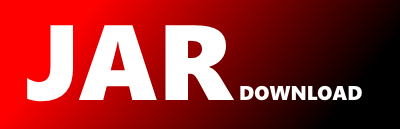
com.pulumi.azurenative.media.kotlin.LiveOutputArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin
import com.pulumi.azurenative.media.LiveOutputArgs.builder
import com.pulumi.azurenative.media.kotlin.inputs.HlsArgs
import com.pulumi.azurenative.media.kotlin.inputs.HlsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The Live Output.
* Azure REST API version: 2022-11-01. Prior API version in Azure Native 1.x: 2020-05-01.
* ## Example Usage
* ### Create a LiveOutput
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var liveOutput = new AzureNative.Media.LiveOutput("liveOutput", new()
* {
* AccountName = "slitestmedia10",
* ArchiveWindowLength = "PT5M",
* AssetName = "6f3264f5-a189-48b4-a29a-a40f22575212",
* Description = "test live output 1",
* Hls = new AzureNative.Media.Inputs.HlsArgs
* {
* FragmentsPerTsSegment = 5,
* },
* LiveEventName = "myLiveEvent1",
* LiveOutputName = "myLiveOutput1",
* ManifestName = "testmanifest",
* ResourceGroupName = "mediaresources",
* RewindWindowLength = "PT4M",
* });
* });
* ```
* ```go
* package main
* import (
* media "github.com/pulumi/pulumi-azure-native-sdk/media/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := media.NewLiveOutput(ctx, "liveOutput", &media.LiveOutputArgs{
* AccountName: pulumi.String("slitestmedia10"),
* ArchiveWindowLength: pulumi.String("PT5M"),
* AssetName: pulumi.String("6f3264f5-a189-48b4-a29a-a40f22575212"),
* Description: pulumi.String("test live output 1"),
* Hls: &media.HlsArgs{
* FragmentsPerTsSegment: pulumi.Int(5),
* },
* LiveEventName: pulumi.String("myLiveEvent1"),
* LiveOutputName: pulumi.String("myLiveOutput1"),
* ManifestName: pulumi.String("testmanifest"),
* ResourceGroupName: pulumi.String("mediaresources"),
* RewindWindowLength: pulumi.String("PT4M"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.media.LiveOutput;
* import com.pulumi.azurenative.media.LiveOutputArgs;
* import com.pulumi.azurenative.media.inputs.HlsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var liveOutput = new LiveOutput("liveOutput", LiveOutputArgs.builder()
* .accountName("slitestmedia10")
* .archiveWindowLength("PT5M")
* .assetName("6f3264f5-a189-48b4-a29a-a40f22575212")
* .description("test live output 1")
* .hls(HlsArgs.builder()
* .fragmentsPerTsSegment(5)
* .build())
* .liveEventName("myLiveEvent1")
* .liveOutputName("myLiveOutput1")
* .manifestName("testmanifest")
* .resourceGroupName("mediaresources")
* .rewindWindowLength("PT4M")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:media:LiveOutput myLiveOutput1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Media/mediaservices/{accountName}/liveEvents/{liveEventName}/liveOutputs/{liveOutputName}
* ```
* @property accountName The Media Services account name.
* @property archiveWindowLength ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
* @property assetName The asset that the live output will write to.
* @property description The description of the live output.
* @property hls HTTP Live Streaming (HLS) packing setting for the live output.
* @property liveEventName The name of the live event, maximum length is 32.
* @property liveOutputName The name of the live output.
* @property manifestName The manifest file name. If not provided, the service will generate one automatically.
* @property outputSnapTime The initial timestamp that the live output will start at, any content before this value will not be archived.
* @property resourceGroupName The name of the resource group within the Azure subscription.
* @property rewindWindowLength ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*/
public data class LiveOutputArgs(
public val accountName: Output? = null,
public val archiveWindowLength: Output? = null,
public val assetName: Output? = null,
public val description: Output? = null,
public val hls: Output? = null,
public val liveEventName: Output? = null,
public val liveOutputName: Output? = null,
public val manifestName: Output? = null,
public val outputSnapTime: Output? = null,
public val resourceGroupName: Output? = null,
public val rewindWindowLength: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.LiveOutputArgs =
com.pulumi.azurenative.media.LiveOutputArgs.builder()
.accountName(accountName?.applyValue({ args0 -> args0 }))
.archiveWindowLength(archiveWindowLength?.applyValue({ args0 -> args0 }))
.assetName(assetName?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.hls(hls?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.liveEventName(liveEventName?.applyValue({ args0 -> args0 }))
.liveOutputName(liveOutputName?.applyValue({ args0 -> args0 }))
.manifestName(manifestName?.applyValue({ args0 -> args0 }))
.outputSnapTime(outputSnapTime?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.rewindWindowLength(rewindWindowLength?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LiveOutputArgs].
*/
@PulumiTagMarker
public class LiveOutputArgsBuilder internal constructor() {
private var accountName: Output? = null
private var archiveWindowLength: Output? = null
private var assetName: Output? = null
private var description: Output? = null
private var hls: Output? = null
private var liveEventName: Output? = null
private var liveOutputName: Output? = null
private var manifestName: Output? = null
private var outputSnapTime: Output? = null
private var resourceGroupName: Output? = null
private var rewindWindowLength: Output? = null
/**
* @param value The Media Services account name.
*/
@JvmName("htewutgohvgylxnl")
public suspend fun accountName(`value`: Output) {
this.accountName = value
}
/**
* @param value ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*/
@JvmName("nepdtwagdbtvxbbc")
public suspend fun archiveWindowLength(`value`: Output) {
this.archiveWindowLength = value
}
/**
* @param value The asset that the live output will write to.
*/
@JvmName("jurffrexoydhckms")
public suspend fun assetName(`value`: Output) {
this.assetName = value
}
/**
* @param value The description of the live output.
*/
@JvmName("srjlaxsawixrynoh")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value HTTP Live Streaming (HLS) packing setting for the live output.
*/
@JvmName("nrmmqtfdwscpniwk")
public suspend fun hls(`value`: Output) {
this.hls = value
}
/**
* @param value The name of the live event, maximum length is 32.
*/
@JvmName("shdkrklkrxvvocbt")
public suspend fun liveEventName(`value`: Output) {
this.liveEventName = value
}
/**
* @param value The name of the live output.
*/
@JvmName("kkohpdxnqiulxvdo")
public suspend fun liveOutputName(`value`: Output) {
this.liveOutputName = value
}
/**
* @param value The manifest file name. If not provided, the service will generate one automatically.
*/
@JvmName("puuobfiopxealijn")
public suspend fun manifestName(`value`: Output) {
this.manifestName = value
}
/**
* @param value The initial timestamp that the live output will start at, any content before this value will not be archived.
*/
@JvmName("tlhcxpixabextvtp")
public suspend fun outputSnapTime(`value`: Output) {
this.outputSnapTime = value
}
/**
* @param value The name of the resource group within the Azure subscription.
*/
@JvmName("uchbpqsjeperaqqh")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*/
@JvmName("cwusfxxmnmawgtkt")
public suspend fun rewindWindowLength(`value`: Output) {
this.rewindWindowLength = value
}
/**
* @param value The Media Services account name.
*/
@JvmName("evhpwanqwkpbwvwp")
public suspend fun accountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accountName = mapped
}
/**
* @param value ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*/
@JvmName("wojyfncwqfkcffwn")
public suspend fun archiveWindowLength(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.archiveWindowLength = mapped
}
/**
* @param value The asset that the live output will write to.
*/
@JvmName("rmirrohysjgakonr")
public suspend fun assetName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.assetName = mapped
}
/**
* @param value The description of the live output.
*/
@JvmName("temasdcjdathhyxq")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value HTTP Live Streaming (HLS) packing setting for the live output.
*/
@JvmName("plosoedthiwxmqtn")
public suspend fun hls(`value`: HlsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hls = mapped
}
/**
* @param argument HTTP Live Streaming (HLS) packing setting for the live output.
*/
@JvmName("mwahbxtlcsxyorgt")
public suspend fun hls(argument: suspend HlsArgsBuilder.() -> Unit) {
val toBeMapped = HlsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.hls = mapped
}
/**
* @param value The name of the live event, maximum length is 32.
*/
@JvmName("ryyllyngqtaawssy")
public suspend fun liveEventName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.liveEventName = mapped
}
/**
* @param value The name of the live output.
*/
@JvmName("hpjbouxjioiwpvtc")
public suspend fun liveOutputName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.liveOutputName = mapped
}
/**
* @param value The manifest file name. If not provided, the service will generate one automatically.
*/
@JvmName("avjevdtemwanggpd")
public suspend fun manifestName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.manifestName = mapped
}
/**
* @param value The initial timestamp that the live output will start at, any content before this value will not be archived.
*/
@JvmName("tfrbhqmemlbshxhw")
public suspend fun outputSnapTime(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outputSnapTime = mapped
}
/**
* @param value The name of the resource group within the Azure subscription.
*/
@JvmName("hcnrhsakrspmvogj")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*/
@JvmName("xeqqwmsvsrhfqamc")
public suspend fun rewindWindowLength(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rewindWindowLength = mapped
}
internal fun build(): LiveOutputArgs = LiveOutputArgs(
accountName = accountName,
archiveWindowLength = archiveWindowLength,
assetName = assetName,
description = description,
hls = hls,
liveEventName = liveEventName,
liveOutputName = liveOutputName,
manifestName = manifestName,
outputSnapTime = outputSnapTime,
resourceGroupName = resourceGroupName,
rewindWindowLength = rewindWindowLength,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy