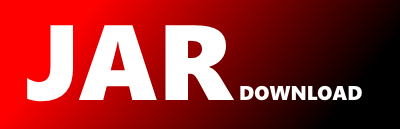
com.pulumi.azurenative.managednetworkfabric.kotlin.NetworkTapRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.managednetworkfabric.kotlin
import com.pulumi.azurenative.managednetworkfabric.NetworkTapRuleArgs.builder
import com.pulumi.azurenative.managednetworkfabric.kotlin.enums.ConfigurationType
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.CommonDynamicMatchConfigurationArgs
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.CommonDynamicMatchConfigurationArgsBuilder
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.NetworkTapRuleMatchConfigurationArgs
import com.pulumi.azurenative.managednetworkfabric.kotlin.inputs.NetworkTapRuleMatchConfigurationArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The NetworkTapRule resource definition.
* Azure REST API version: 2023-06-15.
* ## Example Usage
* ### NetworkTapRules_Create_MaximumSet_Gen
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var networkTapRule = new AzureNative.ManagedNetworkFabric.NetworkTapRule("networkTapRule", new()
* {
* Annotation = "annotation",
* ConfigurationType = AzureNative.ManagedNetworkFabric.ConfigurationType.File,
* DynamicMatchConfigurations = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.CommonDynamicMatchConfigurationArgs
* {
* IpGroups = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.IpGroupPropertiesArgs
* {
* IpAddressType = AzureNative.ManagedNetworkFabric.IPAddressType.IPv4,
* IpPrefixes = new[]
* {
* "10.10.10.10/30",
* },
* Name = "example-ipGroup1",
* },
* },
* PortGroups = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.PortGroupPropertiesArgs
* {
* Name = "example-portGroup1",
* Ports = new[]
* {
* "100-200",
* },
* },
* new AzureNative.ManagedNetworkFabric.Inputs.PortGroupPropertiesArgs
* {
* Name = "example-portGroup2",
* Ports = new[]
* {
* "900",
* "1000-2000",
* },
* },
* },
* VlanGroups = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.VlanGroupPropertiesArgs
* {
* Name = "exmaple-vlanGroup",
* Vlans = new[]
* {
* "10",
* "100-200",
* },
* },
* },
* },
* },
* Location = "eastus",
* MatchConfigurations = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.NetworkTapRuleMatchConfigurationArgs
* {
* Actions = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.NetworkTapRuleActionArgs
* {
* DestinationId = "/subscriptions/1234ABCD-0A1B-1234-5678-123456ABCDEF/resourcegroups/example-rg/providers/Microsoft.ManagedNetworkFabric/neighborGroups/example-neighborGroup",
* IsTimestampEnabled = AzureNative.ManagedNetworkFabric.BooleanEnumProperty.True,
* MatchConfigurationName = "match1",
* Truncate = "100",
* Type = AzureNative.ManagedNetworkFabric.TapRuleActionType.Drop,
* },
* },
* IpAddressType = AzureNative.ManagedNetworkFabric.IPAddressType.IPv4,
* MatchConditions = new[]
* {
* new AzureNative.ManagedNetworkFabric.Inputs.NetworkTapRuleMatchConditionArgs
* {
* EncapsulationType = AzureNative.ManagedNetworkFabric.EncapsulationType.None,
* IpCondition = new AzureNative.ManagedNetworkFabric.Inputs.IpMatchConditionArgs
* {
* IpGroupNames = new[]
* {
* "example-ipGroup",
* },
* IpPrefixValues = new[]
* {
* "10.10.10.10/20",
* },
* PrefixType = AzureNative.ManagedNetworkFabric.PrefixType.Prefix,
* Type = AzureNative.ManagedNetworkFabric.SourceDestinationType.SourceIP,
* },
* PortCondition = new AzureNative.ManagedNetworkFabric.Inputs.PortConditionArgs
* {
* Layer4Protocol = AzureNative.ManagedNetworkFabric.Layer4Protocol.TCP,
* PortGroupNames = new[]
* {
* "example-portGroup1",
* },
* PortType = AzureNative.ManagedNetworkFabric.PortType.SourcePort,
* Ports = new[]
* {
* "100",
* },
* },
* ProtocolTypes = new[]
* {
* "TCP",
* },
* VlanMatchCondition = new AzureNative.ManagedNetworkFabric.Inputs.VlanMatchConditionArgs
* {
* InnerVlans = new[]
* {
* "11-20",
* },
* VlanGroupNames = new[]
* {
* "exmaple-vlanGroup",
* },
* Vlans = new[]
* {
* "10",
* },
* },
* },
* },
* MatchConfigurationName = "config1",
* SequenceNumber = 10,
* },
* },
* NetworkTapRuleName = "example-tapRule",
* PollingIntervalInSeconds = 30,
* ResourceGroupName = "example-rg",
* Tags =
* {
* { "keyID", "keyValue" },
* },
* TapRulesUrl = "https://microsoft.com/a",
* });
* });
* ```
* ```go
* package main
* import (
* managednetworkfabric "github.com/pulumi/pulumi-azure-native-sdk/managednetworkfabric/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := managednetworkfabric.NewNetworkTapRule(ctx, "networkTapRule", &managednetworkfabric.NetworkTapRuleArgs{
* Annotation: pulumi.String("annotation"),
* ConfigurationType: pulumi.String(managednetworkfabric.ConfigurationTypeFile),
* DynamicMatchConfigurations: managednetworkfabric.CommonDynamicMatchConfigurationArray{
* &managednetworkfabric.CommonDynamicMatchConfigurationArgs{
* IpGroups: managednetworkfabric.IpGroupPropertiesArray{
* &managednetworkfabric.IpGroupPropertiesArgs{
* IpAddressType: pulumi.String(managednetworkfabric.IPAddressTypeIPv4),
* IpPrefixes: pulumi.StringArray{
* pulumi.String("10.10.10.10/30"),
* },
* Name: pulumi.String("example-ipGroup1"),
* },
* },
* PortGroups: managednetworkfabric.PortGroupPropertiesArray{
* &managednetworkfabric.PortGroupPropertiesArgs{
* Name: pulumi.String("example-portGroup1"),
* Ports: pulumi.StringArray{
* pulumi.String("100-200"),
* },
* },
* &managednetworkfabric.PortGroupPropertiesArgs{
* Name: pulumi.String("example-portGroup2"),
* Ports: pulumi.StringArray{
* pulumi.String("900"),
* pulumi.String("1000-2000"),
* },
* },
* },
* VlanGroups: managednetworkfabric.VlanGroupPropertiesArray{
* &managednetworkfabric.VlanGroupPropertiesArgs{
* Name: pulumi.String("exmaple-vlanGroup"),
* Vlans: pulumi.StringArray{
* pulumi.String("10"),
* pulumi.String("100-200"),
* },
* },
* },
* },
* },
* Location: pulumi.String("eastus"),
* MatchConfigurations: managednetworkfabric.NetworkTapRuleMatchConfigurationArray{
* &managednetworkfabric.NetworkTapRuleMatchConfigurationArgs{
* Actions: managednetworkfabric.NetworkTapRuleActionArray{
* &managednetworkfabric.NetworkTapRuleActionArgs{
* DestinationId: pulumi.String("/subscriptions/1234ABCD-0A1B-1234-5678-123456ABCDEF/resourcegroups/example-rg/providers/Microsoft.ManagedNetworkFabric/neighborGroups/example-neighborGroup"),
* IsTimestampEnabled: pulumi.String(managednetworkfabric.BooleanEnumPropertyTrue),
* MatchConfigurationName: pulumi.String("match1"),
* Truncate: pulumi.String("100"),
* Type: pulumi.String(managednetworkfabric.TapRuleActionTypeDrop),
* },
* },
* IpAddressType: pulumi.String(managednetworkfabric.IPAddressTypeIPv4),
* MatchConditions: managednetworkfabric.NetworkTapRuleMatchConditionArray{
* &managednetworkfabric.NetworkTapRuleMatchConditionArgs{
* EncapsulationType: pulumi.String(managednetworkfabric.EncapsulationTypeNone),
* IpCondition: &managednetworkfabric.IpMatchConditionArgs{
* IpGroupNames: pulumi.StringArray{
* pulumi.String("example-ipGroup"),
* },
* IpPrefixValues: pulumi.StringArray{
* pulumi.String("10.10.10.10/20"),
* },
* PrefixType: pulumi.String(managednetworkfabric.PrefixTypePrefix),
* Type: pulumi.String(managednetworkfabric.SourceDestinationTypeSourceIP),
* },
* PortCondition: &managednetworkfabric.PortConditionArgs{
* Layer4Protocol: pulumi.String(managednetworkfabric.Layer4ProtocolTCP),
* PortGroupNames: pulumi.StringArray{
* pulumi.String("example-portGroup1"),
* },
* PortType: pulumi.String(managednetworkfabric.PortTypeSourcePort),
* Ports: pulumi.StringArray{
* pulumi.String("100"),
* },
* },
* ProtocolTypes: pulumi.StringArray{
* pulumi.String("TCP"),
* },
* VlanMatchCondition: &managednetworkfabric.VlanMatchConditionArgs{
* InnerVlans: pulumi.StringArray{
* pulumi.String("11-20"),
* },
* VlanGroupNames: pulumi.StringArray{
* pulumi.String("exmaple-vlanGroup"),
* },
* Vlans: pulumi.StringArray{
* pulumi.String("10"),
* },
* },
* },
* },
* MatchConfigurationName: pulumi.String("config1"),
* SequenceNumber: pulumi.Float64(10),
* },
* },
* NetworkTapRuleName: pulumi.String("example-tapRule"),
* PollingIntervalInSeconds: pulumi.Int(30),
* ResourceGroupName: pulumi.String("example-rg"),
* Tags: pulumi.StringMap{
* "keyID": pulumi.String("keyValue"),
* },
* TapRulesUrl: pulumi.String("https://microsoft.com/a"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.managednetworkfabric.NetworkTapRule;
* import com.pulumi.azurenative.managednetworkfabric.NetworkTapRuleArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.CommonDynamicMatchConfigurationArgs;
* import com.pulumi.azurenative.managednetworkfabric.inputs.NetworkTapRuleMatchConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var networkTapRule = new NetworkTapRule("networkTapRule", NetworkTapRuleArgs.builder()
* .annotation("annotation")
* .configurationType("File")
* .dynamicMatchConfigurations(CommonDynamicMatchConfigurationArgs.builder()
* .ipGroups(IpGroupPropertiesArgs.builder()
* .ipAddressType("IPv4")
* .ipPrefixes("10.10.10.10/30")
* .name("example-ipGroup1")
* .build())
* .portGroups(
* PortGroupPropertiesArgs.builder()
* .name("example-portGroup1")
* .ports("100-200")
* .build(),
* PortGroupPropertiesArgs.builder()
* .name("example-portGroup2")
* .ports(
* "900",
* "1000-2000")
* .build())
* .vlanGroups(VlanGroupPropertiesArgs.builder()
* .name("exmaple-vlanGroup")
* .vlans(
* "10",
* "100-200")
* .build())
* .build())
* .location("eastus")
* .matchConfigurations(NetworkTapRuleMatchConfigurationArgs.builder()
* .actions(NetworkTapRuleActionArgs.builder()
* .destinationId("/subscriptions/1234ABCD-0A1B-1234-5678-123456ABCDEF/resourcegroups/example-rg/providers/Microsoft.ManagedNetworkFabric/neighborGroups/example-neighborGroup")
* .isTimestampEnabled("True")
* .matchConfigurationName("match1")
* .truncate("100")
* .type("Drop")
* .build())
* .ipAddressType("IPv4")
* .matchConditions(NetworkTapRuleMatchConditionArgs.builder()
* .encapsulationType("None")
* .ipCondition(IpMatchConditionArgs.builder()
* .ipGroupNames("example-ipGroup")
* .ipPrefixValues("10.10.10.10/20")
* .prefixType("Prefix")
* .type("SourceIP")
* .build())
* .portCondition(PortConditionArgs.builder()
* .layer4Protocol("TCP")
* .portGroupNames("example-portGroup1")
* .portType("SourcePort")
* .ports("100")
* .build())
* .protocolTypes("TCP")
* .vlanMatchCondition(VlanMatchConditionArgs.builder()
* .innerVlans("11-20")
* .vlanGroupNames("exmaple-vlanGroup")
* .vlans("10")
* .build())
* .build())
* .matchConfigurationName("config1")
* .sequenceNumber(10)
* .build())
* .networkTapRuleName("example-tapRule")
* .pollingIntervalInSeconds(30)
* .resourceGroupName("example-rg")
* .tags(Map.of("keyID", "keyValue"))
* .tapRulesUrl("https://microsoft.com/a")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:managednetworkfabric:NetworkTapRule example-tapRule /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedNetworkFabric/networkTapRules/{networkTapRuleName}
* ```
* @property annotation Switch configuration description.
* @property configurationType Input method to configure Network Tap Rule.
* @property dynamicMatchConfigurations List of dynamic match configurations.
* @property location The geo-location where the resource lives
* @property matchConfigurations List of match configurations.
* @property networkTapRuleName Name of the Network Tap Rule.
* @property pollingIntervalInSeconds Polling interval in seconds.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property tags Resource tags.
* @property tapRulesUrl Network Tap Rules file URL.
*/
public data class NetworkTapRuleArgs(
public val `annotation`: Output? = null,
public val configurationType: Output>? = null,
public val dynamicMatchConfigurations: Output>? = null,
public val location: Output? = null,
public val matchConfigurations: Output>? = null,
public val networkTapRuleName: Output? = null,
public val pollingIntervalInSeconds: Output? = null,
public val resourceGroupName: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy