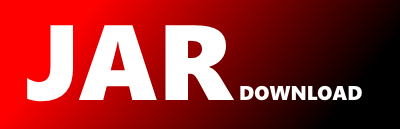
com.pulumi.azurenative.media.kotlin.inputs.AudioTrackArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin.inputs
import com.pulumi.azurenative.media.inputs.AudioTrackArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Represents an audio track in the asset.
* @property dashSettings The DASH specific setting for the audio track.
* @property displayName The display name of the audio track on a video player. In HLS, this maps to the NAME attribute of EXT-X-MEDIA.
* @property fileName The file name to the source file. This file is located in the storage container of the asset.
* @property hlsSettings The HLS specific setting for the audio track.
* @property languageCode The RFC5646 language code for the audio track.
* @property mpeg4TrackId The MPEG-4 audio track ID for the audio track.
* @property odataType The discriminator for derived types.
* Expected value is '#Microsoft.Media.AudioTrack'.
*/
public data class AudioTrackArgs(
public val dashSettings: Output? = null,
public val displayName: Output? = null,
public val fileName: Output? = null,
public val hlsSettings: Output? = null,
public val languageCode: Output? = null,
public val mpeg4TrackId: Output? = null,
public val odataType: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.inputs.AudioTrackArgs =
com.pulumi.azurenative.media.inputs.AudioTrackArgs.builder()
.dashSettings(dashSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.fileName(fileName?.applyValue({ args0 -> args0 }))
.hlsSettings(hlsSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.languageCode(languageCode?.applyValue({ args0 -> args0 }))
.mpeg4TrackId(mpeg4TrackId?.applyValue({ args0 -> args0 }))
.odataType(odataType.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AudioTrackArgs].
*/
@PulumiTagMarker
public class AudioTrackArgsBuilder internal constructor() {
private var dashSettings: Output? = null
private var displayName: Output? = null
private var fileName: Output? = null
private var hlsSettings: Output? = null
private var languageCode: Output? = null
private var mpeg4TrackId: Output? = null
private var odataType: Output? = null
/**
* @param value The DASH specific setting for the audio track.
*/
@JvmName("wxnsqpvbltxfxchb")
public suspend fun dashSettings(`value`: Output) {
this.dashSettings = value
}
/**
* @param value The display name of the audio track on a video player. In HLS, this maps to the NAME attribute of EXT-X-MEDIA.
*/
@JvmName("eqtxritffojrarxn")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The file name to the source file. This file is located in the storage container of the asset.
*/
@JvmName("nyhbbsywvcohcrsv")
public suspend fun fileName(`value`: Output) {
this.fileName = value
}
/**
* @param value The HLS specific setting for the audio track.
*/
@JvmName("wedwmmuobbegerxm")
public suspend fun hlsSettings(`value`: Output) {
this.hlsSettings = value
}
/**
* @param value The RFC5646 language code for the audio track.
*/
@JvmName("gbbelxvhwkecuvnv")
public suspend fun languageCode(`value`: Output) {
this.languageCode = value
}
/**
* @param value The MPEG-4 audio track ID for the audio track.
*/
@JvmName("koaxnkoipnmjnthy")
public suspend fun mpeg4TrackId(`value`: Output) {
this.mpeg4TrackId = value
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.Media.AudioTrack'.
*/
@JvmName("mfhkacsixoeuhava")
public suspend fun odataType(`value`: Output) {
this.odataType = value
}
/**
* @param value The DASH specific setting for the audio track.
*/
@JvmName("mpgqwqudtyxjhetj")
public suspend fun dashSettings(`value`: DashSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dashSettings = mapped
}
/**
* @param argument The DASH specific setting for the audio track.
*/
@JvmName("urcauhbxevjevtvt")
public suspend fun dashSettings(argument: suspend DashSettingsArgsBuilder.() -> Unit) {
val toBeMapped = DashSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.dashSettings = mapped
}
/**
* @param value The display name of the audio track on a video player. In HLS, this maps to the NAME attribute of EXT-X-MEDIA.
*/
@JvmName("yooebbdcduknmqhi")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The file name to the source file. This file is located in the storage container of the asset.
*/
@JvmName("wuwugrwwswrslfak")
public suspend fun fileName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileName = mapped
}
/**
* @param value The HLS specific setting for the audio track.
*/
@JvmName("xrvsrqmjbyvpscxu")
public suspend fun hlsSettings(`value`: HlsSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hlsSettings = mapped
}
/**
* @param argument The HLS specific setting for the audio track.
*/
@JvmName("hpwheurovfdsmynj")
public suspend fun hlsSettings(argument: suspend HlsSettingsArgsBuilder.() -> Unit) {
val toBeMapped = HlsSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.hlsSettings = mapped
}
/**
* @param value The RFC5646 language code for the audio track.
*/
@JvmName("ckiyeqgimgncysqa")
public suspend fun languageCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.languageCode = mapped
}
/**
* @param value The MPEG-4 audio track ID for the audio track.
*/
@JvmName("scxbhfqhqijeumtd")
public suspend fun mpeg4TrackId(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mpeg4TrackId = mapped
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.Media.AudioTrack'.
*/
@JvmName("uyyathxfelgdcpkr")
public suspend fun odataType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.odataType = mapped
}
internal fun build(): AudioTrackArgs = AudioTrackArgs(
dashSettings = dashSettings,
displayName = displayName,
fileName = fileName,
hlsSettings = hlsSettings,
languageCode = languageCode,
mpeg4TrackId = mpeg4TrackId,
odataType = odataType ?: throw PulumiNullFieldException("odataType"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy