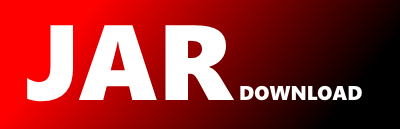
com.pulumi.azurenative.media.kotlin.inputs.MediaGraphRtspSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.media.kotlin.inputs
import com.pulumi.azurenative.media.inputs.MediaGraphRtspSourceArgs.builder
import com.pulumi.azurenative.media.kotlin.enums.MediaGraphRtspTransport
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* RTSP source.
* @property endpoint RTSP endpoint of the stream being connected to.
* @property name Source name.
* @property odataType The discriminator for derived types.
* Expected value is '#Microsoft.Media.MediaGraphRtspSource'.
* @property transport Underlying RTSP transport. This can be used to enable or disable HTTP tunneling.
*/
public data class MediaGraphRtspSourceArgs(
public val endpoint: Output>,
public val name: Output,
public val odataType: Output,
public val transport: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.media.inputs.MediaGraphRtspSourceArgs =
com.pulumi.azurenative.media.inputs.MediaGraphRtspSourceArgs.builder()
.endpoint(
endpoint.applyValue({ args0 ->
args0.transform({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.name(name.applyValue({ args0 -> args0 }))
.odataType(odataType.applyValue({ args0 -> args0 }))
.transport(
transport.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [MediaGraphRtspSourceArgs].
*/
@PulumiTagMarker
public class MediaGraphRtspSourceArgsBuilder internal constructor() {
private var endpoint: Output>? =
null
private var name: Output? = null
private var odataType: Output? = null
private var transport: Output>? = null
/**
* @param value RTSP endpoint of the stream being connected to.
*/
@JvmName("ffknlijseafkfhsd")
public suspend fun endpoint(`value`: Output>) {
this.endpoint = value
}
/**
* @param value Source name.
*/
@JvmName("fyhlgphosdbkjevq")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.Media.MediaGraphRtspSource'.
*/
@JvmName("rxcidaxnmnqesfvx")
public suspend fun odataType(`value`: Output) {
this.odataType = value
}
/**
* @param value Underlying RTSP transport. This can be used to enable or disable HTTP tunneling.
*/
@JvmName("yjevbhvgvkkobneq")
public suspend fun transport(`value`: Output>) {
this.transport = value
}
/**
* @param value RTSP endpoint of the stream being connected to.
*/
@JvmName("bvcuxysqwllsbmux")
public suspend fun endpoint(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value RTSP endpoint of the stream being connected to.
*/
@JvmName("rambejbrjyrhtpba")
public fun endpoint(`value`: MediaGraphClearEndpointArgs) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value RTSP endpoint of the stream being connected to.
*/
@JvmName("mrempxohtdnbipvm")
public fun endpoint(`value`: MediaGraphTlsEndpointArgs) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value Source name.
*/
@JvmName("nnibnjmlshmbrdes")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.Media.MediaGraphRtspSource'.
*/
@JvmName("baiwspbcwifpmswu")
public suspend fun odataType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.odataType = mapped
}
/**
* @param value Underlying RTSP transport. This can be used to enable or disable HTTP tunneling.
*/
@JvmName("xfxdunapuwiprbaw")
public suspend fun transport(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.transport = mapped
}
/**
* @param value Underlying RTSP transport. This can be used to enable or disable HTTP tunneling.
*/
@JvmName("jfwtqohhstdtubkg")
public fun transport(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.transport = mapped
}
/**
* @param value Underlying RTSP transport. This can be used to enable or disable HTTP tunneling.
*/
@JvmName("iuijyrwdkirgrxwh")
public fun transport(`value`: MediaGraphRtspTransport) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.transport = mapped
}
internal fun build(): MediaGraphRtspSourceArgs = MediaGraphRtspSourceArgs(
endpoint = endpoint ?: throw PulumiNullFieldException("endpoint"),
name = name ?: throw PulumiNullFieldException("name"),
odataType = odataType ?: throw PulumiNullFieldException("odataType"),
transport = transport ?: throw PulumiNullFieldException("transport"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy