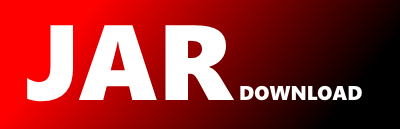
com.pulumi.azurenative.machinelearningservices.kotlin.LabelingJobArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.machinelearningservices.kotlin
import com.pulumi.azurenative.machinelearningservices.LabelingJobArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Azure Resource Manager resource envelope.
* Azure REST API version: 2023-04-01-preview. Prior API version in Azure Native 1.x: 2020-09-01-preview.
* Other available API versions: 2020-09-01-preview, 2021-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-04-01-preview.
* ## Example Usage
* ### CreateOrUpdate Labeling Job.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var labelingJob = new AzureNative.MachineLearningServices.LabelingJob("labelingJob", new()
* {
* Id = "testLabelingJob",
* LabelingJobProperties = new AzureNative.MachineLearningServices.Inputs.LabelingJobArgs
* {
* Description = "string",
* JobInstructions = new AzureNative.MachineLearningServices.Inputs.LabelingJobInstructionsArgs
* {
* Uri = "link/to/instructions",
* },
* JobType = "Labeling",
* LabelCategories =
* {
* { "myCategory1", new AzureNative.MachineLearningServices.Inputs.LabelCategoryArgs
* {
* Classes =
* {
* { "myLabelClass1", new AzureNative.MachineLearningServices.Inputs.LabelClassArgs
* {
* DisplayName = "myLabelClass1",
* Subclasses = null,
* } },
* { "myLabelClass2", new AzureNative.MachineLearningServices.Inputs.LabelClassArgs
* {
* DisplayName = "myLabelClass2",
* Subclasses = null,
* } },
* },
* DisplayName = "myCategory1Title",
* MultiSelect = AzureNative.MachineLearningServices.MultiSelect.Disabled,
* } },
* { "myCategory2", new AzureNative.MachineLearningServices.Inputs.LabelCategoryArgs
* {
* Classes =
* {
* { "myLabelClass1", new AzureNative.MachineLearningServices.Inputs.LabelClassArgs
* {
* DisplayName = "myLabelClass1",
* Subclasses = null,
* } },
* { "myLabelClass2", new AzureNative.MachineLearningServices.Inputs.LabelClassArgs
* {
* DisplayName = "myLabelClass2",
* Subclasses = null,
* } },
* },
* DisplayName = "myCategory2Title",
* MultiSelect = AzureNative.MachineLearningServices.MultiSelect.Disabled,
* } },
* },
* LabelingJobMediaProperties = new AzureNative.MachineLearningServices.Inputs.LabelingJobImagePropertiesArgs
* {
* MediaType = "Image",
* },
* MlAssistConfiguration = new AzureNative.MachineLearningServices.Inputs.MLAssistConfigurationEnabledArgs
* {
* InferencingComputeBinding = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/resourceGroup-1234/providers/Microsoft.MachineLearningServices/workspaces/testworkspace/computes/myscoringcompute",
* MlAssist = "Enabled",
* TrainingComputeBinding = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/resourceGroup-1234/providers/Microsoft.MachineLearningServices/workspaces/testworkspace/computes/mytrainingompute",
* },
* Properties =
* {
* { "additionalProp1", "string" },
* { "additionalProp2", "string" },
* { "additionalProp3", "string" },
* },
* Tags =
* {
* { "additionalProp1", "string" },
* { "additionalProp2", "string" },
* { "additionalProp3", "string" },
* },
* },
* ResourceGroupName = "workspace-1234",
* WorkspaceName = "testworkspace",
* });
* });
* ```
* ```go
* package main
* import (
* machinelearningservices "github.com/pulumi/pulumi-azure-native-sdk/machinelearningservices/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := machinelearningservices.NewLabelingJob(ctx, "labelingJob", &machinelearningservices.LabelingJobArgs{
* Id: pulumi.String("testLabelingJob"),
* LabelingJobProperties: &machinelearningservices.LabelingJobTypeArgs{
* Description: pulumi.String("string"),
* JobInstructions: &machinelearningservices.LabelingJobInstructionsArgs{
* Uri: pulumi.String("link/to/instructions"),
* },
* JobType: pulumi.String("Labeling"),
* LabelCategories: machinelearningservices.LabelCategoryMap{
* "myCategory1": &machinelearningservices.LabelCategoryArgs{
* Classes: machinelearningservices.LabelClassMap{
* "myLabelClass1": &machinelearningservices.LabelClassArgs{
* DisplayName: pulumi.String("myLabelClass1"),
* Subclasses: nil,
* },
* "myLabelClass2": &machinelearningservices.LabelClassArgs{
* DisplayName: pulumi.String("myLabelClass2"),
* Subclasses: nil,
* },
* },
* DisplayName: pulumi.String("myCategory1Title"),
* MultiSelect: pulumi.String(machinelearningservices.MultiSelectDisabled),
* },
* "myCategory2": &machinelearningservices.LabelCategoryArgs{
* Classes: machinelearningservices.LabelClassMap{
* "myLabelClass1": &machinelearningservices.LabelClassArgs{
* DisplayName: pulumi.String("myLabelClass1"),
* Subclasses: nil,
* },
* "myLabelClass2": &machinelearningservices.LabelClassArgs{
* DisplayName: pulumi.String("myLabelClass2"),
* Subclasses: nil,
* },
* },
* DisplayName: pulumi.String("myCategory2Title"),
* MultiSelect: pulumi.String(machinelearningservices.MultiSelectDisabled),
* },
* },
* LabelingJobMediaProperties: machinelearningservices.LabelingJobImageProperties{
* MediaType: "Image",
* },
* MlAssistConfiguration: machinelearningservices.MLAssistConfigurationEnabled{
* InferencingComputeBinding: "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/resourceGroup-1234/providers/Microsoft.MachineLearningServices/workspaces/testworkspace/computes/myscoringcompute",
* MlAssist: "Enabled",
* TrainingComputeBinding: "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/resourceGroup-1234/providers/Microsoft.MachineLearningServices/workspaces/testworkspace/computes/mytrainingompute",
* },
* Properties: pulumi.StringMap{
* "additionalProp1": pulumi.String("string"),
* "additionalProp2": pulumi.String("string"),
* "additionalProp3": pulumi.String("string"),
* },
* Tags: pulumi.StringMap{
* "additionalProp1": pulumi.String("string"),
* "additionalProp2": pulumi.String("string"),
* "additionalProp3": pulumi.String("string"),
* },
* },
* ResourceGroupName: pulumi.String("workspace-1234"),
* WorkspaceName: pulumi.String("testworkspace"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.machinelearningservices.LabelingJob;
* import com.pulumi.azurenative.machinelearningservices.LabelingJobArgs;
* import com.pulumi.azurenative.machinelearningservices.inputs.LabelingJobArgs;
* import com.pulumi.azurenative.machinelearningservices.inputs.LabelingJobInstructionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var labelingJob = new LabelingJob("labelingJob", LabelingJobArgs.builder()
* .id("testLabelingJob")
* .labelingJobProperties(LabelingJobArgs.builder()
* .description("string")
* .jobInstructions(LabelingJobInstructionsArgs.builder()
* .uri("link/to/instructions")
* .build())
* .jobType("Labeling")
* .labelCategories(Map.ofEntries(
* Map.entry("myCategory1", Map.ofEntries(
* Map.entry("classes", Map.ofEntries(
* Map.entry("myLabelClass1", Map.ofEntries(
* Map.entry("displayName", "myLabelClass1"),
* Map.entry("subclasses", )
* )),
* Map.entry("myLabelClass2", Map.ofEntries(
* Map.entry("displayName", "myLabelClass2"),
* Map.entry("subclasses", )
* ))
* )),
* Map.entry("displayName", "myCategory1Title"),
* Map.entry("multiSelect", "Disabled")
* )),
* Map.entry("myCategory2", Map.ofEntries(
* Map.entry("classes", Map.ofEntries(
* Map.entry("myLabelClass1", Map.ofEntries(
* Map.entry("displayName", "myLabelClass1"),
* Map.entry("subclasses", )
* )),
* Map.entry("myLabelClass2", Map.ofEntries(
* Map.entry("displayName", "myLabelClass2"),
* Map.entry("subclasses", )
* ))
* )),
* Map.entry("displayName", "myCategory2Title"),
* Map.entry("multiSelect", "Disabled")
* ))
* ))
* .labelingJobMediaProperties(LabelingJobImagePropertiesArgs.builder()
* .mediaType("Image")
* .build())
* .mlAssistConfiguration(MLAssistConfigurationEnabledArgs.builder()
* .inferencingComputeBinding("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/resourceGroup-1234/providers/Microsoft.MachineLearningServices/workspaces/testworkspace/computes/myscoringcompute")
* .mlAssist("Enabled")
* .trainingComputeBinding("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/resourceGroup-1234/providers/Microsoft.MachineLearningServices/workspaces/testworkspace/computes/mytrainingompute")
* .build())
* .properties(Map.ofEntries(
* Map.entry("additionalProp1", "string"),
* Map.entry("additionalProp2", "string"),
* Map.entry("additionalProp3", "string")
* ))
* .tags(Map.ofEntries(
* Map.entry("additionalProp1", "string"),
* Map.entry("additionalProp2", "string"),
* Map.entry("additionalProp3", "string")
* ))
* .build())
* .resourceGroupName("workspace-1234")
* .workspaceName("testworkspace")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:machinelearningservices:LabelingJob testLabelingJob /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.MachineLearningServices/workspaces/{workspaceName}/labelingJobs/{id}
* ```
* @property id The name and identifier for the LabelingJob.
* @property labelingJobProperties [Required] Additional attributes of the entity.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property workspaceName Name of Azure Machine Learning workspace.
*/
public data class LabelingJobArgs(
public val id: Output? = null,
public val labelingJobProperties: Output? = null,
public val resourceGroupName: Output? = null,
public val workspaceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.machinelearningservices.LabelingJobArgs =
com.pulumi.azurenative.machinelearningservices.LabelingJobArgs.builder()
.id(id?.applyValue({ args0 -> args0 }))
.labelingJobProperties(
labelingJobProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.workspaceName(workspaceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LabelingJobArgs].
*/
@PulumiTagMarker
public class LabelingJobArgsBuilder internal constructor() {
private var id: Output? = null
private var labelingJobProperties:
Output? = null
private var resourceGroupName: Output? = null
private var workspaceName: Output? = null
/**
* @param value The name and identifier for the LabelingJob.
*/
@JvmName("qkrsgwivdlafnosq")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value [Required] Additional attributes of the entity.
*/
@JvmName("hoitingblyhtradl")
public suspend fun labelingJobProperties(`value`: Output) {
this.labelingJobProperties = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("lfchospujlriuhjw")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Name of Azure Machine Learning workspace.
*/
@JvmName("puxragvctcdhodog")
public suspend fun workspaceName(`value`: Output) {
this.workspaceName = value
}
/**
* @param value The name and identifier for the LabelingJob.
*/
@JvmName("bdjmwbbjkdjpiynr")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value [Required] Additional attributes of the entity.
*/
@JvmName("wtedapsyvvyhuroq")
public suspend fun labelingJobProperties(`value`: com.pulumi.azurenative.machinelearningservices.kotlin.inputs.LabelingJobArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.labelingJobProperties = mapped
}
/**
* @param argument [Required] Additional attributes of the entity.
*/
@JvmName("spmnjeepruukybuy")
public suspend fun labelingJobProperties(argument: suspend com.pulumi.azurenative.machinelearningservices.kotlin.inputs.LabelingJobArgsBuilder.() -> Unit) {
val toBeMapped =
com.pulumi.azurenative.machinelearningservices.kotlin.inputs.LabelingJobArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.labelingJobProperties = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("tkrirftyhcnvwwpr")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Name of Azure Machine Learning workspace.
*/
@JvmName("falxcdrjxitlfvop")
public suspend fun workspaceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workspaceName = mapped
}
internal fun build(): LabelingJobArgs = LabelingJobArgs(
id = id,
labelingJobProperties = labelingJobProperties,
resourceGroupName = resourceGroupName,
workspaceName = workspaceName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy