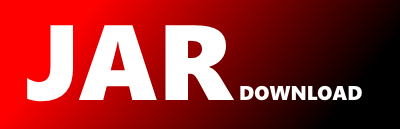
com.pulumi.azurenative.media.kotlin.inputs.EnvelopeEncryptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin.inputs
import com.pulumi.azurenative.media.inputs.EnvelopeEncryptionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Class for EnvelopeEncryption encryption scheme
* @property clearTracks Representing which tracks should not be encrypted
* @property contentKeys Representing default content key for each encryption scheme and separate content keys for specific tracks
* @property customKeyAcquisitionUrlTemplate Template for the URL of the custom service delivering keys to end user players. Not required when using Azure Media Services for issuing keys. The template supports replaceable tokens that the service will update at runtime with the value specific to the request. The currently supported token values are {AlternativeMediaId}, which is replaced with the value of StreamingLocatorId.AlternativeMediaId, and {ContentKeyId}, which is replaced with the value of identifier of the key being requested.
* @property enabledProtocols Representing supported protocols
*/
public data class EnvelopeEncryptionArgs(
public val clearTracks: Output>? = null,
public val contentKeys: Output? = null,
public val customKeyAcquisitionUrlTemplate: Output? = null,
public val enabledProtocols: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.inputs.EnvelopeEncryptionArgs =
com.pulumi.azurenative.media.inputs.EnvelopeEncryptionArgs.builder()
.clearTracks(
clearTracks?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.contentKeys(contentKeys?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.customKeyAcquisitionUrlTemplate(customKeyAcquisitionUrlTemplate?.applyValue({ args0 -> args0 }))
.enabledProtocols(
enabledProtocols?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [EnvelopeEncryptionArgs].
*/
@PulumiTagMarker
public class EnvelopeEncryptionArgsBuilder internal constructor() {
private var clearTracks: Output>? = null
private var contentKeys: Output? = null
private var customKeyAcquisitionUrlTemplate: Output? = null
private var enabledProtocols: Output? = null
/**
* @param value Representing which tracks should not be encrypted
*/
@JvmName("poocrnrfxmrcwppp")
public suspend fun clearTracks(`value`: Output>) {
this.clearTracks = value
}
@JvmName("yltdgfuqcadftcvn")
public suspend fun clearTracks(vararg values: Output) {
this.clearTracks = Output.all(values.asList())
}
/**
* @param values Representing which tracks should not be encrypted
*/
@JvmName("dbsapavkrsbbsqek")
public suspend fun clearTracks(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy