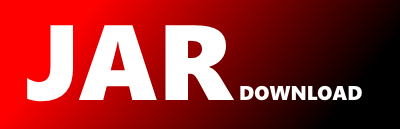
com.pulumi.azurenative.media.kotlin.inputs.LiveEventPreviewArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin.inputs
import com.pulumi.azurenative.media.inputs.LiveEventPreviewArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Live event preview settings.
* @property accessControl The access control for live event preview.
* @property alternativeMediaId An alternative media identifier associated with the streaming locator created for the preview. This value is specified at creation time and cannot be updated. The identifier can be used in the CustomLicenseAcquisitionUrlTemplate or the CustomKeyAcquisitionUrlTemplate of the StreamingPolicy specified in the StreamingPolicyName field.
* @property endpoints The endpoints for preview. Do not share the preview URL with the live event audience.
* @property previewLocator The identifier of the preview locator in Guid format. Specifying this at creation time allows the caller to know the preview locator url before the event is created. If omitted, the service will generate a random identifier. This value cannot be updated once the live event is created.
* @property streamingPolicyName The name of streaming policy used for the live event preview. This value is specified at creation time and cannot be updated.
*/
public data class LiveEventPreviewArgs(
public val accessControl: Output? = null,
public val alternativeMediaId: Output? = null,
public val endpoints: Output>? = null,
public val previewLocator: Output? = null,
public val streamingPolicyName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.inputs.LiveEventPreviewArgs =
com.pulumi.azurenative.media.inputs.LiveEventPreviewArgs.builder()
.accessControl(accessControl?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.alternativeMediaId(alternativeMediaId?.applyValue({ args0 -> args0 }))
.endpoints(
endpoints?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.previewLocator(previewLocator?.applyValue({ args0 -> args0 }))
.streamingPolicyName(streamingPolicyName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LiveEventPreviewArgs].
*/
@PulumiTagMarker
public class LiveEventPreviewArgsBuilder internal constructor() {
private var accessControl: Output? = null
private var alternativeMediaId: Output? = null
private var endpoints: Output>? = null
private var previewLocator: Output? = null
private var streamingPolicyName: Output? = null
/**
* @param value The access control for live event preview.
*/
@JvmName("brlibrqtvcskqspp")
public suspend fun accessControl(`value`: Output) {
this.accessControl = value
}
/**
* @param value An alternative media identifier associated with the streaming locator created for the preview. This value is specified at creation time and cannot be updated. The identifier can be used in the CustomLicenseAcquisitionUrlTemplate or the CustomKeyAcquisitionUrlTemplate of the StreamingPolicy specified in the StreamingPolicyName field.
*/
@JvmName("vgfelwonwanmjmni")
public suspend fun alternativeMediaId(`value`: Output) {
this.alternativeMediaId = value
}
/**
* @param value The endpoints for preview. Do not share the preview URL with the live event audience.
*/
@JvmName("yqpcvmbkrfgcuxge")
public suspend fun endpoints(`value`: Output>) {
this.endpoints = value
}
@JvmName("blgcvmcsbxrskcow")
public suspend fun endpoints(vararg values: Output) {
this.endpoints = Output.all(values.asList())
}
/**
* @param values The endpoints for preview. Do not share the preview URL with the live event audience.
*/
@JvmName("oadxhligtynbddbm")
public suspend fun endpoints(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy