}
/**
* @param value Flag specifying the Marketplace Subscription Status of the resource. If payment is not made in time, the resource will go in Suspended state.
*/
@JvmName("ncsqoyttitchntsr")
public fun marketplaceSubscriptionStatus(`value`: MarketplaceSubscriptionStatus) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.marketplaceSubscriptionStatus = mapped
}
/**
* @param value Flag specifying if the resource monitoring is enabled or disabled.
*/
@JvmName("dwoefdbremfhlhkv")
public suspend fun monitoringStatus(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitoringStatus = mapped
}
/**
* @param value Flag specifying if the resource monitoring is enabled or disabled.
*/
@JvmName("xhbyspnnpvgwfuph")
public fun monitoringStatus(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.monitoringStatus = mapped
}
/**
* @param value Flag specifying if the resource monitoring is enabled or disabled.
*/
@JvmName("ksyebvtsrpkeosta")
public fun monitoringStatus(`value`: MonitoringStatus) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.monitoringStatus = mapped
}
/**
* @param value
*/
@JvmName("dppflgqayaaasntn")
public suspend fun planData(`value`: PlanDataArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.planData = mapped
}
/**
* @param argument
*/
@JvmName("jhqedytjkkfuvofh")
public suspend fun planData(argument: suspend PlanDataArgsBuilder.() -> Unit) {
val toBeMapped = PlanDataArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.planData = mapped
}
/**
* @param value
*/
@JvmName("cbkapabqmaotpaim")
public suspend fun userInfo(`value`: UserInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userInfo = mapped
}
/**
* @param argument
*/
@JvmName("vnjudjcgpbyoqseh")
public suspend fun userInfo(argument: suspend UserInfoArgsBuilder.() -> Unit) {
val toBeMapped = UserInfoArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.userInfo = mapped
}
internal fun build(): MonitorPropertiesArgs = MonitorPropertiesArgs(
logzOrganizationProperties = logzOrganizationProperties,
marketplaceSubscriptionStatus = marketplaceSubscriptionStatus,
monitoringStatus = monitoringStatus,
planData = planData,
userInfo = userInfo,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy