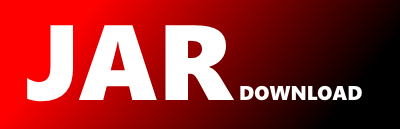
com.pulumi.azurenative.machinelearningservices.kotlin.inputs.ModelVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.machinelearningservices.kotlin.inputs
import com.pulumi.azurenative.machinelearningservices.inputs.ModelVersionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Model asset version details.
* @property description The asset description text.
* @property flavors Mapping of model flavors to their properties.
* @property isAnonymous If the name version are system generated (anonymous registration).
* @property isArchived Is the asset archived?
* @property jobName Name of the training job which produced this model
* @property modelType The storage format for this entity. Used for NCD.
* @property modelUri The URI path to the model contents.
* @property properties The asset property dictionary.
* @property stage Stage in the model lifecycle assigned to this model
* @property tags Tag dictionary. Tags can be added, removed, and updated.
*/
public data class ModelVersionArgs(
public val description: Output? = null,
public val flavors: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy