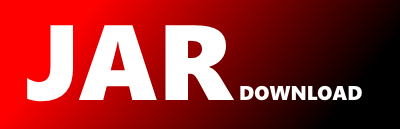
com.pulumi.azurenative.media.kotlin.inputs.JobOutputAssetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin.inputs
import com.pulumi.azurenative.media.inputs.JobOutputAssetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Represents an Asset used as a JobOutput.
* @property assetName The name of the output Asset.
* @property label A label that is assigned to a JobOutput in order to help uniquely identify it. This is useful when your Transform has more than one TransformOutput, whereby your Job has more than one JobOutput. In such cases, when you submit the Job, you will add two or more JobOutputs, in the same order as TransformOutputs in the Transform. Subsequently, when you retrieve the Job, either through events or on a GET request, you can use the label to easily identify the JobOutput. If a label is not provided, a default value of '{presetName}_{outputIndex}' will be used, where the preset name is the name of the preset in the corresponding TransformOutput and the output index is the relative index of the this JobOutput within the Job. Note that this index is the same as the relative index of the corresponding TransformOutput within its Transform.
* @property odataType The discriminator for derived types.
* Expected value is '#Microsoft.Media.JobOutputAsset'.
* @property presetOverride A preset used to override the preset in the corresponding transform output.
*/
public data class JobOutputAssetArgs(
public val assetName: Output,
public val label: Output? = null,
public val odataType: Output,
public val presetOverride: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.inputs.JobOutputAssetArgs =
com.pulumi.azurenative.media.inputs.JobOutputAssetArgs.builder()
.assetName(assetName.applyValue({ args0 -> args0 }))
.label(label?.applyValue({ args0 -> args0 }))
.odataType(odataType.applyValue({ args0 -> args0 }))
.presetOverride(presetOverride?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JobOutputAssetArgs].
*/
@PulumiTagMarker
public class JobOutputAssetArgsBuilder internal constructor() {
private var assetName: Output? = null
private var label: Output? = null
private var odataType: Output? = null
private var presetOverride: Output? = null
/**
* @param value The name of the output Asset.
*/
@JvmName("ejpkywhvusllanhl")
public suspend fun assetName(`value`: Output) {
this.assetName = value
}
/**
* @param value A label that is assigned to a JobOutput in order to help uniquely identify it. This is useful when your Transform has more than one TransformOutput, whereby your Job has more than one JobOutput. In such cases, when you submit the Job, you will add two or more JobOutputs, in the same order as TransformOutputs in the Transform. Subsequently, when you retrieve the Job, either through events or on a GET request, you can use the label to easily identify the JobOutput. If a label is not provided, a default value of '{presetName}_{outputIndex}' will be used, where the preset name is the name of the preset in the corresponding TransformOutput and the output index is the relative index of the this JobOutput within the Job. Note that this index is the same as the relative index of the corresponding TransformOutput within its Transform.
*/
@JvmName("madsffbfsmuieljq")
public suspend fun label(`value`: Output) {
this.label = value
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.Media.JobOutputAsset'.
*/
@JvmName("dkgtrhawyiqyjutd")
public suspend fun odataType(`value`: Output) {
this.odataType = value
}
/**
* @param value A preset used to override the preset in the corresponding transform output.
*/
@JvmName("tbfmcwwcvhgdhryg")
public suspend fun presetOverride(`value`: Output) {
this.presetOverride = value
}
/**
* @param value The name of the output Asset.
*/
@JvmName("yiyvlosbwgmveruq")
public suspend fun assetName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.assetName = mapped
}
/**
* @param value A label that is assigned to a JobOutput in order to help uniquely identify it. This is useful when your Transform has more than one TransformOutput, whereby your Job has more than one JobOutput. In such cases, when you submit the Job, you will add two or more JobOutputs, in the same order as TransformOutputs in the Transform. Subsequently, when you retrieve the Job, either through events or on a GET request, you can use the label to easily identify the JobOutput. If a label is not provided, a default value of '{presetName}_{outputIndex}' will be used, where the preset name is the name of the preset in the corresponding TransformOutput and the output index is the relative index of the this JobOutput within the Job. Note that this index is the same as the relative index of the corresponding TransformOutput within its Transform.
*/
@JvmName("kkhtimqorfdhpgpu")
public suspend fun label(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.label = mapped
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.Media.JobOutputAsset'.
*/
@JvmName("djvxltvmthsdchoh")
public suspend fun odataType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.odataType = mapped
}
/**
* @param value A preset used to override the preset in the corresponding transform output.
*/
@JvmName("wqietcyqfuchfdny")
public suspend fun presetOverride(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.presetOverride = mapped
}
internal fun build(): JobOutputAssetArgs = JobOutputAssetArgs(
assetName = assetName ?: throw PulumiNullFieldException("assetName"),
label = label,
odataType = odataType ?: throw PulumiNullFieldException("odataType"),
presetOverride = presetOverride,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy