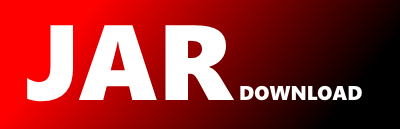
com.pulumi.azurenative.managedservices.kotlin.inputs.AuthorizationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.managedservices.kotlin.inputs
import com.pulumi.azurenative.managedservices.inputs.AuthorizationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The Azure Active Directory principal identifier and Azure built-in role that describes the access the principal will receive on the delegated resource in the managed tenant.
* @property delegatedRoleDefinitionIds The delegatedRoleDefinitionIds field is required when the roleDefinitionId refers to the User Access Administrator Role. It is the list of role definition ids which define all the permissions that the user in the authorization can assign to other principals.
* @property principalId The identifier of the Azure Active Directory principal.
* @property principalIdDisplayName The display name of the Azure Active Directory principal.
* @property roleDefinitionId The identifier of the Azure built-in role that defines the permissions that the Azure Active Directory principal will have on the projected scope.
*/
public data class AuthorizationArgs(
public val delegatedRoleDefinitionIds: Output>? = null,
public val principalId: Output,
public val principalIdDisplayName: Output? = null,
public val roleDefinitionId: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.managedservices.inputs.AuthorizationArgs =
com.pulumi.azurenative.managedservices.inputs.AuthorizationArgs.builder()
.delegatedRoleDefinitionIds(
delegatedRoleDefinitionIds?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.principalId(principalId.applyValue({ args0 -> args0 }))
.principalIdDisplayName(principalIdDisplayName?.applyValue({ args0 -> args0 }))
.roleDefinitionId(roleDefinitionId.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AuthorizationArgs].
*/
@PulumiTagMarker
public class AuthorizationArgsBuilder internal constructor() {
private var delegatedRoleDefinitionIds: Output>? = null
private var principalId: Output? = null
private var principalIdDisplayName: Output? = null
private var roleDefinitionId: Output? = null
/**
* @param value The delegatedRoleDefinitionIds field is required when the roleDefinitionId refers to the User Access Administrator Role. It is the list of role definition ids which define all the permissions that the user in the authorization can assign to other principals.
*/
@JvmName("konvkqgnxxqckoxs")
public suspend fun delegatedRoleDefinitionIds(`value`: Output>) {
this.delegatedRoleDefinitionIds = value
}
@JvmName("mlmekaniwfbkhwse")
public suspend fun delegatedRoleDefinitionIds(vararg values: Output) {
this.delegatedRoleDefinitionIds = Output.all(values.asList())
}
/**
* @param values The delegatedRoleDefinitionIds field is required when the roleDefinitionId refers to the User Access Administrator Role. It is the list of role definition ids which define all the permissions that the user in the authorization can assign to other principals.
*/
@JvmName("bawyndwgwqaonyrm")
public suspend fun delegatedRoleDefinitionIds(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy