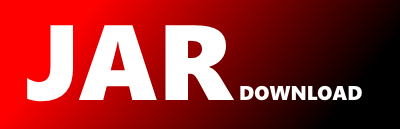
com.pulumi.azurenative.managednetworkfabric.kotlin.NetworkFabricArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.managednetworkfabric.kotlin
import com.pulumi.azurenative.managednetworkfabric.NetworkFabricArgs.builder
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.ManagementNetworkConfigurationArgs
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.ManagementNetworkConfigurationArgsBuilder
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.TerminalServerConfigurationArgs
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.TerminalServerConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The NetworkFabric resource definition.
* Azure REST API version: 2023-02-01-preview. Prior API version in Azure Native 1.x: 2023-02-01-preview.
* Other available API versions: 2023-06-15.
* ## Example Usage
* ### NetworkFabrics_Create_MaximumSet_Gen
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var networkFabric = new AzureNative.ManagedNetworkFabric.NetworkFabric("networkFabric", new()
* {
* Annotation = "annotationValue",
* FabricASN = 29249,
* Ipv4Prefix = "10.18.0.0/19",
* Ipv6Prefix = "3FFE:FFFF:0:CD40::/59",
* Location = "eastuseuap",
* ManagementNetworkConfiguration = new AzureNative.ManagedNetworkFabric.Inputs.ManagementNetworkConfigurationArgs
* {
* InfrastructureVpnConfiguration = new AzureNative.ManagedNetworkFabric.Inputs.VpnConfigurationPropertiesArgs
* {
* OptionAProperties = new AzureNative.ManagedNetworkFabric.Inputs.OptionAPropertiesArgs
* {
* Mtu = 5892,
* PeerASN = 42666,
* PrimaryIpv4Prefix = "20.0.0.12/30",
* PrimaryIpv6Prefix = "3FFE:FFFF:0:CD30::a8/126",
* SecondaryIpv4Prefix = "20.0.0.13/30",
* SecondaryIpv6Prefix = "3FFE:FFFF:0:CD30::ac/126",
* VlanId = 2724,
* },
* OptionBProperties = new AzureNative.ManagedNetworkFabric.Inputs.FabricOptionBPropertiesArgs
* {
* ExportRouteTargets = new[]
* {
* "65046:10039",
* },
* ImportRouteTargets = new[]
* {
* "65046:10039",
* },
* },
* PeeringOption = AzureNative.ManagedNetworkFabric.PeeringOption.OptionA,
* },
* WorkloadVpnConfiguration = new AzureNative.ManagedNetworkFabric.Inputs.VpnConfigurationPropertiesArgs
* {
* OptionAProperties = new AzureNative.ManagedNetworkFabric.Inputs.OptionAPropertiesArgs
* {
* Mtu = 5892,
* PeerASN = 42666,
* PrimaryIpv4Prefix = "10.0.0.14/30",
* PrimaryIpv6Prefix = "2FFE:FFFF:0:CD30::a7/126",
* SecondaryIpv4Prefix = "10.0.0.15/30",
* SecondaryIpv6Prefix = "2FFE:FFFF:0:CD30::ac/126",
* VlanId = 2724,
* },
* OptionBProperties = new AzureNative.ManagedNetworkFabric.Inputs.FabricOptionBPropertiesArgs
* {
* ExportRouteTargets = new[]
* {
* "65046:10050",
* },
* ImportRouteTargets = new[]
* {
* "65046:10050",
* },
* },
* PeeringOption = AzureNative.ManagedNetworkFabric.PeeringOption.OptionA,
* },
* },
* NetworkFabricControllerId = "/subscriptions/subscriptionId/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabricControllers/fabricControllerName",
* NetworkFabricName = "FabricName",
* NetworkFabricSku = "M4-A400-A100-C16-aa",
* RackCount = 4,
* ResourceGroupName = "resourceGroupName",
* ServerCountPerRack = 8,
* Tags =
* {
* { "key6468", "" },
* },
* TerminalServerConfiguration = new AzureNative.ManagedNetworkFabric.Inputs.TerminalServerConfigurationArgs
* {
* Password = "xxxx",
* PrimaryIpv4Prefix = "20.0.0.12/30",
* PrimaryIpv6Prefix = "3FFE:FFFF:0:CD30::a8/126",
* SecondaryIpv4Prefix = "20.0.0.13/30",
* SecondaryIpv6Prefix = "3FFE:FFFF:0:CD30::ac/126",
* SerialNumber = "123456",
* Username = "username",
* },
* });
* });
* ```
* ```go
* package main
* import (
* managednetworkfabric "github.com/pulumi/pulumi-azure-native-sdk/managednetworkfabric/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := managednetworkfabric.NewNetworkFabric(ctx, "networkFabric", &managednetworkfabric.NetworkFabricArgs{
* Annotation: pulumi.String("annotationValue"),
* FabricASN: pulumi.Int(29249),
* Ipv4Prefix: pulumi.String("10.18.0.0/19"),
* Ipv6Prefix: pulumi.String("3FFE:FFFF:0:CD40::/59"),
* Location: pulumi.String("eastuseuap"),
* ManagementNetworkConfiguration: &managednetworkfabric.ManagementNetworkConfigurationArgs{
* InfrastructureVpnConfiguration: &managednetworkfabric.VpnConfigurationPropertiesArgs{
* OptionAProperties: &managednetworkfabric.OptionAPropertiesArgs{
* Mtu: pulumi.Int(5892),
* PeerASN: pulumi.Int(42666),
* PrimaryIpv4Prefix: pulumi.String("20.0.0.12/30"),
* PrimaryIpv6Prefix: pulumi.String("3FFE:FFFF:0:CD30::a8/126"),
* SecondaryIpv4Prefix: pulumi.String("20.0.0.13/30"),
* SecondaryIpv6Prefix: pulumi.String("3FFE:FFFF:0:CD30::ac/126"),
* VlanId: pulumi.Int(2724),
* },
* OptionBProperties: &managednetworkfabric.FabricOptionBPropertiesArgs{
* ExportRouteTargets: pulumi.StringArray{
* pulumi.String("65046:10039"),
* },
* ImportRouteTargets: pulumi.StringArray{
* pulumi.String("65046:10039"),
* },
* },
* PeeringOption: pulumi.String(managednetworkfabric.PeeringOptionOptionA),
* },
* WorkloadVpnConfiguration: &managednetworkfabric.VpnConfigurationPropertiesArgs{
* OptionAProperties: &managednetworkfabric.OptionAPropertiesArgs{
* Mtu: pulumi.Int(5892),
* PeerASN: pulumi.Int(42666),
* PrimaryIpv4Prefix: pulumi.String("10.0.0.14/30"),
* PrimaryIpv6Prefix: pulumi.String("2FFE:FFFF:0:CD30::a7/126"),
* SecondaryIpv4Prefix: pulumi.String("10.0.0.15/30"),
* SecondaryIpv6Prefix: pulumi.String("2FFE:FFFF:0:CD30::ac/126"),
* VlanId: pulumi.Int(2724),
* },
* OptionBProperties: &managednetworkfabric.FabricOptionBPropertiesArgs{
* ExportRouteTargets: pulumi.StringArray{
* pulumi.String("65046:10050"),
* },
* ImportRouteTargets: pulumi.StringArray{
* pulumi.String("65046:10050"),
* },
* },
* PeeringOption: pulumi.String(managednetworkfabric.PeeringOptionOptionA),
* },
* },
* NetworkFabricControllerId: pulumi.String("/subscriptions/subscriptionId/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabricControllers/fabricControllerName"),
* NetworkFabricName: pulumi.String("FabricName"),
* NetworkFabricSku: pulumi.String("M4-A400-A100-C16-aa"),
* RackCount: pulumi.Int(4),
* ResourceGroupName: pulumi.String("resourceGroupName"),
* ServerCountPerRack: pulumi.Int(8),
* Tags: pulumi.StringMap{
* "key6468": pulumi.String(""),
* },
* TerminalServerConfiguration: &managednetworkfabric.TerminalServerConfigurationArgs{
* Password: pulumi.String("xxxx"),
* PrimaryIpv4Prefix: pulumi.String("20.0.0.12/30"),
* PrimaryIpv6Prefix: pulumi.String("3FFE:FFFF:0:CD30::a8/126"),
* SecondaryIpv4Prefix: pulumi.String("20.0.0.13/30"),
* SecondaryIpv6Prefix: pulumi.String("3FFE:FFFF:0:CD30::ac/126"),
* SerialNumber: pulumi.String("123456"),
* Username: pulumi.String("username"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.managednetworkfabric.NetworkFabric;
* import com.pulumi.azurenative.managednetworkfabric.NetworkFabricArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.ManagementNetworkConfigurationArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.VpnConfigurationPropertiesArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.OptionAPropertiesArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.FabricOptionBPropertiesArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.TerminalServerConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var networkFabric = new NetworkFabric("networkFabric", NetworkFabricArgs.builder()
* .annotation("annotationValue")
* .fabricASN(29249)
* .ipv4Prefix("10.18.0.0/19")
* .ipv6Prefix("3FFE:FFFF:0:CD40::/59")
* .location("eastuseuap")
* .managementNetworkConfiguration(ManagementNetworkConfigurationArgs.builder()
* .infrastructureVpnConfiguration(VpnConfigurationPropertiesArgs.builder()
* .optionAProperties(OptionAPropertiesArgs.builder()
* .mtu(5892)
* .peerASN(42666)
* .primaryIpv4Prefix("20.0.0.12/30")
* .primaryIpv6Prefix("3FFE:FFFF:0:CD30::a8/126")
* .secondaryIpv4Prefix("20.0.0.13/30")
* .secondaryIpv6Prefix("3FFE:FFFF:0:CD30::ac/126")
* .vlanId(2724)
* .build())
* .optionBProperties(FabricOptionBPropertiesArgs.builder()
* .exportRouteTargets("65046:10039")
* .importRouteTargets("65046:10039")
* .build())
* .peeringOption("OptionA")
* .build())
* .workloadVpnConfiguration(VpnConfigurationPropertiesArgs.builder()
* .optionAProperties(OptionAPropertiesArgs.builder()
* .mtu(5892)
* .peerASN(42666)
* .primaryIpv4Prefix("10.0.0.14/30")
* .primaryIpv6Prefix("2FFE:FFFF:0:CD30::a7/126")
* .secondaryIpv4Prefix("10.0.0.15/30")
* .secondaryIpv6Prefix("2FFE:FFFF:0:CD30::ac/126")
* .vlanId(2724)
* .build())
* .optionBProperties(FabricOptionBPropertiesArgs.builder()
* .exportRouteTargets("65046:10050")
* .importRouteTargets("65046:10050")
* .build())
* .peeringOption("OptionA")
* .build())
* .build())
* .networkFabricControllerId("/subscriptions/subscriptionId/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabricControllers/fabricControllerName")
* .networkFabricName("FabricName")
* .networkFabricSku("M4-A400-A100-C16-aa")
* .rackCount(4)
* .resourceGroupName("resourceGroupName")
* .serverCountPerRack(8)
* .tags(Map.of("key6468", ""))
* .terminalServerConfiguration(TerminalServerConfigurationArgs.builder()
* .password("xxxx")
* .primaryIpv4Prefix("20.0.0.12/30")
* .primaryIpv6Prefix("3FFE:FFFF:0:CD30::a8/126")
* .secondaryIpv4Prefix("20.0.0.13/30")
* .secondaryIpv6Prefix("3FFE:FFFF:0:CD30::ac/126")
* .serialNumber("123456")
* .username("username")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:managednetworkfabric:NetworkFabric FabricName /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedNetworkFabric/networkFabrics/{networkFabricName}
* ```
* @property annotation Switch configuration description.
* @property fabricASN ASN of CE devices for CE/PE connectivity.
* @property ipv4Prefix IPv4Prefix for Management Network. Example: 10.1.0.0/19.
* @property ipv6Prefix IPv6Prefix for Management Network. Example: 3FFE:FFFF:0:CD40::/59.
* @property location The geo-location where the resource lives
* @property managementNetworkConfiguration Configuration to be used to setup the management network.
* @property networkFabricControllerId Azure resource ID for the NetworkFabricController the NetworkFabric belongs.
* @property networkFabricName Name of the Network Fabric
* @property networkFabricSku Supported Network Fabric SKU.Example: Compute / Aggregate racks. Once the user chooses a particular SKU, only supported racks can be added to the Network Fabric. The SKU determines whether it is a single / multi rack Network Fabric.
* @property rackCount Number of racks associated to Network Fabric.Possible values are from 2-8.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property serverCountPerRack Number of servers.Possible values are from 1-16.
* @property tags Resource tags.
* @property terminalServerConfiguration Network and credentials configuration currently applied to terminal server.
*/
public data class NetworkFabricArgs(
public val `annotation`: Output? = null,
public val fabricASN: Output? = null,
public val ipv4Prefix: Output? = null,
public val ipv6Prefix: Output? = null,
public val location: Output? = null,
public val managementNetworkConfiguration: Output? = null,
public val networkFabricControllerId: Output? = null,
public val networkFabricName: Output? = null,
public val networkFabricSku: Output? = null,
public val rackCount: Output? = null,
public val resourceGroupName: Output? = null,
public val serverCountPerRack: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy